Class hours: 9:40 – 2:05
Mr. Bohmann
wbohmann@ewsd.org
Week Thirteen
Today’s Notes
- Today is an EHS B Day
- Visitors – today at 10:45 – 11:30. We have five visitors. 8 minute rotations
- Schuyler – Creating in VR – show the Photography Gallery
- Eli – Playtesting Unity Game
- Hayden – Working Unreal – show off your island and how Unreal works
- Asa – Photo Editing and Camera Raw or discuss Skills USA, show game / talk about trip to Atlanta
9:40 Attendance
9:45 JavaScript Morning
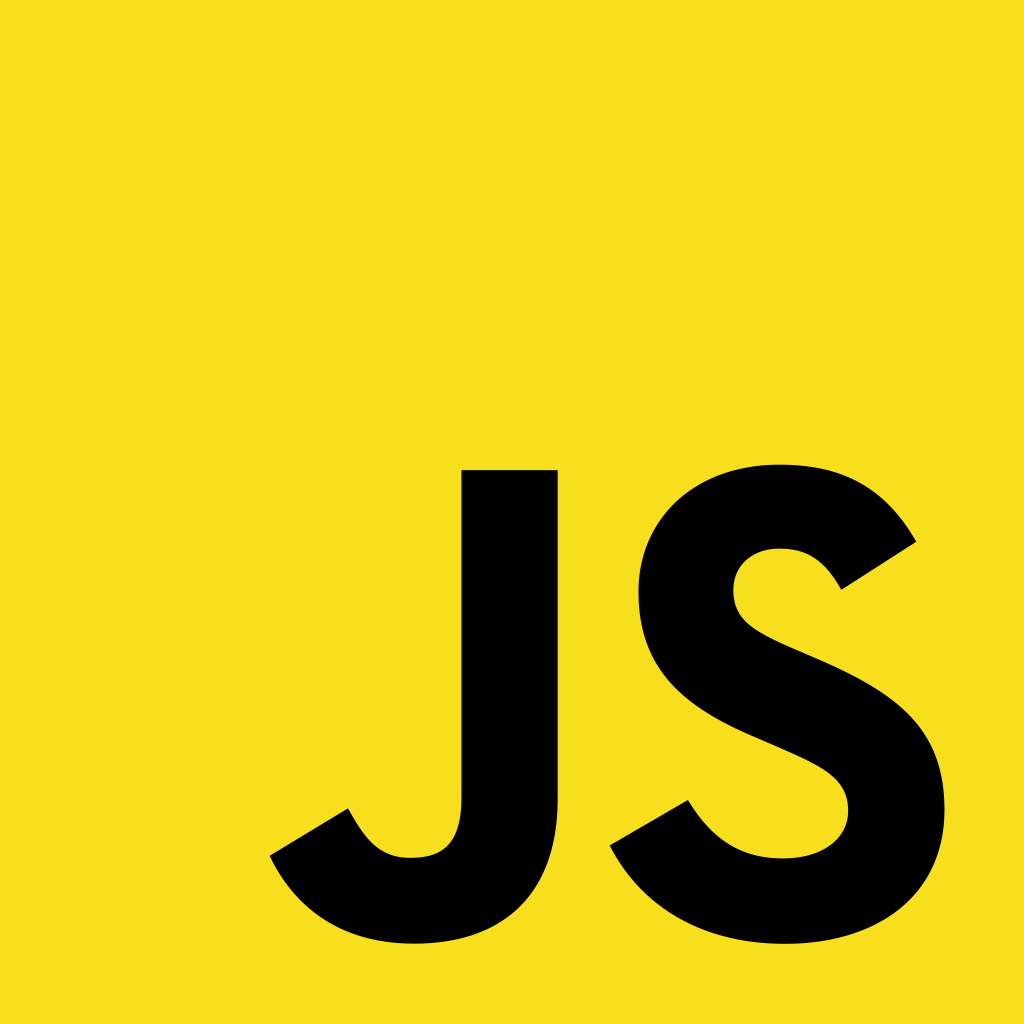
The last two weeks we’ve been digging into the basics of JavaScript, writing functions that are linked to a js file, creating alerts and accessing the DOM to change elements.
<script>
document.getElementById("demo").innerHTML = "Hello World!";
</script>
The easiest way to get the content of an element is by using the innerHTML
property. The innerHTML
property is useful for getting or replacing the content of HTML elements in the DOM
One example we created was a simple counter. If you use the inspector you will be able to see how you are changing the DOM everytime you click the button.
Let’s up the ante and add some logic. Perhaps we only want an alert message to happen when our user gets 10 clicks.
In plain english we might say “if the user clicks the button 10 times, display an alert”.
We can write this in code pretty easily with a conditional statement. We also want to pass along what is inside the HTML to the alert statement.
In this case we have a variable and we might want to add that variable into a string. A string is a group of words – like “our count is now:”
Template literals provide an easy way to interpolate variables and expressions into strings.
The method is called string interpolation. Template literals are used by using the key above your Tab key
The look like this: “
let counter = 5
function count() {
// counter = counter + 1; same as below
counter++;
document.querySelector('h2').innerHTML = counter;
if (counter % 10 === 0) {
alert(`The count is now ${counter}`)
}
}
//you can see ${counter} is how we add our variable to the string
// %10 is called a modulus. We are going to divide the variable by //multiples of 10 and when we have a remainder that is equal to 0, an //alert will pop up
In addition to writing some basic JS, we’ve been adding our JS to the HTML document. This is not really best practice. The better choice is to keep all of the JS in your JS folder.
You can access your JS with Event Listeners – also called Event Handlers – so that the HTML and JS are not mixed.
On the web events are fired inside the browser window, and tend to be attached to a specific item that resides in it. This might be a single element, a set of elements, the HTML document loaded in the current tab, or the entire browser window. There are many different types of events that can occur.
For example:
- The user selects, clicks, or hovers the cursor over a certain element. (we’ve done this)
- The user chooses a key on the keyboard.
- The user resizes or closes the browser window.
- A web page finishes loading.
- A form is submitted.
- A video is played, paused, or ends.
- An error occurs.
document.getElementById("pizza").onclick = count;
//.onclick is the event listener and count is the name of the function to run
*One problem we might run into with our code is the order in which everything loads. If the button does not count – it could be that the JS has loaded before it finds a button with the id of “pizza”. We can work around this by where we place our javascript link in our HTML document. We can also create an event that will load all of the JS before executing any code.
//at the top of your JS document run the onload event to load all of the JS //before running any of the JS
window.onload = function () {
//all of your JS code will run inside this area...
}
Let’s do an example of user filling out a form and then having the code respond to our user. This is pretty common in Web design.
//HTML Code
<form >
<input autofocus id="name" placeholder="Your Name" type="text">
<input id="sub" type="submit">
</form>
//JS Code
document.querySelector("form").onsubmit = function () {
const name = document.querySelector("#name").value;
alert(`Hello ${name}`);
}
10:35 Break
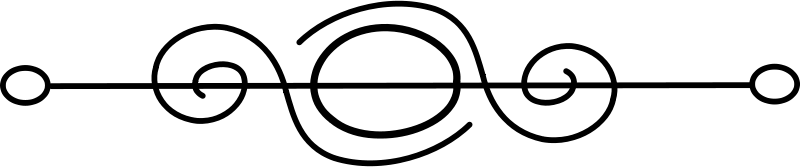
10:45 Visitors or Grid Mark-Up
Drop box for CodePen design
Use the following page as your design template. Re-create using CSS grid. Don’t worry about the background image. Use a color instead. You can get the images and text from the webpage.
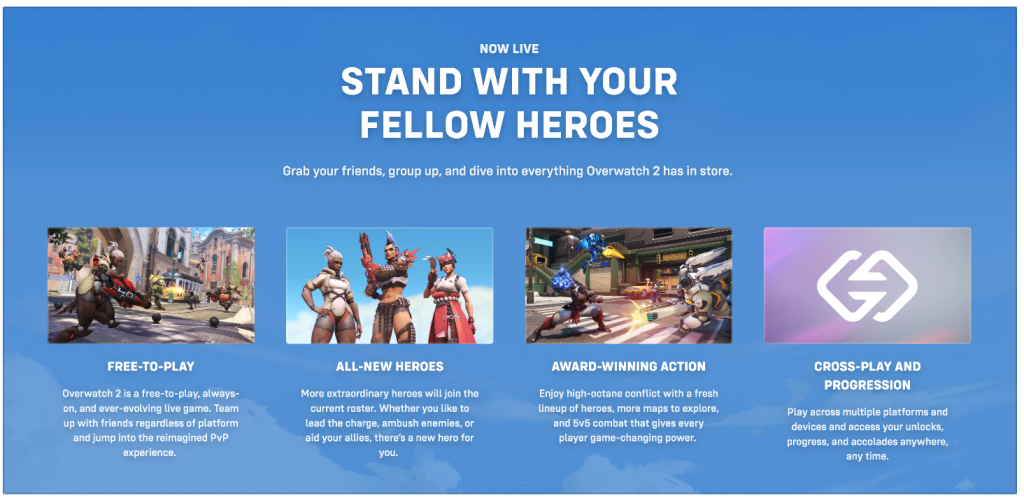
11:30 CSS Grids Team Project
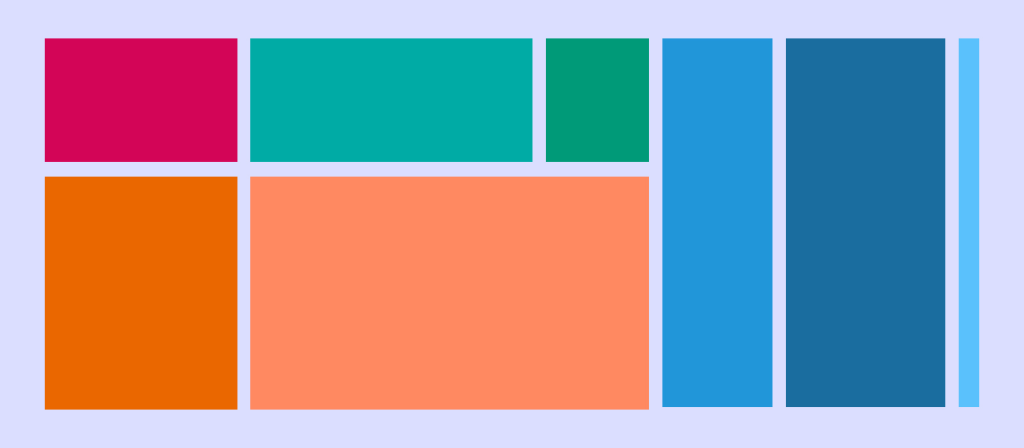
CSS Grid Comic – Each team will have 3 members.
Your Goal: Work as a team. Create a Comic. Output using CSS Grid for layout
- Project Manager / Problem Solver / Scrum Master- The project manager is the organizer, the speaker for the group and the one person I will count on to give me an update and for keeping the project in scope. This person is going to keep the project moving, on time, in scope and will help out the other two roles as needed.
- Artistic Director / Story Creator / Creative Director– responsible for the paper mock up of the comic, color palette and creative aspects of the design
- Front End Coder – your job is to take the design from the artistic director and turn it into the code necessary to complete the project. HTML / CSS and full validation. Strong and creative coders. Responsible for files/publishing
This is a team deliverable project. Everyone should be working – all the time! You will have (3) 45 minute work sessions. (Today, Wednesday, Thursday).
Project is due on Monday, December 5th. Project Managers will present the project
To be successful, you do not have to wait for a design to code. Just follow the deliverables:
- An original comic (9 different size panels)
- Layout using CSS Grid
- Filename CSSComic
- css Folder
- images Folder
- index.html page
- A wireframe / layout of the comic with details /art for each panel
- You may go analog or digital for your wireframe and story
- Custom font(s)
- One breakpoint at 480px for mobile devices (look it up if you don’t know how to do this)
- Valid HTML and CSS (1 point off for each error)
- Published to each members server – exact filenames please
Inspirational CSS Grid Designs
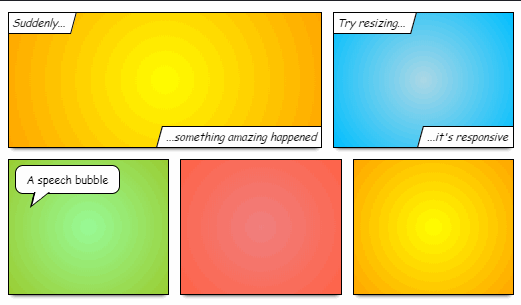
speech bubbles and text can be organized using FlexBox
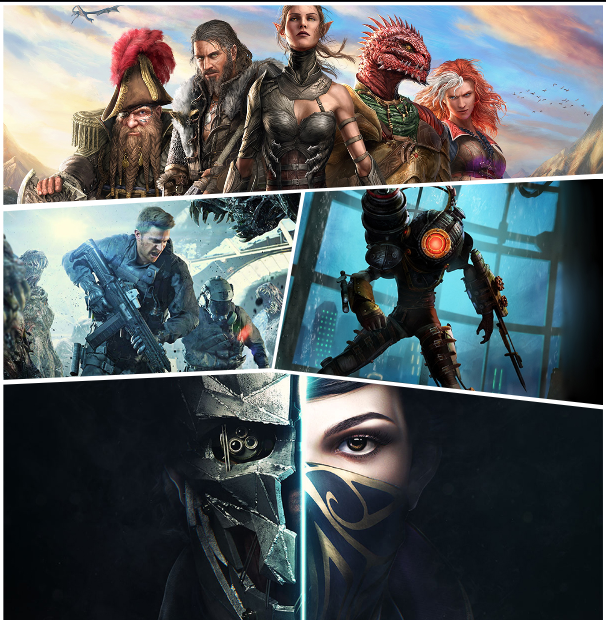
This isn’t quite a comic, but comics often have uneven containers
House Stark
- Bevins – Artistic Director
- LaMotte – Project Manager
- Blow – Front End Developer/Coder
House Lannister
- Fasching – Front End Developer/Coder
- O’Neal – Artistic Director
- Sample – Project Manager
House Mormont
- Watson – Project Manager
- Spofford – Artistic Director
- Murray – Front End Developer/Coder
House Targaryen
- Cincotta – Artistic Director
- DeCell – Project Manager
- Pine – Front End Developer/Coder
House Baratheon
- Bright – Artistic Director
- Adams – Coder
- Noble – Project Manager
12:15 Lunch
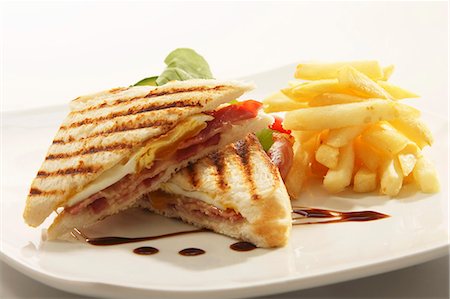
12:45 Literacy in Practice
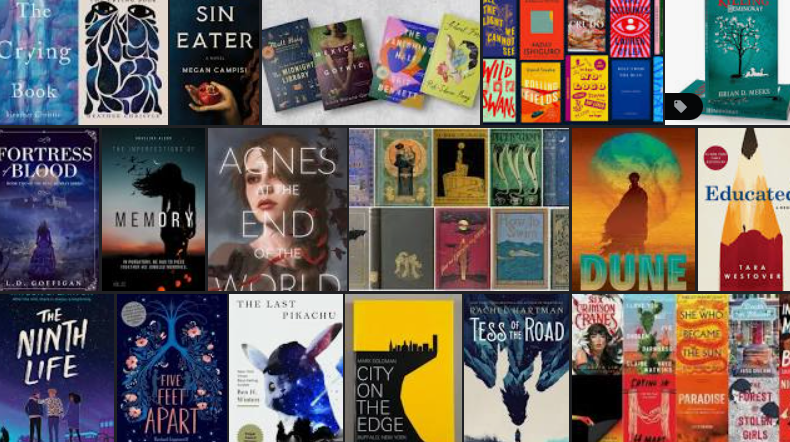
1:10 Break
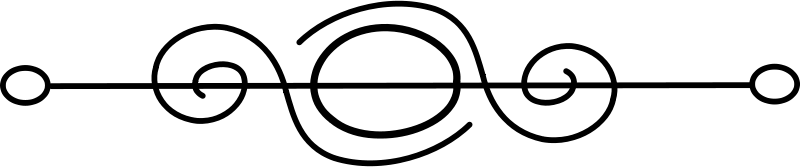
1:20 20% Production Time & Guided Support
- Grid MarkUp
- Famous Photographer’s Research Project – December 8th
- 20% Week 13
- CSS Grid Comic
- Parallax and FlexTradingCards now overdue