Class hours: 9:40 – 2:05
Mr. Bohmann
wbohmann@ewsd.org
Week Thirty Two
Today’s Notes
- Today is an EHS A Day
- Hireability – Friday
- Eric 10
- Tom 10:30
- Wall Sit Challenge
- Hayden, Finn, Emma, Garrett – at break – see Ms. Sorrell in CTE Office, bring your license
9:45 Making Toast
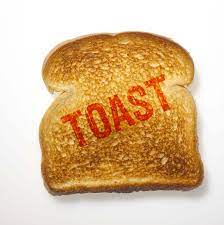
David Kelley – IDEO
Design Thinking: A repetitive process of prototyping, testing and refining a product or process based on the user’s needs. Empathy for the user is important!
Design Thinking Reflection
Making Toast (systems, links and nodes)
10:35 Break
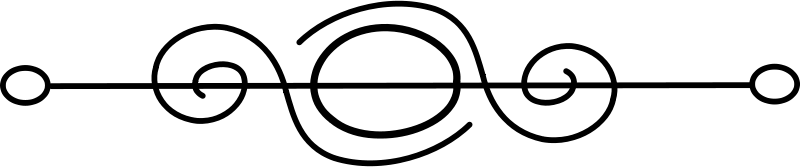
10:45 Improving Your Game – working in 3D
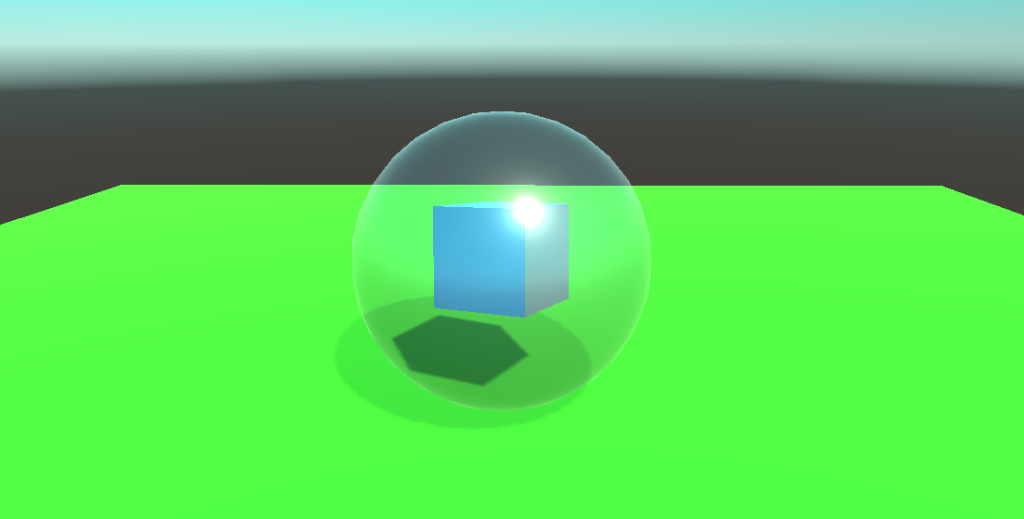
Remember our CAWD RollerBall Game? The core game mechanic is to roll the ball and navigate it around the different platforms. We can create custom platforms (and lots of other assets) in Unity and even in Blender.
Today, let’s add some collectibles, a scoring system and a UI to create more of a game.
Let’s start by looking at making a collectable prefab. We’ll need:
- sphere or box collider with trigger
- tag labeled “coin” on this prefab
- simple rotation script on the coin collectable (probably there already)
Instead of destroying each coin we collect, we’ll try a new method called SetActive. We’ll set our collectable item so that when they are collected the active state will return to false. We can put this block of code right on the player script.
void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("coin"))
{
other.gameObject.SetActive(false);
}
Test. If all is working well, your ball will collide and turn change the active state.
Next, Let’s add a scoring system so that when we collect the coins on the different platforms we can create a game with more purpose and a core game loop.
- create a private variable called score
- initialize the variable to 0 – this can be done in the Start() method
private void Start()
{
score = 0;
}
then update the OnTriggerEnter method which now will read:
void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("coin"))
{
other.gameObject.SetActive(false);
score++; //this adds one to the score for each key you collect
}
The next step is to add:
- UI Canvas
- Two Text Fields
- Score Text
- Win Text
- Update our script
- public text variable for scoreText
- public text variable for winText (to display win message)
- don’t forget we need to add the using TMPro library to the top of our script!
- Populate our game script with those fields
public TMP_Text scoreText;
public TMP_Text winText;
Now, if you want the score field to show up at the start of the game, we’ll write a new method so we don’t have to write the same line of code in two places (bad form)
void SetScoreText()
{
scoreText.text = "Score: " + score;
}
We can then call this method in the Start() method. You’ll also notice we’ll create an empty string for the Win message.
private void Start()
{
score = 0;
SetScoreText();
winText.text = ""; //called an empty string
}
Finally, your updated OnTriggerEnter method
void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("coin"))
{
other.gameObject.SetActive(false);
score++;
SetScoreText(); //this will display the updated score
if (score >= 2) // set the number to how many to win
{
winText.text = "You Win!";
}
}
Dealer’s Choice Options:
- Set up a lose condition with “You Lose” statement on your UI
- Customize your fonts
- Add particle effect when you collect a coin
- Add more platforms and more challenges
- Create assets, obstacles, walls
- Create an environment
- Add sound when you collect a coin
Basically, customize your game. We’ll work right up to lunch and take a walk around to see what you’ve built for your RollerBall game.
12:15 Lunch
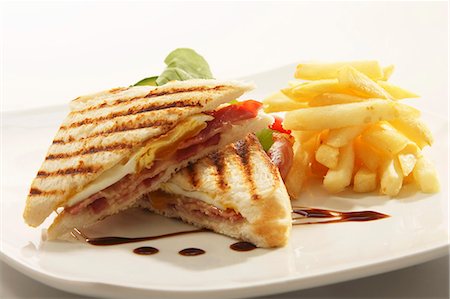
12:45 Independent Reading
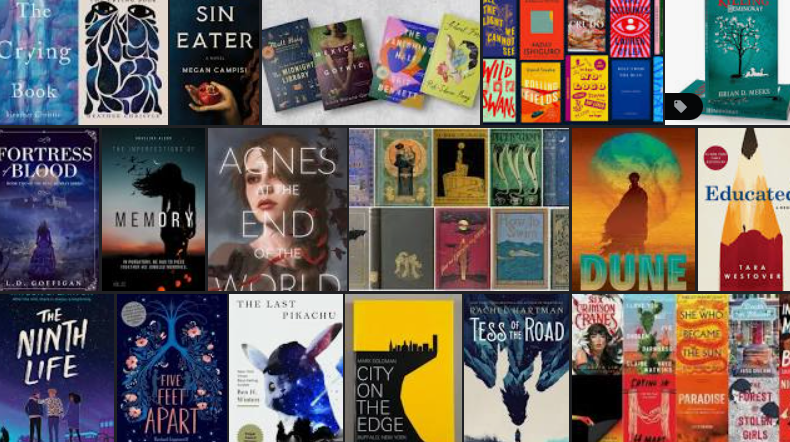
1:10 Break
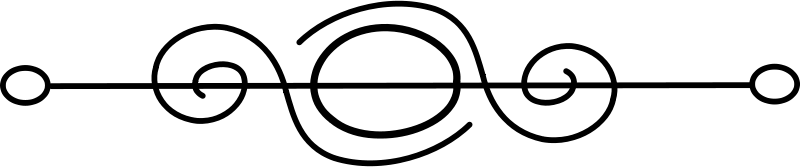
1:20 Independent Project Work Time of Individual Support
- Overdue work – Breakout / One Button / TileMaps level / Sprite Sheet / Exploring Game Careers
- CawdRoller Ball
- 20% for this week