Class hours: 9:40 – 2:05
Mr. Bohmann
wbohmann@ewsd.org
Today’s Notes
- Today is an EHS A Day
- Web Professionals Exam – Tuesday, June 7th
- Last week we built some Minimum Viable Products (Pinball and Breakout Games), this week we will add to those games by coding additional functionality while at the same time learning about variables and flow control.
- Games… Is there a game you want to make? Start thinking….
- Workkeys testing on Tuesday, May 10th and 12th.
Let’s break it down….No One is here on Tuesday, May 10th – you all scored a 5 or better- Some of you are here on Thursday, May 12th – see graphic below
- If you are not one of the students listed you are off on May 12th too.
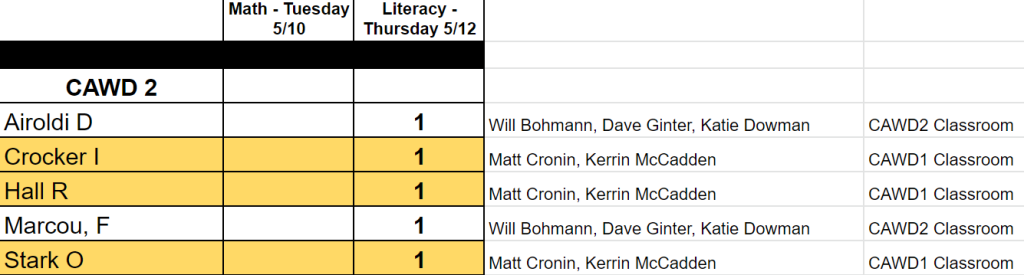
9:40 Attendance
9:45 Unity – Setting Up Scores
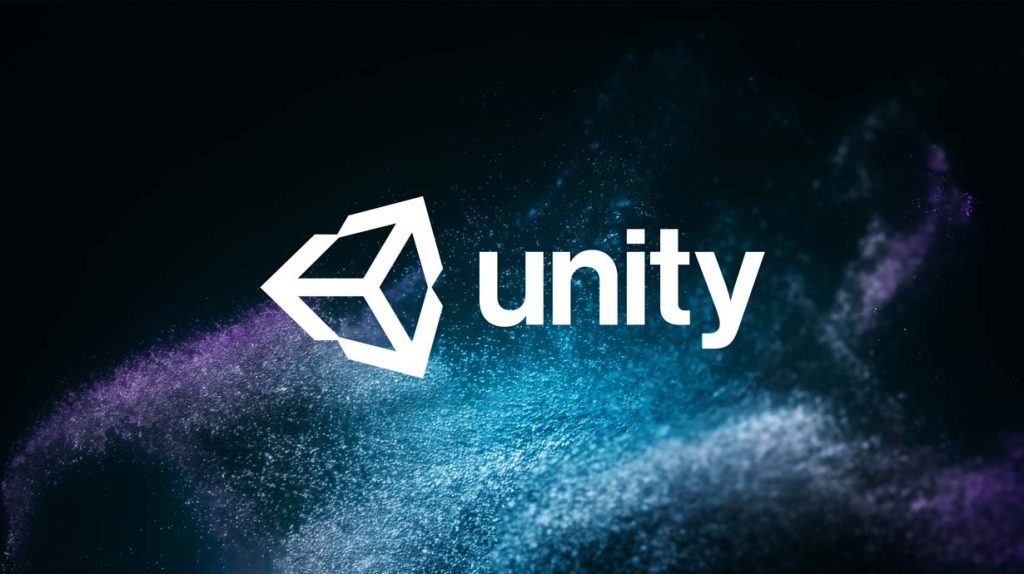
Pinball – Let’s level up using variables
You can use variables to do important things within your game. For example, many games will need to keep a score. Let’s add a score keeping variable to the pinball game and have the results printed in a Debug.Log statement. We use Debug.Log as a way to see if everything is working.
To begin, you will need to declare a variable for score (what type of variable will you use? What will you name your variable? and where should you put it?). We want the Score to be displayed when the game begins (so we’ll place a Debug.Log statement in the Start() method.
One last decision, – which game object should we create a script for?
void Start ()
{
// display the current score on start
Debug.Log ("Score = " + score);
}
//score is a good name for our variable - very semantic
Inside the OnCollisionEnter2D() function, set the score variable value to 1. Then, again use Debug.Log() to display the current score to the screen.
void OnCollisionEnter2D (Collision2D other)
{
// set the score to 1
score = 1;
// display the new score
Debug.Log ("Score = " + score);
}
In the example above, the score just stays at 1. Not entirely what we want. So, let’s look at using some operational shortcuts in our code to how we can calculate the score each time the ball collides with a gameObject that has a collider.
score = score +1; //adds 1 to the variable
score +=1; //means the same above. Add what's on the right to the left
score ++; //means add one to the score
Instead of using just the Debug.Log function, we could initialize our score variable at the top of our script as a public variable and then as the game plays, we can watch the score increase in the inspector.
Let’s Level Up some more: Setting up Score with the UI Canvas – Instructions
Project Details:
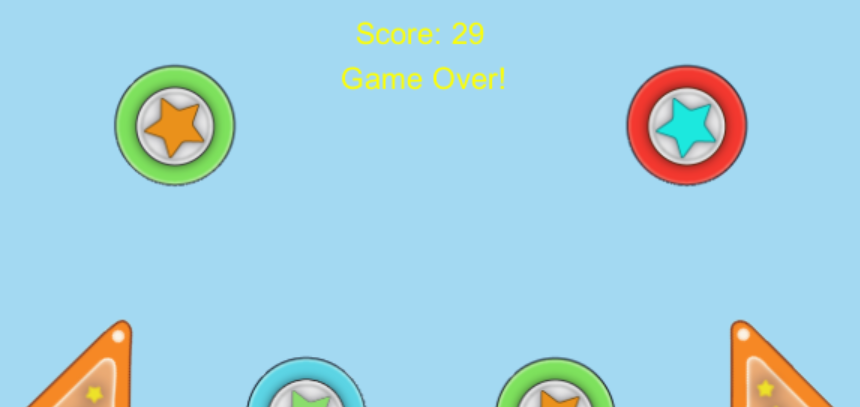
The player’s score should start at 0 and be displayed in the top center of the screen. Each time the ball collides with any other object (bumpers, walls, paddle), the score should increase by 1. When the game ends, the phrase “Game Over!” should be displayed underneath the score.
Here is the big picture which is an outline of the basic steps to add these features
- Add a Canvas and two Text objects (one for the score, one for the game-over message)
- Edit the existing BallScript and add a score variable and two public Text variables
- Modify the BallScript functions to update the score and Text variables as needed
- Link the two Text objects to the two Text variables in the BallScript
Easy right? Actually it is. Let’s work on each of the steps together.
If you get lost – here is a reference you can use.
10:45 English with Ms. Yopp
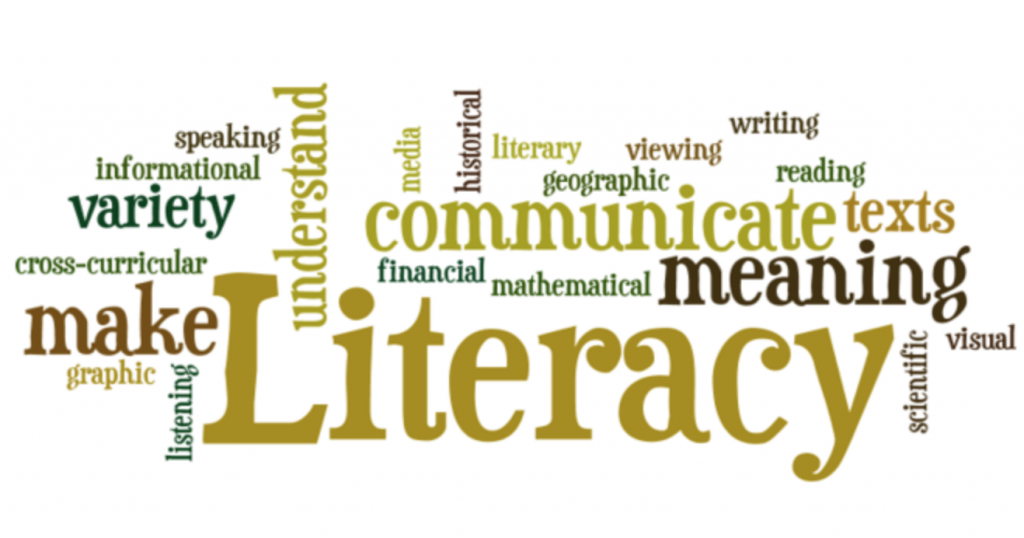
11:35 Unity – Logical Expressions
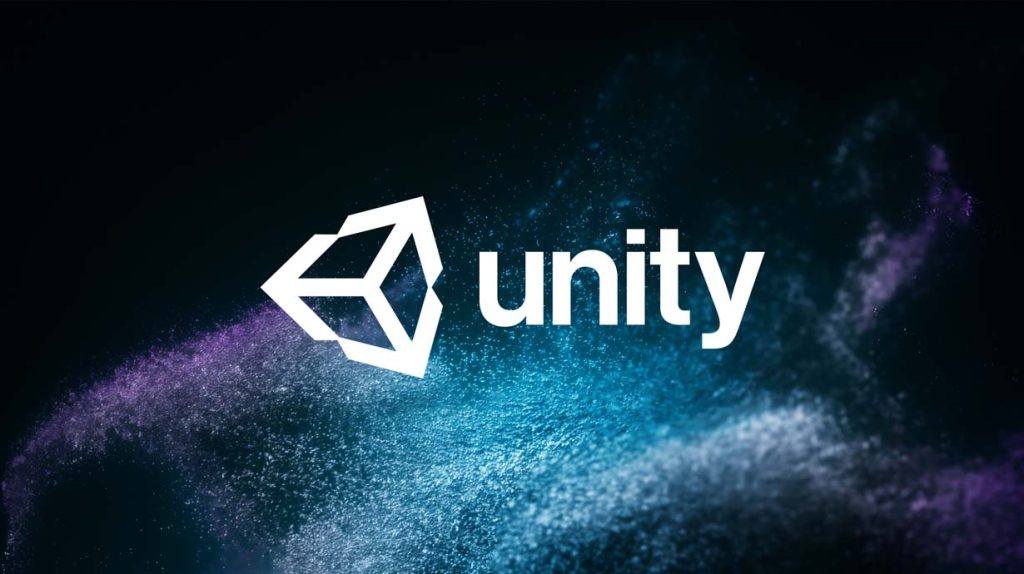
Normally, a pinball game like the one we’ve made will only add to the score when a bumper is hit. You may even want to assign different point values to different targets. To support this kind of advanced scoring, you would need to examine the actual objects involved in the collisions and make decisions about how many points to add to the score.
The general process of selecting statements to run is called flow control.
An expression is a part of a code statement that is evaluated to produce some answer. You are already familiar with mathematical expressions like 4 + 4 that will produce an answer such as 8.
int speed = 4 + 4; //score is a mathematical expression
To make decisions in your code, you need a different kind of expression – a logical expression. That means questions you ask in your code have two possible answers – true (yes) or false (no).
So, you remember from our Endless Runner that a Boolean (bool) data type is used to store a true or false value.
bool alive = health > 0; // health > 0 is a logical expression
There is no mathematical operation, but instead, this is a comparison that looks at the value on both sides of the “>” symbol in the middle. The answer is either true or false.
There are several comparison operators:
- == (equal to operator) the result is true if both sides are equal
- != (not equal operator) the result returns true is two sides are not equal to each other
- < (less than operator)
- >(greater than operator)
- <=(less than or equal to operator)
- >=(greater than or equal to operator)
There are several logical operators:
Logical Operator | C# Symbol | Example | Description |
---|---|---|---|
and | && | A &&B | Returns true if both “A” and “B” is true, or false otherwise |
or | || | A || B | Returns true if either “A” or “B” are true, or false otherwise |
exclusive or | ^ | A ^ B | Returns true if either “A” is true and “B” is false, or vice-versa |
not | ! | !A | Returns true if “A” is false or false if “A” is true |
Let’s Practice. If you had two variables named score and health, how would you write a logical expression that was true if both score was greater than 100 and health was equal to 0?
Let’s Practice more….
void Start ()
{
int value1 = 50;
int value2 = 100;
int value3 = 100;
int value4 = 200;
bool result1 = value1 == value2;
bool result2 = value2 == value3;
bool result3 = value4 > value3;
bool result4 = value1 <= value2;
bool result5 = value2 != value3;
bool result6 = result1 && result2;
bool result7 = result1 || (value4 < 300);
bool result8 = !result1;
bool result9 = !(value3 == 100);
bool result10 = value1 + value2 >= 150;
bool result11 = value2 - value1 * 2 == 100;
Debug.Log ("result1 = " + result1);
Debug.Log ("result2 = " + result2);
Debug.Log ("result3 = " + result3);
Debug.Log ("result4 = " + result4);
Debug.Log ("result5 = " + result5);
Debug.Log ("result6 = " + result6);
Debug.Log ("result7 = " + result7);
Debug.Log ("result8 = " + result8);
Debug.Log ("result9 = " + result9);
Debug.Log ("result10 = " + result10);
Debug.Log ("result11 = " + result11);
//Write on a piece of paper 1-11 and declare if the expressions //will return true or false
}
Let’s check our answers….
If/Else Statements
The if() Statement will execute a block of code if a logical expression evaluates to true.
The “if” keyword is always followed by a logical expression in parentheses. The logical expression can be very simple or complicated, but it must evaluate to either true or false. If the logical expression is true, the code contained within the curly braces below the “if” statement is executed. This block of code is often called the “body” of the if() statement. If the expression is false, the code in the curly braces is skipped, and the program continues on the next line below the ending curly brace.
Let’s practice using If, Else If and Else to see how the logical expression is handled in C#.
void Update()
{
if(score > 1000)
{
Debug.Log("Great Job!");
}
else if(score >500)
{
Debug.Log("Pretty good");
}
else
{
Debug.Log("Try harder...");
}
}
Socrative.com for a little review from today…
12:15 Lunch
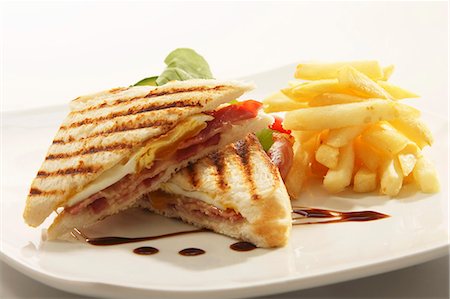
12:45 Focus on Literacy
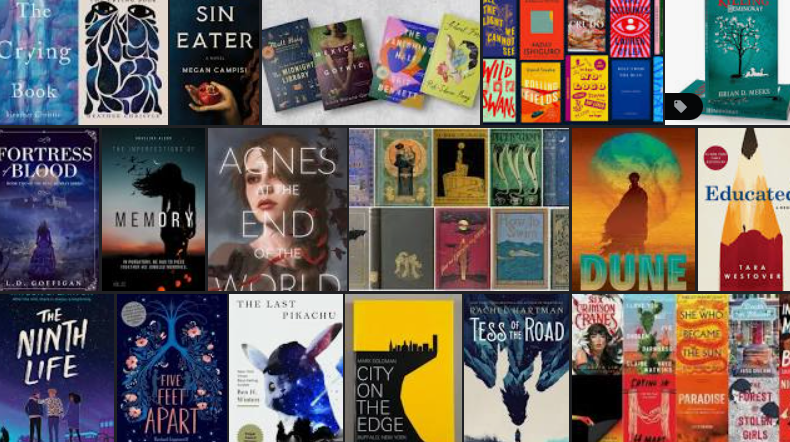
1:05 Mask Break
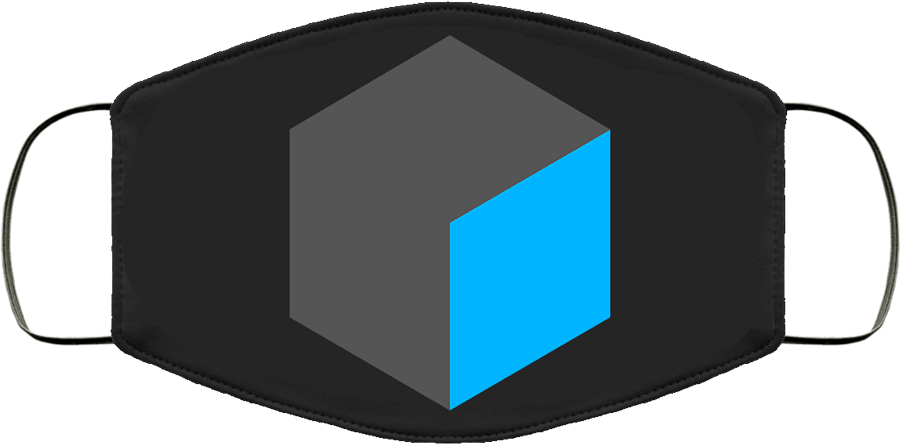
1:15 Production Time & Guided Support
Week 33 Agency is now Assigned – Due May 9th
Past due work – see me if you are not sure