Class hours: 9:40 – 2:05
Mr. Bohmann
wbohmann@ewsd.org
Today’s Notes
- Today is an EHS B Day
- Ryan – SLC Meeting today at 11:45. Lunch Provided
- Carter – Bus at 1:30 today
- Isaac – Meeting Thursday at Noon
Date | Week | Deliverable | Software Development Cycle |
---|---|---|---|
May 16th – 20th | Two | Game Design Document, Asset creation / Coding | Analysis / Design |
May 23rd – May 27th | Three | GUI, Movement, Core Mechanics | Design / Implementation |
May 31st – Jun 3rd | Four | Prototype with game play | Testing/Maintenance / Publishing |
June 6th | June 6th Game Jam |
9:40 Attendance
9:45 Managing Multiple Scenes in Unity – Part II
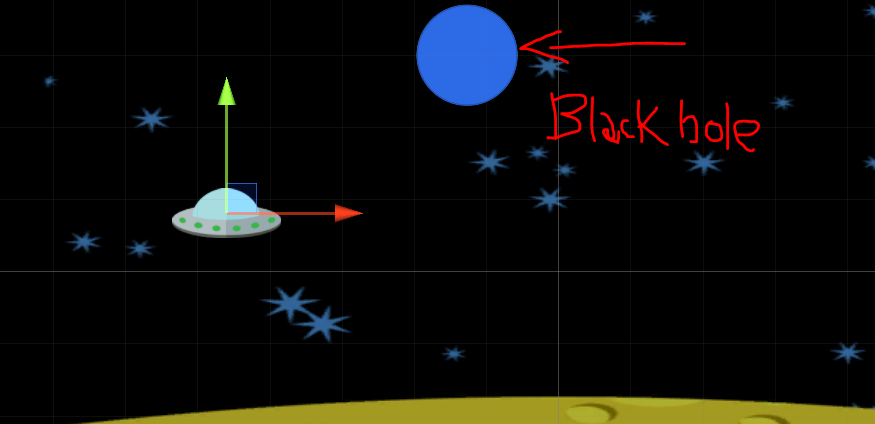
Yesterday we made multiple space scenes and then used a script to link our scenes together through a trigger event. Can you think of some scenes you might want to trigger?
To review
Unity scripts uses a built-in object called the SceneManager to load and unload scenes. Any script that wants to use the SceneManager should first place the following “using” statement at the top of the source file, near the other “using” statements. This adds the SceneManagement library
using UnityEngine.SceneManagement; //place at the top of your script
Now, when your script wants to change from one scene to another, it can simply call the SceneManager.LoadScene() function and pass in the name of the new scene inside your parenthesis.
When you call LoadScene() with just a scene name, as we have demonstrated, you are using single mode.
In additive mode, it is possible to load and display multiple scenes at once. Each scene may contribute something to the set of visible objects and overall gameplay. To use additive mode, simply add a LoadSceneMode.Additive parameter after the scene name in a call to LoadScene().
SceneManager.LoadScene (nextScene, LoadSceneMode.Additive);
You can have multiple scenes loaded in additive mode, but only one of those scenes is active at a time. Let’s experiment. However, you may notice some problems – like lots of spaceship and black holes and many cameras and background in the hierarchy.
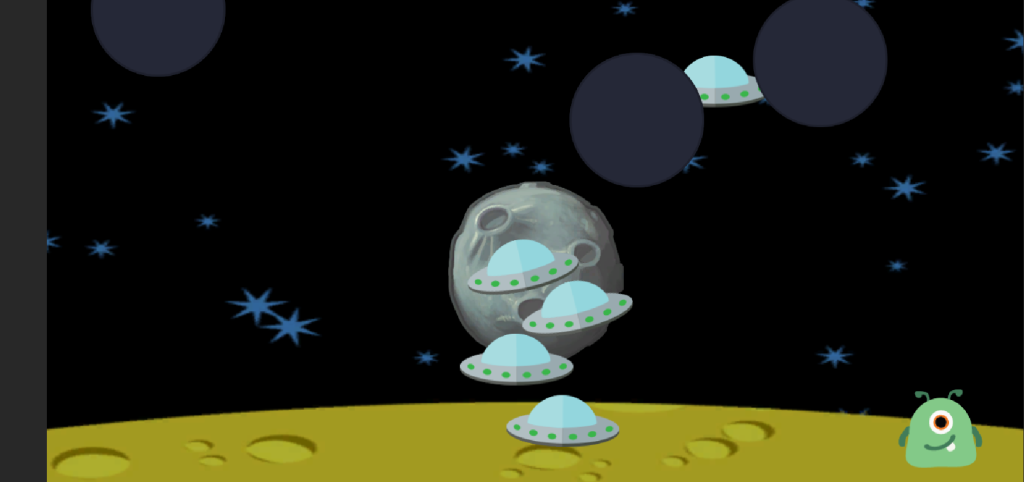
In Single scene mode each time a new scene loads, the previous scene and all of the objects are destroyed. But most games will need to track information like player health, score, inventory, etc…
There are several ways you can save data across multiple scenes
- Using the Unity GameObject.DontDestroyOnLoad() function
- Use the C# “static” keyword in from of class level variables that you want to keep
- Write data to a file or database
- Use the additive mode when loading new scenes. Design one scene as a “manager” that will always be present and hold all of the game’s long-term data and objects.
Loading Additive Scenes
One strategy is to keep one scene open – called the manager scene and then offload and onload new scenes through-out the game. This way, some gameObjects can remain part of every scene.
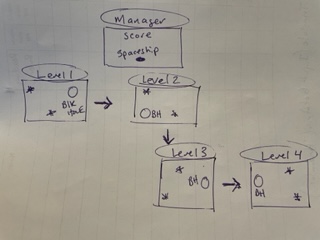
Let’s reconfigure our Scenes to take advantage of this approach.
- Create a New Level and Name it “Manager”
- add your spaceship PreFab
- On your other levels, remove the camera and the spaceship
- Check your Build Settings to make sure everything is looking good and your new Manager Scene has been added
Now, when the game starts with the manager scene, we need some logic on an object within the manager to automatically load the first level in additive mode. The player character from the manager scene will combine with the space scene for exactly the same look and feel.
//Controller Script for the Manager Scene
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Controller : MonoBehaviour
{
public string nextScene; // Variable so we can type in the first scene to load
void Start()
{
SceneManager.LoadScene(nextScene, LoadSceneMode.Additive);
}
}
Next, when the player character enters a black hole, the old level/scene will be unloaded and the new scene/level will be loaded in additive mode. This keeps the manager scene loaded throughout the game, and we will only have one copy of our player object that survives from level to level!
//Transition Script placed on the black hole for managing levels changes
public class BlackHole : MonoBehaviour
{
public string nextLevel;
public string currentLevel;
private void OnTriggerEnter2D(Collider2D collision)
{
SceneManager.LoadScene(nextLevel, LoadSceneMode.Additive);
SceneManager.UnloadSceneAsync(currentLevel);
}
}
Not only can we store long-term data on the player character or other objects within the manager, but we can use knowledge of which black hole was entered on the old scene to position the player character just outside the matching black hole in the new scene. This extra attention to detail makes the game flow more naturally. Let’s try…
10:35 Mask Break
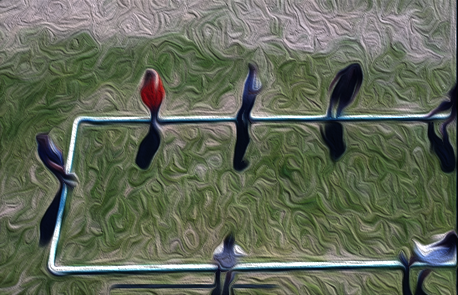
10:45 – 12:15 Game Studio Work time
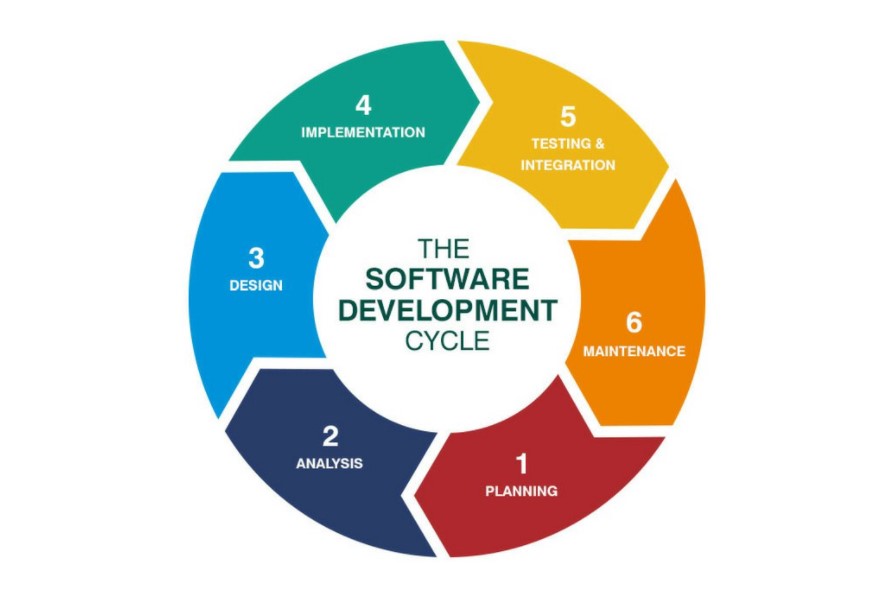
Start with a 5 minutes Scrum Meeting (group or individual)
- What are you working on?
- What will you complete today?
- What help do you need?
Update your Trello Boards (5 minutes) and / or add to the details of your Trello board.
Tackle your core game mechanic. So if running, jumping and movement is at the core of your game, you should be able to do that by the end of the week. If your core mechanic is shooting, then by Friday, your game now is shooting.
Question? How will you know when your game is done? Ask yourself and your group what the bare bones deliverables are. That way you know what you are working towards.
Don’t be surprised if I ask you this question.
12:15 Lunch
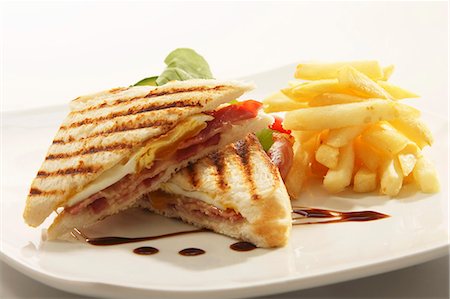
12:45 Focus on Literacy
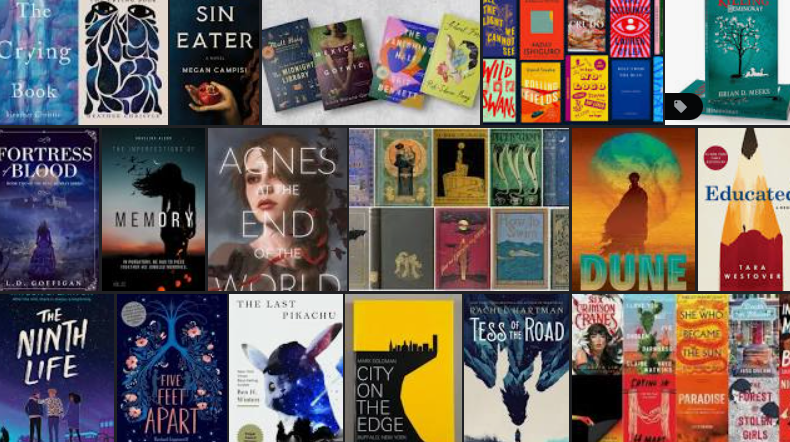
1:05 Mask Break
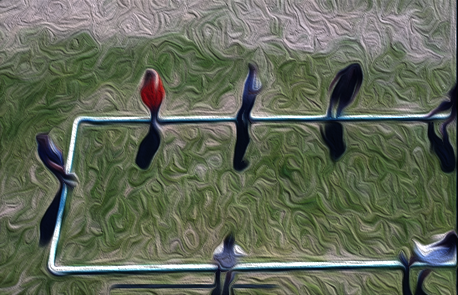
1:15 Production Time & Guided Support
CAWD Fun Games Studio work time (my fancy way of saying work with your game / game team
Unity Micro Lesson – Due Monday, May 23rd – Dropbox