Class hours: 9:40 – 2:05
Mr. Bohmann
wbohmann@ewsd.org
Today’s Notes
- Today is an EHS B Day and a White Day
- Ryan, SLC Meeting at 11:45am
- Carter, bus at 1:30 – see ya
- other news…..
9:40 Attendance and Review
Socrative.com for a little review from yesterday
9:50 Flow Control Continued – New Game
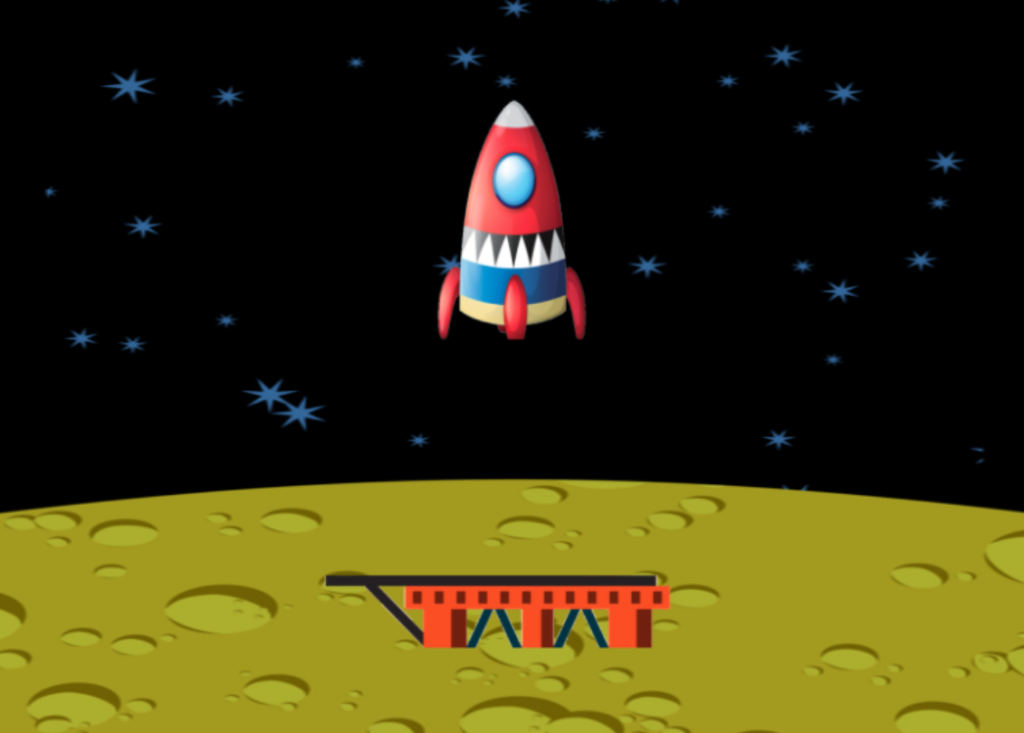
Let’s re-create a classic arcade game (LunarLander) and use your new decision-making skills to script the game logic.
The object of the game is to keep the rocket from crashing into the landing platform. This game will be a single button game – we’ll use the up arrow key – that will provide thrust to the rocket.
Each time the rocket collides with the platform, we’ll program a message response that evaluates the condition of the landing/how hard the rocket touches down, like Crash or Smooth.
We are creating the core mechanics of the game. How would you scale this game?
Go to the FunSprites folder and copy down the three sprites in the picture above.
Activity Details:
- Create a new scene – Lunar Lander
- Organize your gameObjects with layers (background / foreground
- Add Colliders to the Lander and Platform
- Add Physics to your Lunar Lander
- Add a canvas
- Add a UI Text called MessageText and place at the top of the screen
- Add a LunerLander script to your rocket
- Add the Adding UnityEngline.UI library to your script
- Create a public Text variable called messageText
- Create an OnCollisionEnter2d function
Each GameObject has one or more components such as a Transform, Rigidbody2D, Collider2D, and so on. You will find that scripting logic will often need to access these components to handle game features. The Transform object is always present, and you know that you can get to Transform properties and functions simply by using the “transform.” variable as shown below.
transform.Translate(0, Time.deltaTime, 0);
However, it’s not as easy to reach other components that are optional. Not all sprites have a Rigidbody2D or Collider2D object attached. To reach these optional components, you need to call the GetComponent() function like this:
Rigidbody2D rb = GetComponent<Rigidbody2D> ();
For our LunarLander game, we want to apply an upward thrust to the sprite when an up arrow key is pressed. For realistic movement, we don’t simply want to call transform.Translate() to move the ship upwards. Instead, we want a force to gradually counteract gravity and, if held down long enough, begin moving the ship up the screen. These realistic simulations are handled for us by the Unity physics engine. So, we simply need to get the Rigidbody2D component and call AddForce() to apply a directional force on the sprite.
rb.AddForce (new Vector2 (0.0F, Time.deltaTime * 650.0F));
The AddForce() function takes a single parameter – a Vector2 object that contains the forces to be applied in the X and Y directions. We simply want an upwards trust, so used 0 for the X and we use a value of 650 multiplied by Time.deltaTime for the Y values. Of course, we are multiplying by Time.deltaTime in order to make the force the same no matter how fast your computer calls the Update() method.
Let’s look at the OnCollisionEnter2D() Function
This function will be run when the lander touches the platform. The platform has a BoxCollider2D. The first statement declares a local variable called relativeVelocity. This variable gets a number from the Collision2D object. The Collision2D object contains a property called relativeVelocity that gives us the relative X and Y speeds between the two objects involved in the collision.
void OnCollisionEnter2D(Collision2D otherObject)
{
// get the speed at which the lander
// has hit the platform
float relativeVelocity = otherObject.relativeVelocity.magnitude;
Debug.Log ("relativeVelocity = " + relativeVelocity);
// ??? add an if/else chain here that will display
// CRASH if relativeVelocity is greater than 5
// or SAFE otherwise ???
}
Final Code for your Reference
10:35 Mask Break
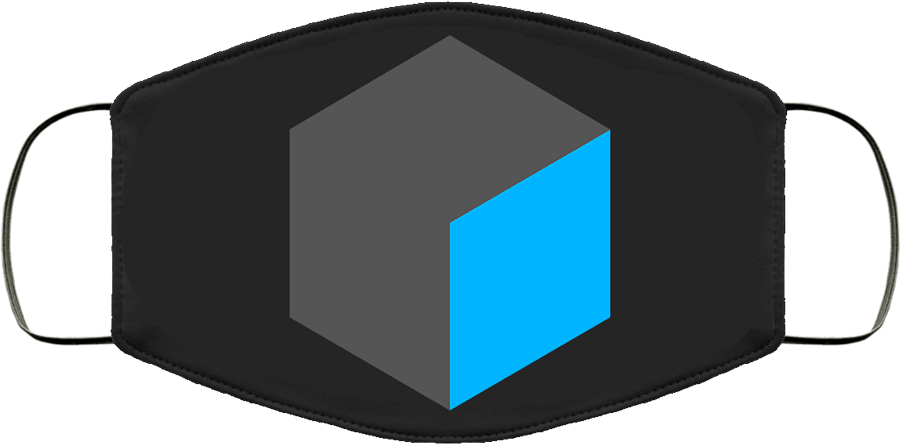
10:45 Lunar Lander Continued
11:00 Core Game Loops and Game Mechanics
Most well thought out games have a core game loop. Every action and feature set is fed back into this core loop. For example, in Sea of Thieves you explore a open world, plunder ships and amass your fortune.
Every game has a core game mechanic. In a platform game this is usually jumping (SuperMario) or in CSGo it is shooting. The core mechanic is an purposeful interaction that happens most frequently.
One of the best ways to figure out what a game is all about is to sit down and play some. Let’s do just that. Spelunky Slither and then take a stab and identifying what the game is all about.
11:30 One Button Game Assignment
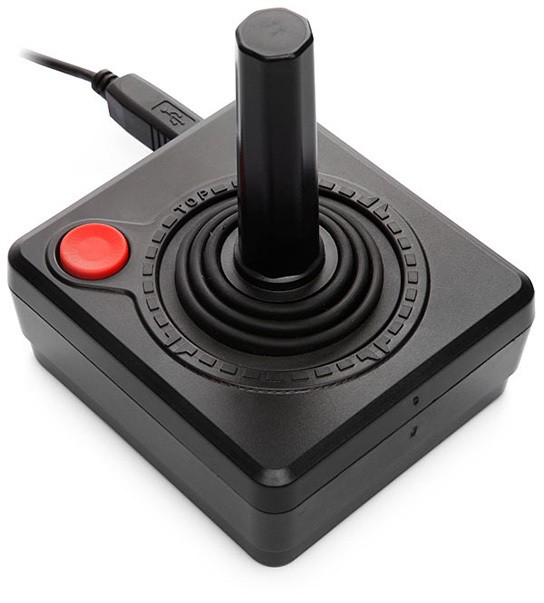
You work for a hard-core gaming company called CAWD Top Games, Inc. The sole purpose of the company is to make money by creating simple and addicting games. The Management wants ideas for a game that can only have one button as the main interaction.
Wild Metal Country is a vintage Rockstar game released in 1999 – Yes, before you were born. This is a classic one button game. In Wild Metal Country the firing of a projectile happens on the release of the button. This has an important effect on the player. They will know that once they have pressed down the button they are committed to firing at some point in the future (or jumping their tank in this game). With the added element of trajectory involved, there are the mechanics for a simple skill based game.
If you have a PC you can download
- Your Task: Write a game proposal for your best one button game. Include:
- Game Title
- Game Genre
- Overview: the basics of the game
- Rules
- Setting
- Challenges
- Core Game Loop
- Game Mechanics
- Victory Conditions
Create your One Button Game Proposal on a Google Doc. You can organize your document as you see fit. Spelling, grammar and punctuation count! Have someone proofread your proposal. Make sure you have a complete and well thought out proposal.
Title it: CAWD Top Games – One Button and Submit in Google Classroom. – Due Monday, May 9th.
12:15 Lunch
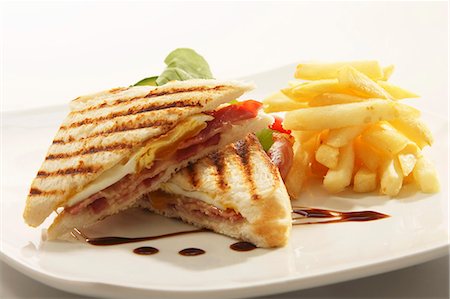
12:45 Focus on Literacy
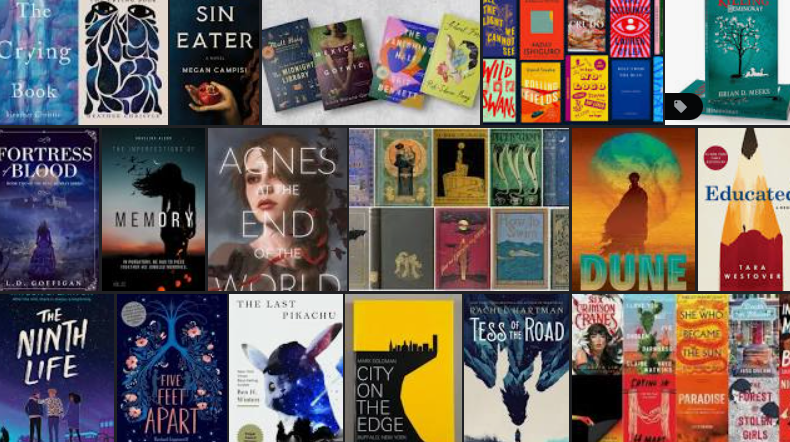
1:05 Mask Break
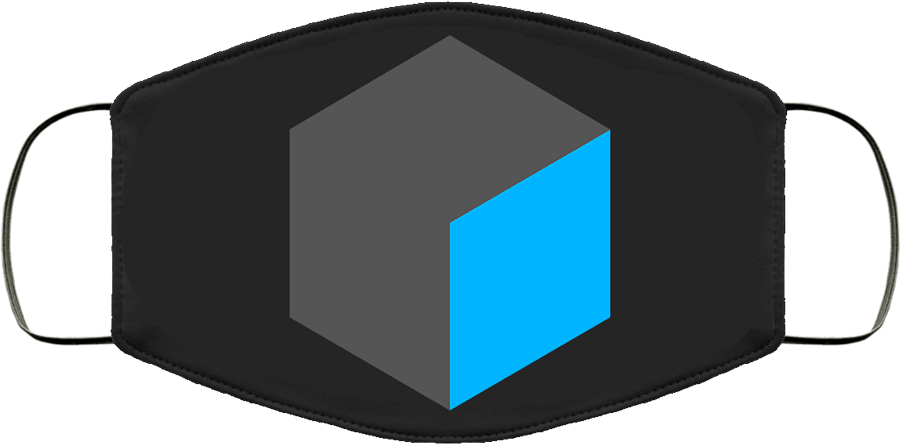
1:15 Production Time & Guided Support
Week 33 Agency – Due Monday, May 9th
Lunar Lander – for grade – due tomorrow
Game Core Loops Google Form
One Button Game Proposal – Due May 9th