Class hours: 10:05 – 2:45
Mr. Bohmann | wbohmann@ewsd.org
10:05 Today’s Notes & Attendance
Welcome to Week 31
After this week, you are on vacation – April 20th – 28th
WorkKeys Testing: Richard & Ariel – Wednesday, April 17th – MWing (below Library m-118)
10:10 Mail Check
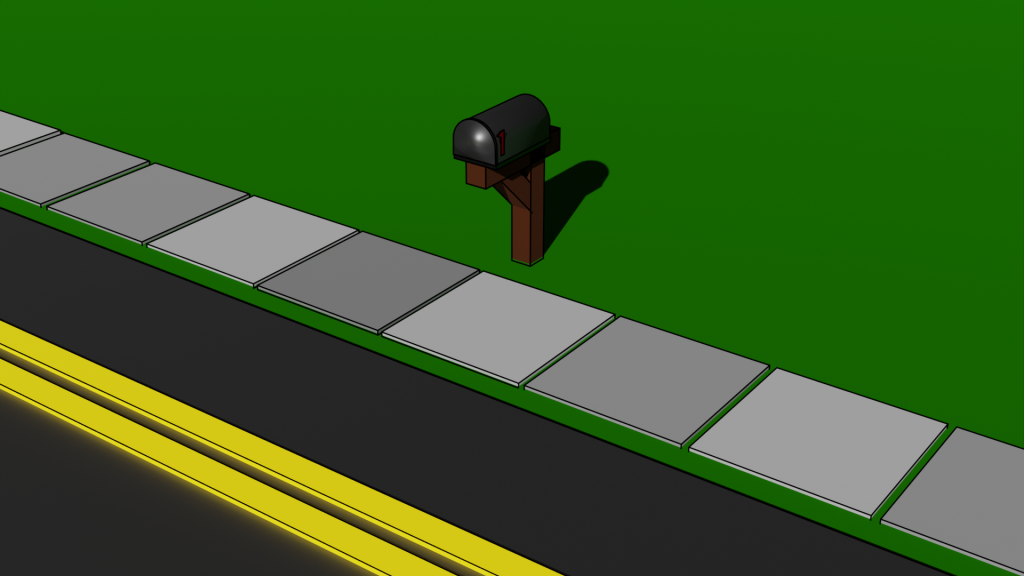
10:15 Unity
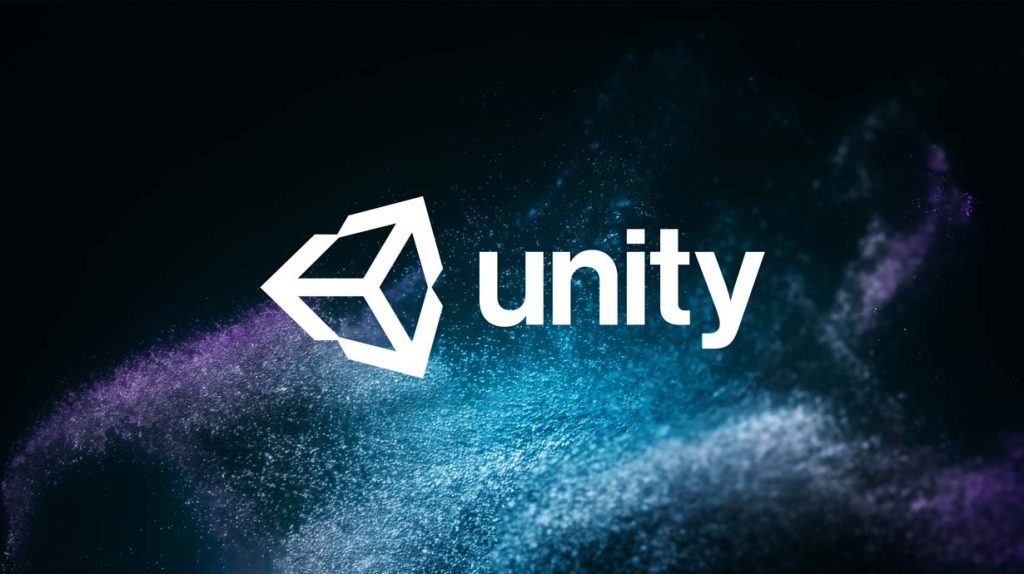
Understanding variables – class vs. local scope variable
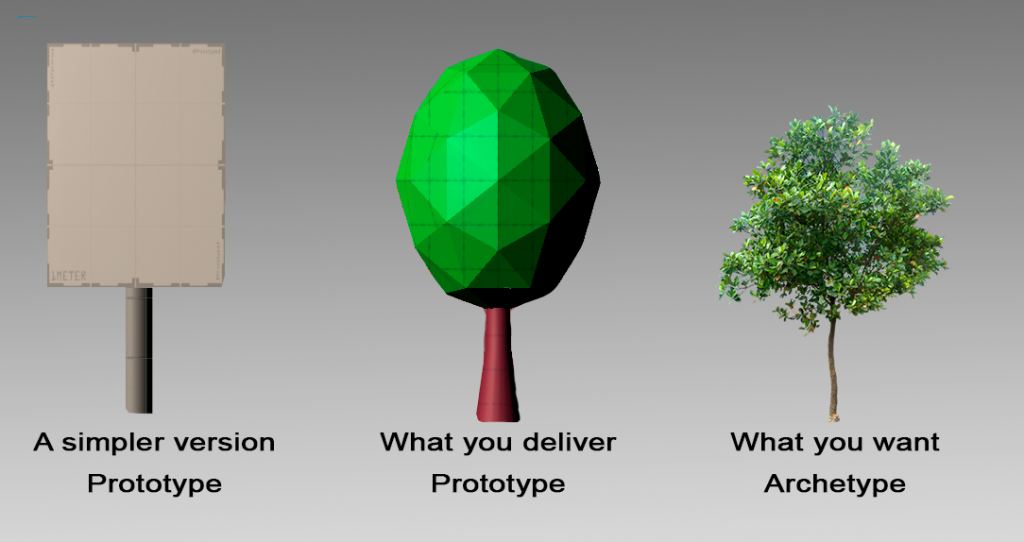
Last Friday we created a simple prototype. Later we an add our own look to customize our game.
We created:
- A Movement Script for your player so that when you use the A or D key, your paddle moves around
- A Ball Script for detecting game over (We coded together with a debug statement)
- Add RigidBody 2D to your pinball
- Use physics materials (that you create) to manage your bumpers
- PlayTest your game
- A Respawn your ball if you lose.
All of our Game Objects have data attached to them. The Transform of a gameObject has 9 pieces of primitive data – like location of x, rotation of z and scale, etc….
We can store information about game object values to calculate health, score points, among other game play options. Each value can be stored in a uniquely named storage area called a variable.
A variable is defined with a specific data type (such as int or float) and a name such as myScore or player1Health. You can change the variable’s contents while the program is running – that’s why it’s called a “variable”. We used lots of variables to store data.
public variables are accessible from game play and from other scripts. Private variables, which is the default for variables are available only for that script.
[SerializeField] is a private variable type but is available from game play to adjust while testing
public class MyScript : MonoBehaviour {
int score; // declare an int called Score
float currentDirection; // declare the float currentDirection
char myInitial; // declare a char called myInitial
string userName; // declare a string called userName
bool isMoving; // declare a bool called isMoving
void Start () {
// numeric data types do not require quotes
score = 8;
// use the "F" suffix to specify a float value
currentDirection = 90.2F;
// use single quotes around individual characters
myInitial = 'A';
// use double quotes around groups of characters
userName = "Will Bohmann";
// boolean values are either true or false
isMoving = true;
}
}
Variable Scope refers to where you place your variables. If you placed your variable in the Start method, it will run when the game begins and then not be accessible again.
We already know that we use variables to provide more code flexibility. In many cases we may want to store mathematical operations in a variable. Let’s look at some common math operations.
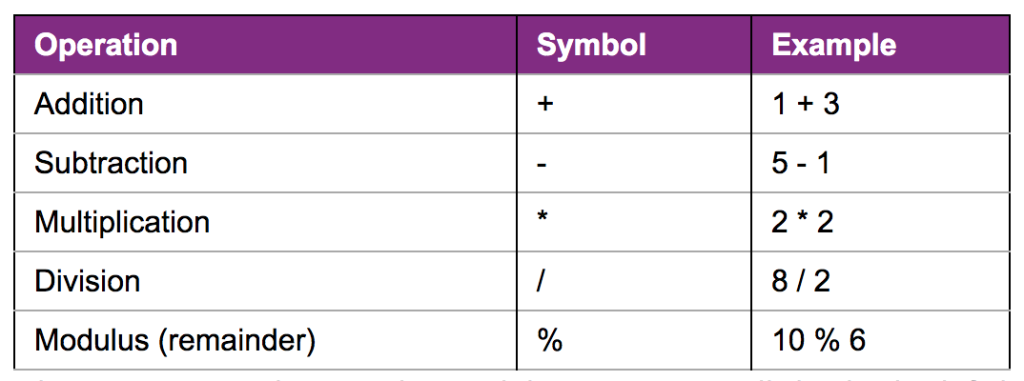
int added = 3 + 4; // added is 7
int subtracted = 3 - 4; // subtracted is -1
int multiplied = 3 * 4; // multiplied is 12
int divided = 12 / 4; // divided is 3
int remainder = 12 % 5; // remainder is 2
Here is some for our paddle. This script can placed on the player paddle. We declared public Class Level variables and one local scope variable. Do you remember which is which?
The following code will limit our paddle crashing into the wall. We can declare and min and max movement value with a float (because it has decimals!)
public float paddleSpeed = 3;
private float hMove;
private float minXPos; //limit to the left
public float maxXPos; // limit to the right
void Start()
{
}
void Update()
{
hMove = Input.GetAxis("Horizontal") * Time.deltaTime * paddleSpeed;
//the following code will create the limits left and right for you paddle //using both the global variables and the local scope variable moveX above
if (transform.position.x + hMove > minXPos && transform.position.x + hMove < maxXPos)
{
transform.Translate(hMove, 0f, 0f);
}
10:50 Break
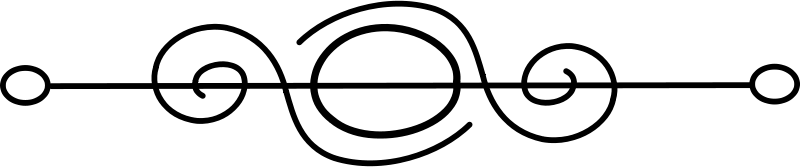
11:00 English with Mx. Yopp
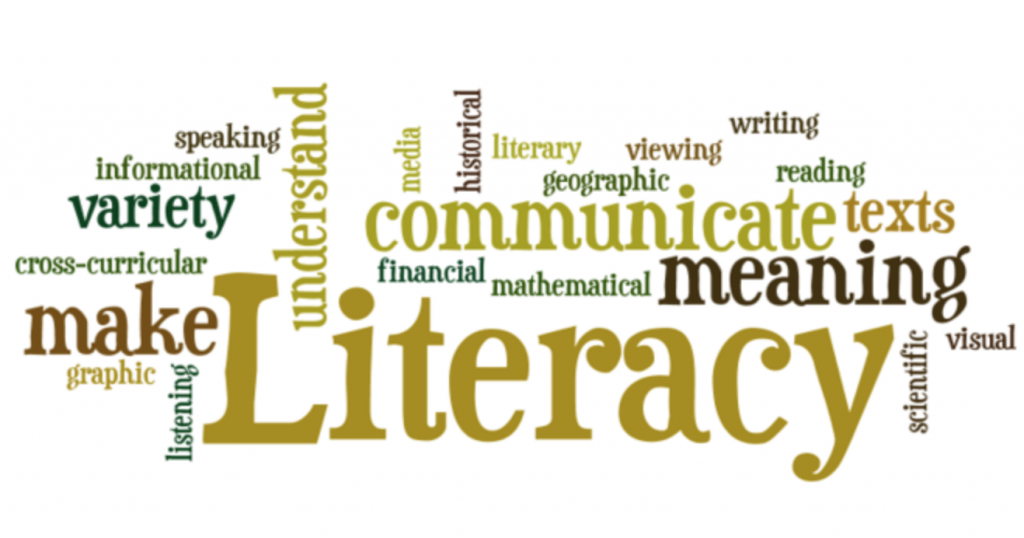
11:50. Pinball – Let’s level up using variables
You can use variables to do important things within your game. For example, many games will need to keep a score. Let’s add a score keeping variable to the pinball game and have the results printed in a Debug.Log statement.
To begin, you will need to declare a variable for score (what type of variable will you use? and where should you put it?). We want the Score to be displayed when the game begins (so we’ll place a Debug.Log statement in the Start() method.
One last decision – which game object should we create the script for?
We’ll also need to make the variable Public so we’ll put it at the top of the script too
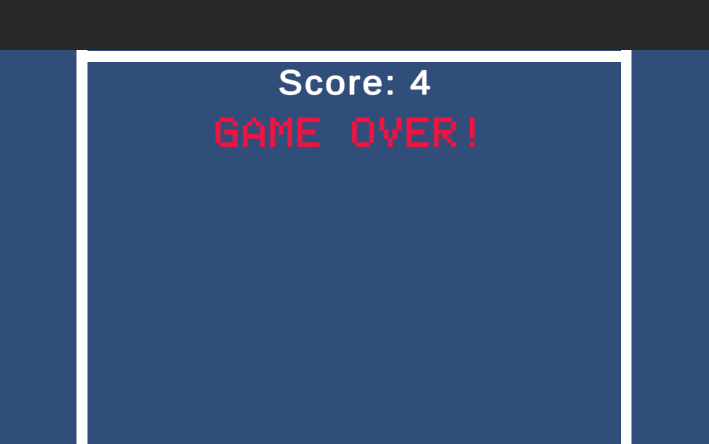
void Start ()
{
// display the current score on start
Debug.Log ("Score = " + score);
}
//score is a great variable name because it is...semantic!
Inside the OnCollisionEnter2D() function, set the score variable value to 1. Then, again use Debug.Log() to display the current score to the screen.
void OnCollisionEnter2D (Collision2D otherObject)
{
// set the score to 1
score = 1;
// display the new score
Debug.Log ("Score = " + score);
}
In the example above, the score just stays at 1. Not entirely what we would want. So, let's look at using some operational shortcuts in our code to how we can calculate the score each time the ball collides with a gameObject that has a collider.
score = score +1 //adds 1 to the variable
score +=1 //same as above, but add what's on the right to the left
score ++ //means add 1 to the score
Instead of using just the Debug.Log function, we could initialize our score variable at the top of our script as a public variable and then as the game plays we can watch the score increase in the inspector.
Let’s Level Up some more: Setting up Score with the UI Canvas – Instruction
Project Details/Deliverables:
- The player’s score should start at 0 when the game begins
- The player’s score should be displayed in the top center of the screen
- Each time the ball collides with (bumpers paddles, walls, the score should increase by 1
- When the game ends, the phrase “Game Over” should be displayed under the score
The big picture – Here is a basic outline of the steps you need to complete the tasks above:
- Add a Canvas and two Text objects (one for the score, one for the game over message)
- Edit the existing BallScript (or whatever you named your script on the ball) and add a score variable and two public Text variables
- Modify the BallScript functions to update the Score and Text variables as needed
- Link the two Text objects to the two Text variables in the BallScript
Easy, right? We can work on the steps together if you are having trouble.
If you get lost, here is a reference worksheet
Your Assignment: Build out your game by improving the prototype to include cool looking bumpers, improve your paddle, make a background.
12:25 – 12:55 Lunch
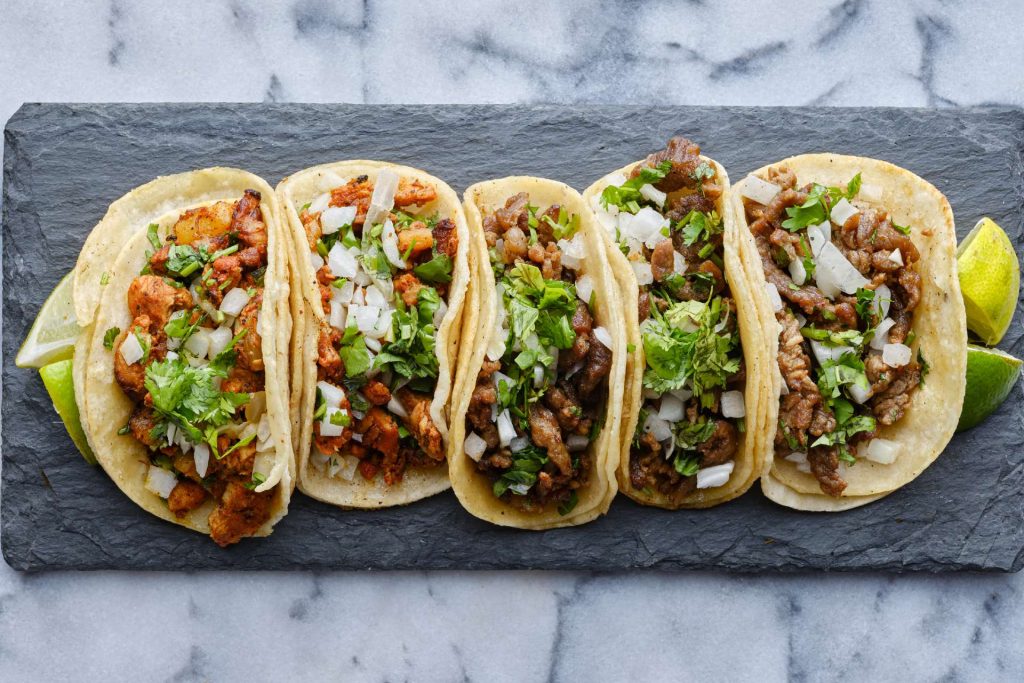
12:55 Independent Reading
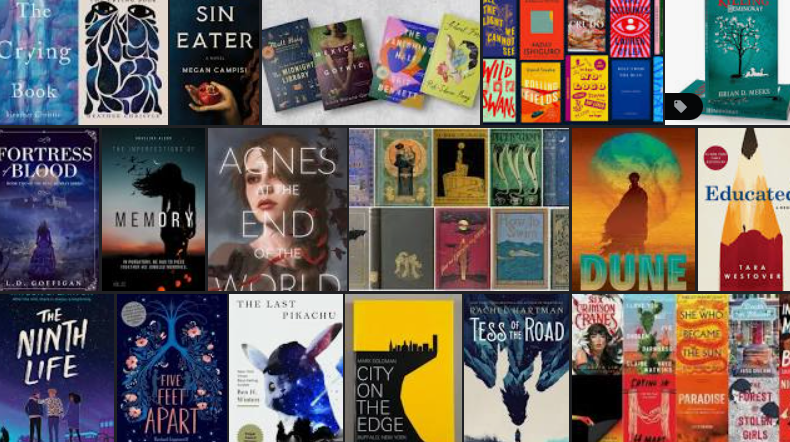
1:20 Break
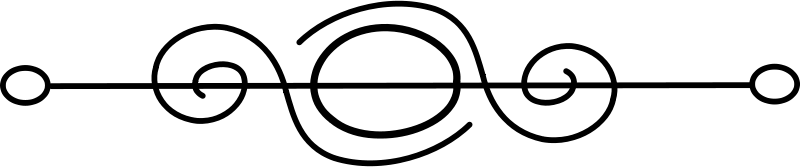
1:30 Design Challenge
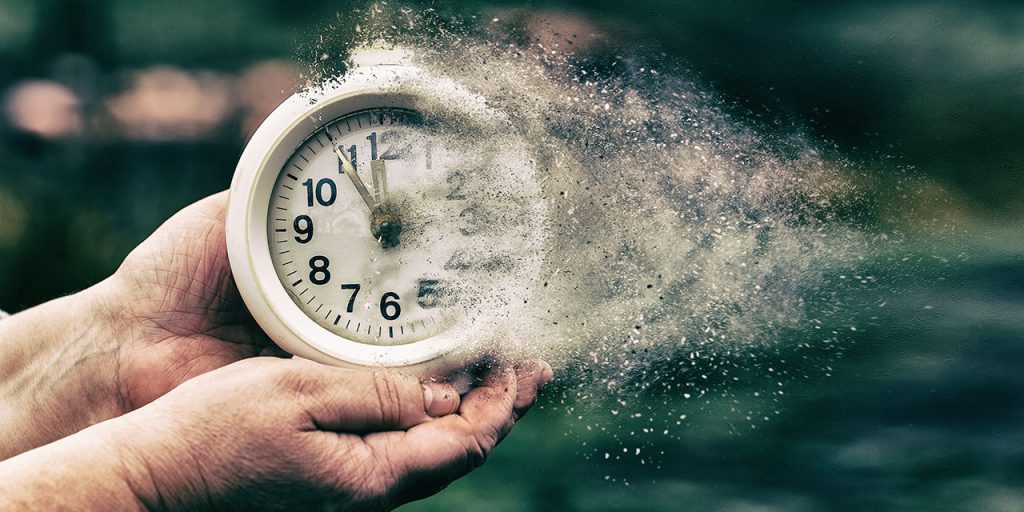
1:55 Production Time and Guided Support
- Help with code from this morning
- Improve your prototype by making your pinball game more interesting to look at.
2:40 Dailies
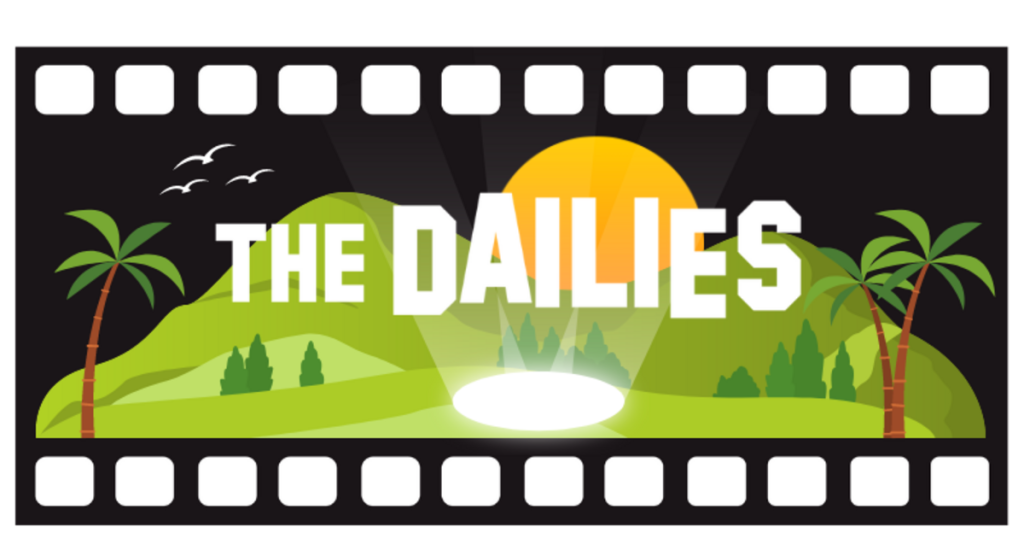
Dailies can be placed in the CAWD2 Dailies Folder on the CAWD2 Public Folders drive