Class hours: 10:05 – 2:45
Mr. Bohmann | wbohmann@ewsd.org
10:05 Today’s Notes & Attendance
Favorite Chip Friday – sign up and bring in your favorite bag of chips to share.
Friday – we’ll be doing some work in the morning, then the afternoon will be relaxing and we intend to go outside. Plan your clothing accordingly. Bring a frisbee, ball, beach chair – whatever will make you comfortable.
10:10 Flow Control Continued….
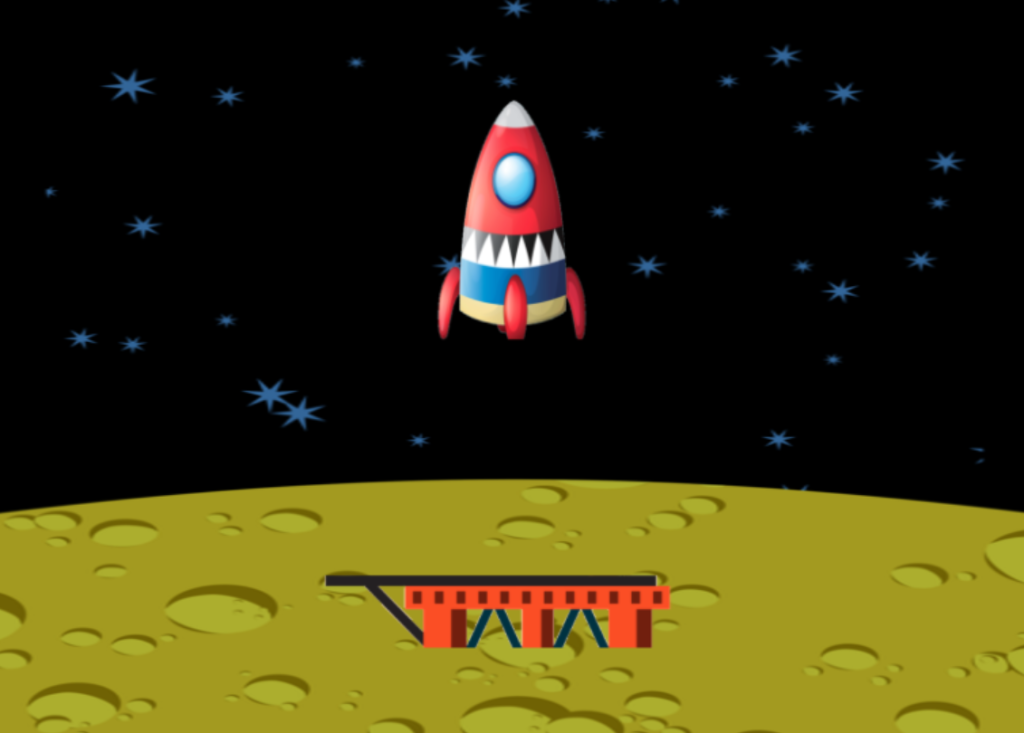
On Wednesday we added some logic to our PinBall prototype that stated if the health was below 1, our game would end and the ball would be destroyed. Let’s continue working with the concept of flow control and logic with the Lunar Lander we made yesterday.
You can use any logical expression inside an if() or else if() statement. So, you can ask complex questions like “is score greater than 100 AND is playerHealth less than or equal to 0?” You can also ask very simple questions such as “is shipStatus equal to 1″? In fact, you may frequently want to ask a series of “equal to” questions about one variable or expression and take some different action with each result.
if (shipStatus == 1)
{
Debug.Log("All Systems Green");
}
else if (shipStatus == 2)
{
Debug.Log("Yellow Alert");
}
else if (shipStatus == 3)
{
Debug.Log("Getting toasty in here");
}
else
{
Debug.Log("Head for the lift pods!");
}
While this if / else-if / else chain will work fine, C# provides a cleaner way to test if one variable or expression is “equal to” many different values.
The if() statement is not the only way to control your program flow. The switch has decision-making power similar to an if / else-if / else chain. Switch statements are useful when you want to compare one value against a series of other values and execute different code on each “equal to” match.
The “switch” statement
The switch() statement will accept one variable or expression to test, and then allow you to write different blocks of code for each possible value or “case“. Let’s rewrite our example using the switch statement.
switch (shipStatus)
{
case 1:
messageText.text = "All Systems Green";
break;
case 2:
messageText.text = "Yellow Alert";
break;
case 3:
messageText.text = "Getting Toasty in Here";
break;
default:
messageText.text = "Head for the Life-pods!";
break;
}
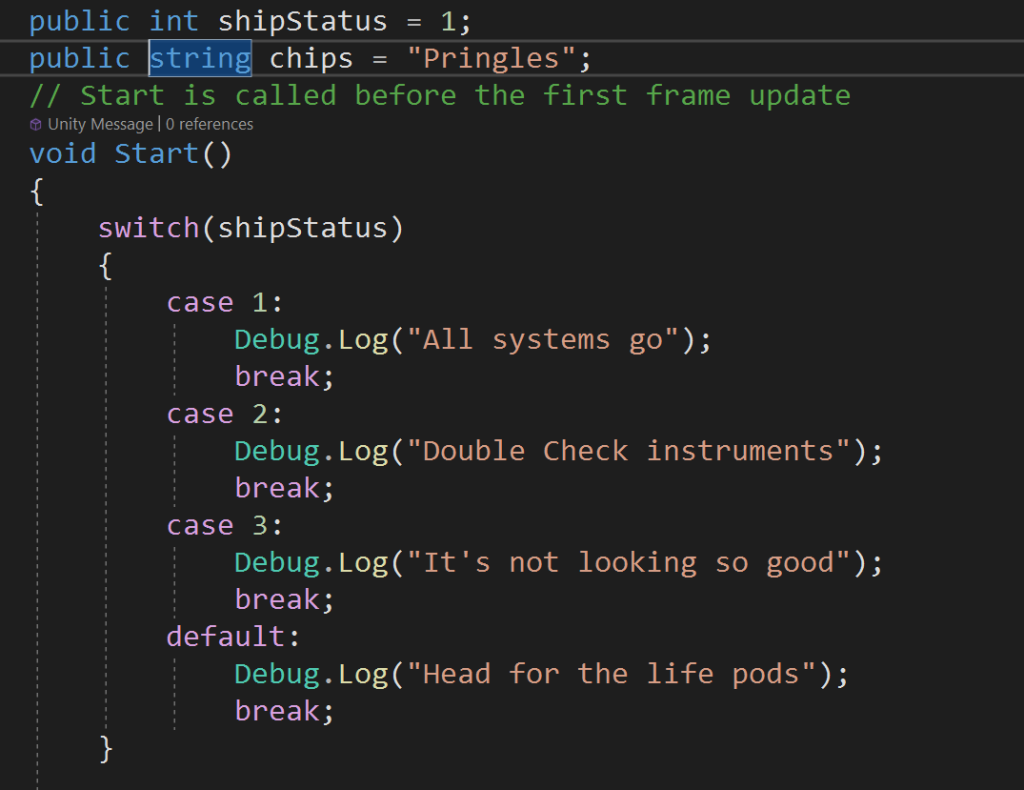
What’s going on here? The switch statement begins with the switch keyword, followed by an expression in parentheses. The expression must evaluate to an integer number such as byte, int or long, or a char or string. In our example, we simply used the variable shipStatus, which has been declared elsewhere as some integer type. Important – You cannot switch on a fractional data type such as a float.
Let’s open the Lunar Lander project and see how this works.
The entire body of the switch statement is contained within the opening and closing curly braces.
Within the switch, curly braces are one or more case statements. A case represents one possible value of the switch expression and the code you want to run when the expression is “equal to” that value.
You can add as many case statements as you like; one for each value you want to check. You can also add a default case at the very end. This is similar to the else statement after an if() expression. The default case will be executed if no other case value matches (see above code example for a default case)
switch (health)
{
case 3:
Debug.Log("Your Health is Excellent");
break;
case 2:
Debug.Log("Your Health is declining");
break;
case 1:
Debug.Log("Your are almost finished!");
break;
case 0:
Debug.Log("You are dead");
break;
}
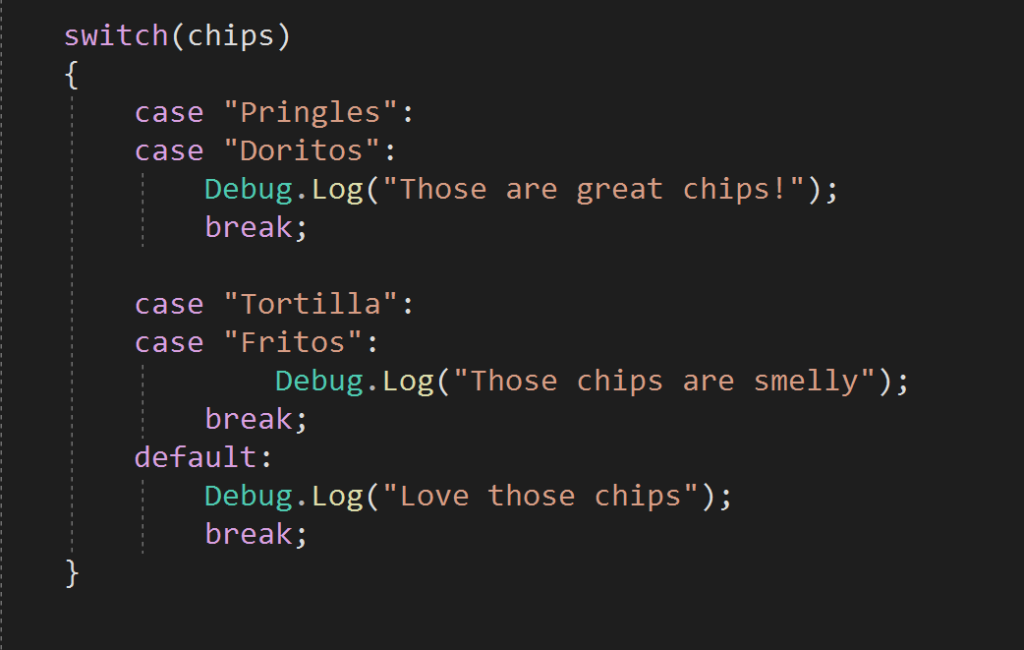
Now that we have seen this done. Let’s look at the if / else statements we made in our Lunar Lander and clean them up with a switch statement. Remember when I said we cannot use floats?. A float is a (fractional) value, and you can’t use fractional data types directly in a switch statement.
So, the first thing you’ll do is convert the float to an integer data type. You can cast (convert) a fractional data type to an integer type by placing the new data type in parentheses in front of the fractional value.
// explicitly cast fractional value to integer
int shipLandingstatus = (int)relativeVelocity; // converts float to int & now we can use in switch statement
10:50 Break
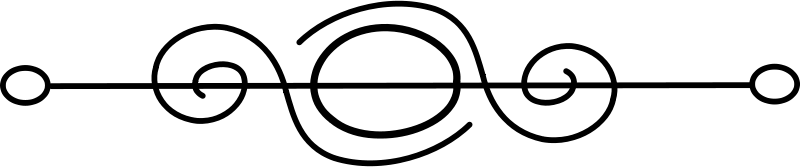
11:00 The Game Developer’s Journal
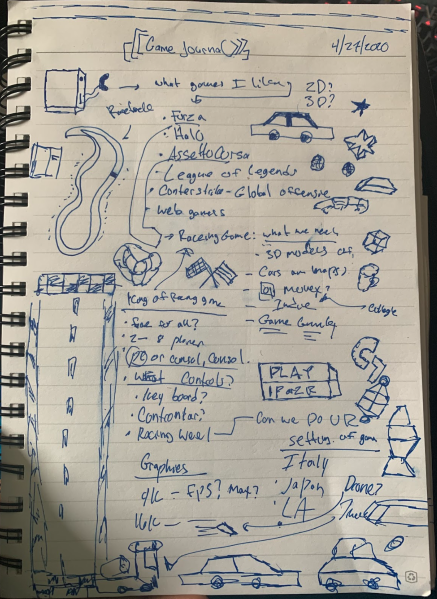
A Game Developer’s Journal is a tool to organize and record the development process of your own ideas and work. It’s like a diary for your game thoughts and ideas. Some people keep journals for business ideas, some for artwork, you get the picture.
Your journal can look like anything you want it to. The easiest route is to your first journal is to find a notebook, legal pad or bound stack of paper. Whatever you choose, protect it and personalize it.
You can include drawings, doodles, color whatever. Game developers & designers need to keep focused to move projects from ideas to execution. The journal is your collect-all and fail safe to ensure this happens.
As such, be sure you:
- Record all your ideas and state how you got them . What was your inspiration?
- Write about the challenges you experienced during the process and how you resolved
them. - Do not erase notes or entries, but revise and expand upon them .
- Add sketches and drawings to make things clear .
- Put a date each time you start a new entry. This will help you track progression.
- Jot down your ideas and sketch them out when appropriate . Sometimes it is easier to draw pictures that illustrate the connections between ideas, sequences, or events.
Your task: Create your very own Game Developer’s Journal and bring to class. Due Friday for an easy, easy grade!
11:10 Unity – Pinball Update
Let’s take a look changing out paddle out to flippers and setting up some new movement code.
We’ll use a HingeJoint2D. With some trial and error, we can get these to work.
- Set Up Pivot Point for each flipper in the sprite editor
- Add rigidBody 2D to each flipper
- Add polygon collider2d to each flipper
- Add Hinge Joint 2D to each flipper
- Add a Left Flipper Script to left flipper
- Add a Right Flipper Script to right flipper
- Assign input keys
- Add torque to the hinge
- Adjust the hinge limits
- Don’t forget to attach the scripts
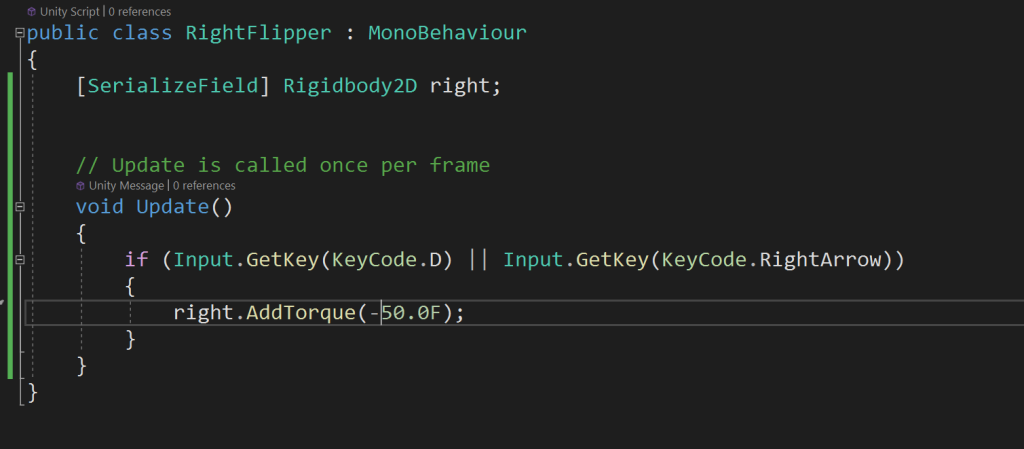
Left Hinge Limits: Lower:-50, Upper: 0
Right Hingle Limits: Lower: 0, Upper: 50
12:25 – 12:55 Lunch
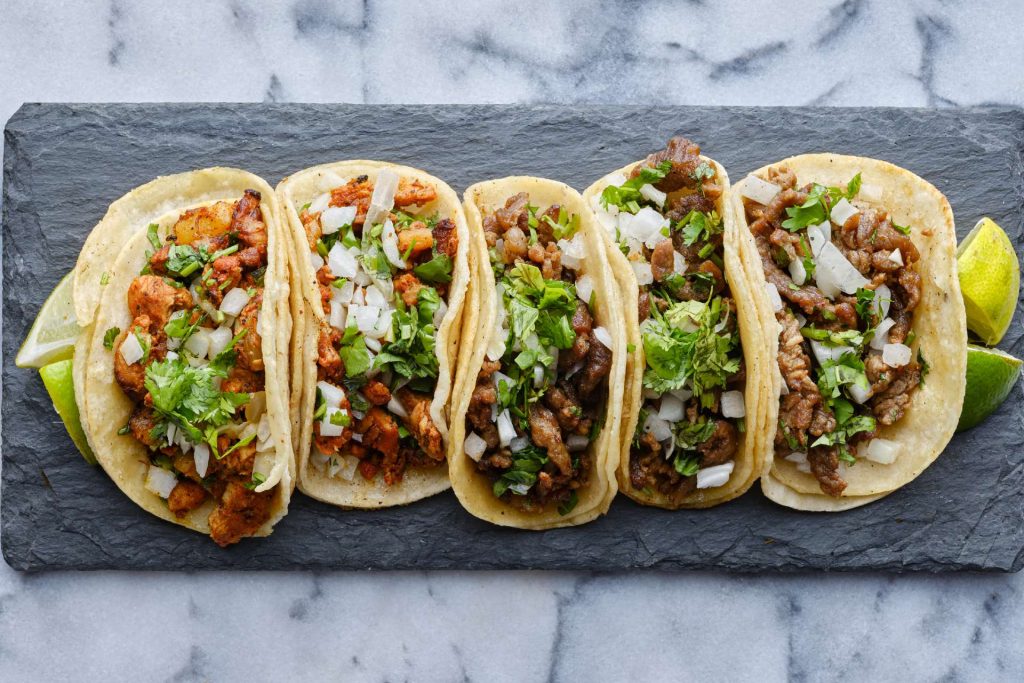
12:55 Independent Reading
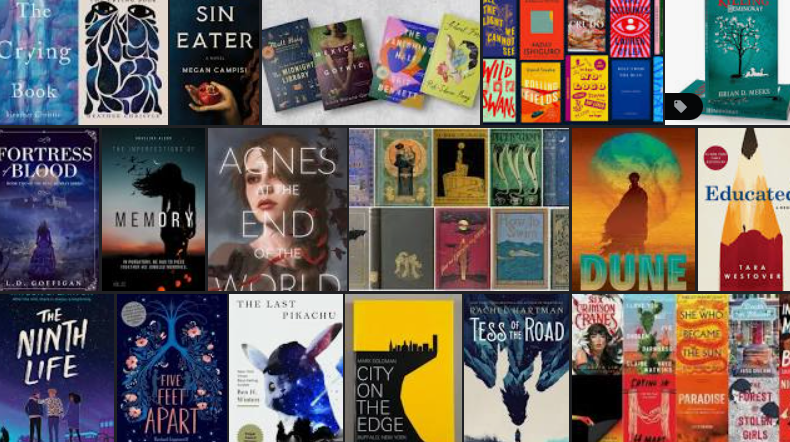
1:20 Break
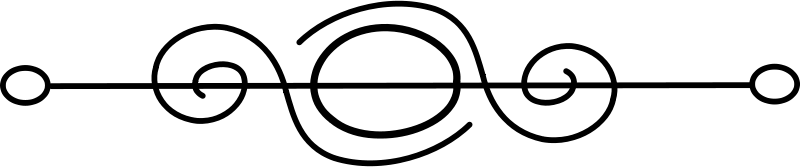
1:30 Design Challenge
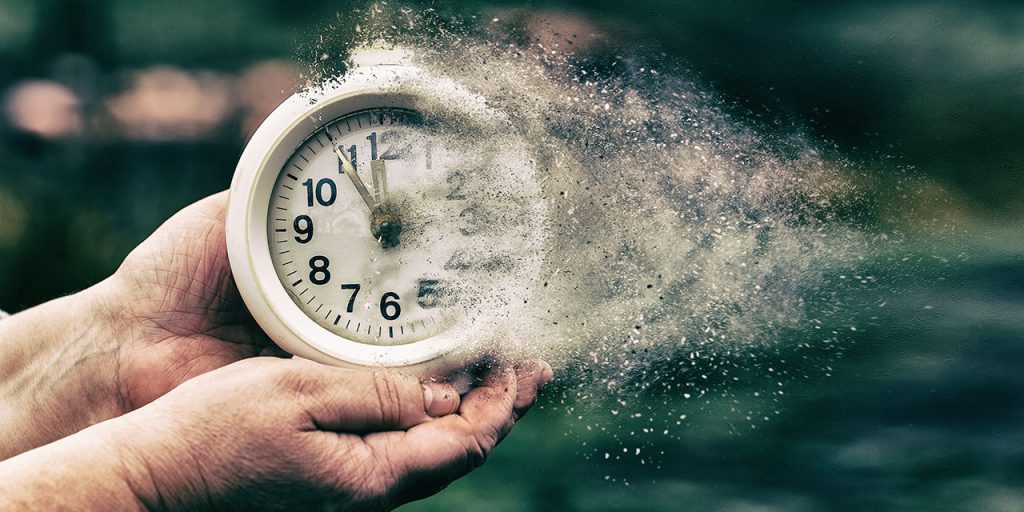
1:55 Production Time and Guided Support
One Button Game proposal
Game Dev. Journal
2:40 Dailies
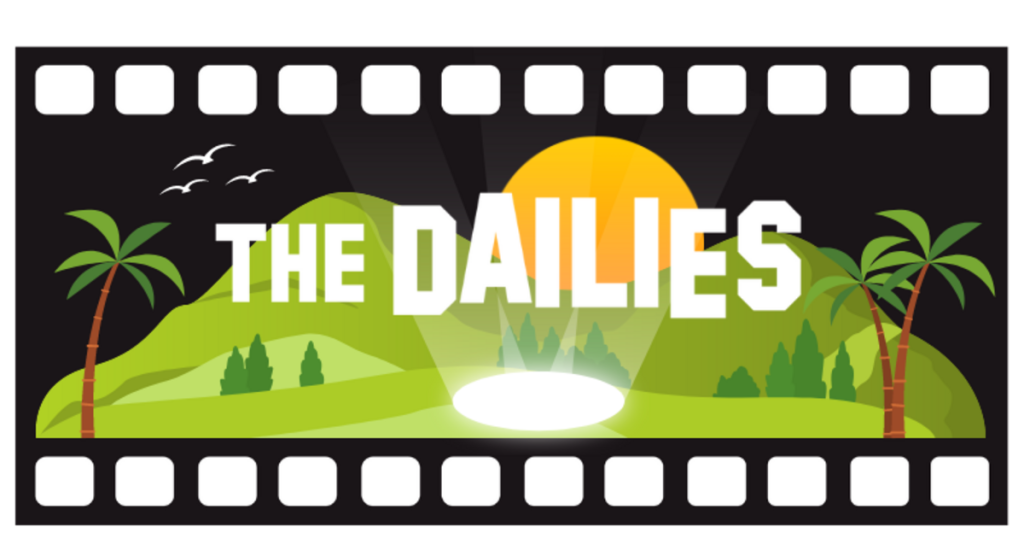
Dailies can be placed in the CAWD2 Dailies Folder on the CAWD2 Public Folders drive