Class hours: 9:40 – 2:05
Mr. Bohmann
wbohmann@ewsd.org
Week Thirty!
Today’s Notes
- Today is an EHS A Day
- Next week is last week before break
- Saturday, April 22nd – Sunday April 30th is break
- Ice Cream Party next Friday at 11:40 – then chill time afterwards
- Mr. B – I’ll be away for a funeral Wed – Fri. Mr. Bisson will be here
- 20% Updates – will be on Monday – Reverse Alpha Order
9:45 Lip Sync Battle
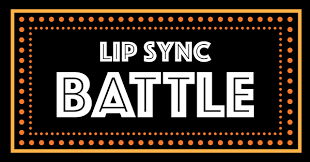
Using a random generator (with supervision from Eric) we’ll set up the pairings for our 2023 LipSync Battle. We’ll declare our preference on Socrative for:
- Quality of Lip Sync
- Staging and Appeal
Winner of each round will move on to the next round.
Let the battle begin!
10:35 Break
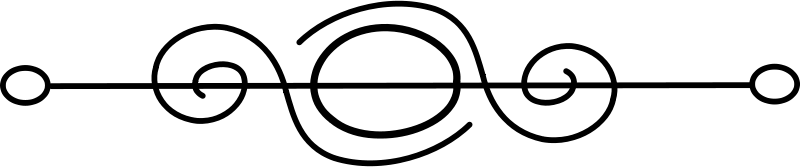
10:45 Unity Stuff – Let’s make a One Button Game together
and do some Flow Control….
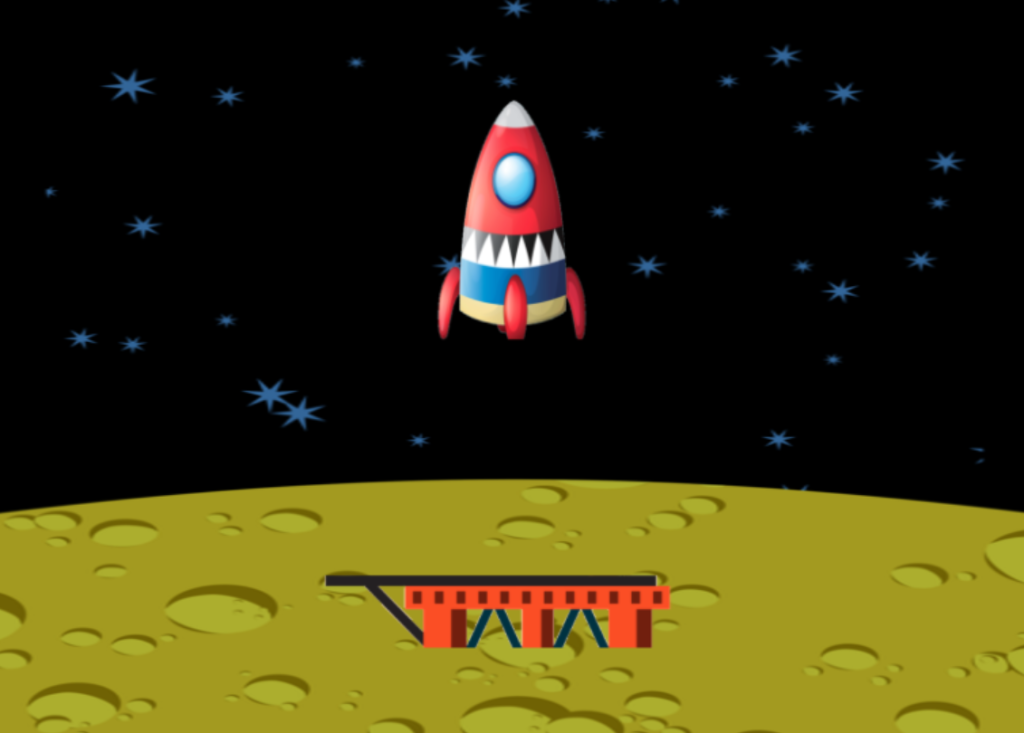
Let’s re-create a classic arcade game (LunarLander) and use our decision-making skills to script the game logic.
The object of the game is to keep the rocket from crashing into the landing platform. This game will be a single button game – we’ll use the up arrow key – that will provide thrust to the rocket.
Each time the rocket collides with the platform, we’ll program a message response that evaluates the condition of the landing/how hard the rocket touches down, like Crash or Smooth.
We are creating the core mechanics of the game. How would you scale this game?
Go to the FunSprites folder and copy down the three sprites in the picture above.
We’ll build this game in the 2D Programming Folder – name the Game Scene – Lunar Lander
Activity Details:
- Create a new scene – Lunar Lander
- Organize your gameObjects with layers (background / foreground
- Add Colliders to the Lander and Platform
- Add Physics to your Lunar Lander
- Add a canvas
- Add a UI Text called MessageText and place at the top of the screen
- Add a LunerLander script to your rocket
- Add the Adding Unity TMPro library to your script
- Create a public Text variable called messageText
- Create an OnCollisionEnter2d function
Each GameObject has one or more components such as a Transform, Rigidbody2D, Collider2D, and so on. You will find that scripting logic will often need to access these components to handle game features. The Transform object is always present, and you know that you can get to Transform properties and functions simply by using the “transform.” variable as shown below.
transform.Translate(0, Time.deltaTime, 0);
However, it’s not as easy to reach other components that are optional. Not all sprites have a Rigidbody2D or Collider2D object attached. To reach these optional components, you need to call the GetComponent() function like this:
Rigidbody2D rb = GetComponent<Rigidbody2D> ();
//What we are doing is creating a variable called "rb" and it is a RigidBody2D variable
For our LunarLander game, we want to apply an upward thrust to the sprite when an up arrow key is pressed. For realistic movement, we don’t simply want to call transform.Translate() to move the ship upwards. Instead, we want a force to gradually counteract gravity and, if held down long enough, begin moving the ship up the screen. These realistic simulations are handled for us by the Unity physics engine. So, we simply need to get the Rigidbody2D component and call AddForce() to apply a directional force on the sprite. Unity’s Documentation is really helpful. AddForce is a method build into Unity.
rb.AddForce (new Vector2 (0.0F, Time.deltaTime * 650.0F));
The AddForce() function takes a single parameter – a Vector2 object that contains the forces to be applied in the X and Y directions. We simply want an upwards thrust, so we use 0 for the X and we use a value of 650 multiplied by Time.deltaTime for the Y values. Of course, we are multiplying by Time.deltaTime in order to make the force the same no matter how fast your computer calls the Update() method.
Let’s look at the OnCollisionEnter2D() Function
This function will be run when the lander touches the platform. The platform has a BoxCollider2D. The first statement declares a local variable called relativeVelocity. This variable gets a number from the Collision2D object. The Collision2D object contains a property called relativeVelocity that gives us the relative X and Y speeds between the two objects involved in the collision.
void OnCollisionEnter2D(Collision2D platform)
{
// get the speed at which the lander
// has hit the platform
float relativeVelocity = platform.relativeVelocity.magnitude;
Debug.Log ("relativeVelocity = " + relativeVelocity);
// ??? add an if/else chain here that will display
// CRASH if relativeVelocity is greater than 5
// or SAFE otherwise ???
}
Final Code for your Reference
How about Particles – we can do that – how about adding Custom Fonts – yeah, we can do that too
- Custom Fonts with Text Mesh Pro:
Windows/Font Asset Creator/Upload a TruType Font/ Generate Font Atlas - You can get fonts from DaFont
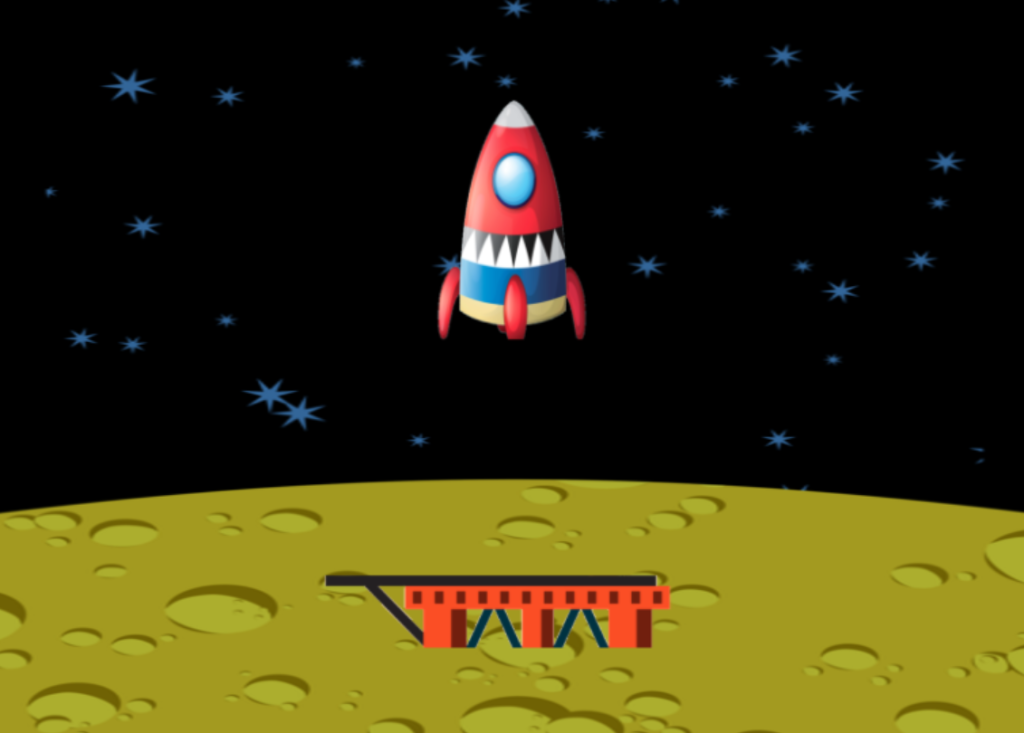
Quick Challenge – You have all of the necessary tools (examples) to complete the following challenge.
- Add a Lander Health Field or a Damage field on your UI canvas
- Create some variables at the class level:
- Create a Text type variable (we’ve done two of these already) to capture the damage or lander health
- Create an Int type variable for damage and initialize it to -1
- Add a nested If() statement inside of your Crash logic expression
- show damage text on your UI
- Show the lander health or damage score, which adds -1 each time you crash
- Add a custom font (go to DaFont) and add your TrueType Font to a Fonts folder in your Project
Bonus – Add a Game Over Message when your damage reaches -3
Super Bonus – reduce your damage by landing correctly.
12:15 Lunch
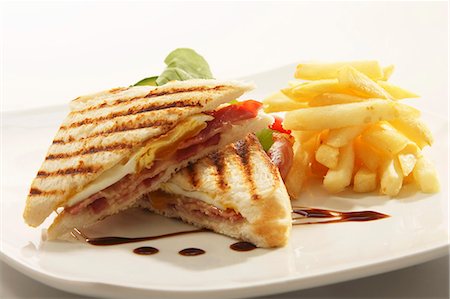
12:45 Independent Reading
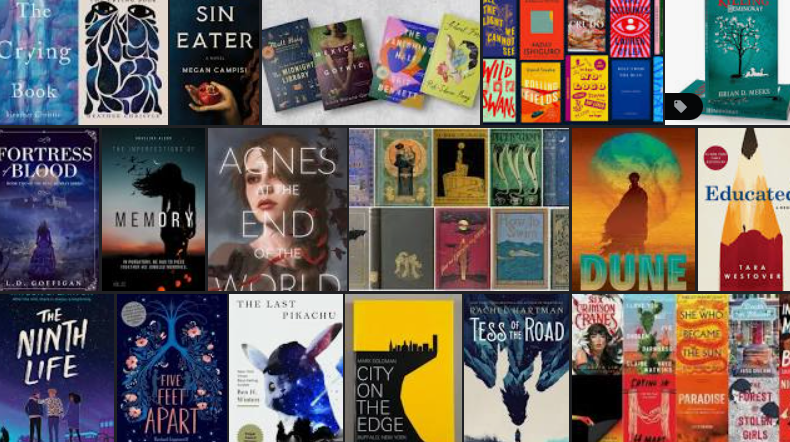
1:10 Break
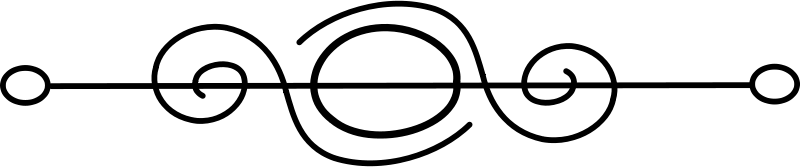
1:20 Independent Project Worktime of Individual Support
- WorkSession for Lip Sync Project
- 20% for Monday (Sprites, Code,
- Help with morning Coding