Class hours: 10:05 – 2:45
Mr. Bohmann | wbohmann@ewsd.org
10:05 Today’s Notes & Attendance
Week 31 and a Tuesday – 4 more days to vacation!
Congratulations again to all of you SkillsUSA participants. For those that earned medals, I have them for you and will distribute.
Gold Medal Winners – there will be a meeting about Nationals on April 30th @6pm.
10:10 Logical Expressions
Normally, a pinball game like the one we’ve made will only add to the score when a bumper is hit. You may even want to assign different point values to different targets. To support this kind of advanced scoring, you would need to examine the actual objects involved in the collisions and make decisions about how many points to add to the score.
The general process of selecting statements to run is called flow control.
An expression is a part of a code statement that is evaluated to produce some answer. You are already familiar with mathematical expressions like 4 + 4 that will produce an answer such as 8.
int speed = 4 + 4; //score is a mathematical expression
To make decisions in your code, you need a different kind of expression – a logical expression. That means questions you ask in your code have two possible answers – true (yes) or false (no).
So, you remember from our conversation yesterday that a Boolean (bool) data type is used to store a true or false values.
bool alive = health > 0; // health > 0 is a logical expression
There is no mathematical operation, but instead, this is a comparison that looks at the value on both sides of the “>” symbol in the middle. The answer is either true or false.
There are several comparison operators:
- == (equal to operator) the result is true if both sides are equal
- != (not equal operator) the result returns true is two sides are not equal to each other
- < (less than operator)
- >(greater than operator)
- <=(less than or equal to operator)
- >=(greater than or equal to operator)
There are several logical operators:
Logical Operator | C# Symbol | Example | Description |
---|---|---|---|
and | && | A &&B | Returns true if both “A” and “B” is true, or false otherwise |
or | || | A || B | Returns true if either “A” or “B” are true, or false otherwise |
exclusive or | ^ | A ^ B | Returns true if either “A” is true and “B” is false, or vice-versa |
not | ! | !A | Returns true if “A” is false or false if “A” is true |
Let’s Practice. If you had two variables named score and health, how would you write a logical expression that was true if both score was greater than 100 and health was equal to 0?
Let’s Practice more….
void Start ()
{
int value1 = 50;
int value2 = 100;
int value3 = 100;
int value4 = 200;
bool result1 = value1 == value2;
bool result2 = value2 == value3;
bool result3 = value4 > value3;
bool result4 = value1 <= value2;
bool result5 = value2 != value3;
bool result6 = result1 && result2;
bool result7 = result1 || (value4 < 300);
bool result8 = !result1;
bool result9 = !(value3 == 100);
bool result10 = value1 + value2 >= 150;
bool result11 = value2 - value1 * 2 == 100;
Debug.Log ("result1 = " + result1);
Debug.Log ("result2 = " + result2);
Debug.Log ("result3 = " + result3);
Debug.Log ("result4 = " + result4);
Debug.Log ("result5 = " + result5);
Debug.Log ("result6 = " + result6);
Debug.Log ("result7 = " + result7);
Debug.Log ("result8 = " + result8);
Debug.Log ("result9 = " + result9);
Debug.Log ("result10 = " + result10);
Debug.Log ("result11 = " + result11);
//Write on a piece of paper 1-11 and declare if the expressions //will return true or false
}
Let’s check our answers…. and then let’s apply what we learned and see if we can come up with some health for the pinball game.
10:50 Break
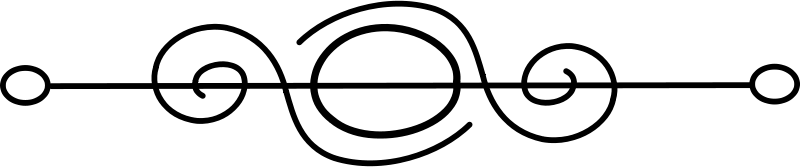
11:00 English withe Mx. Yopp
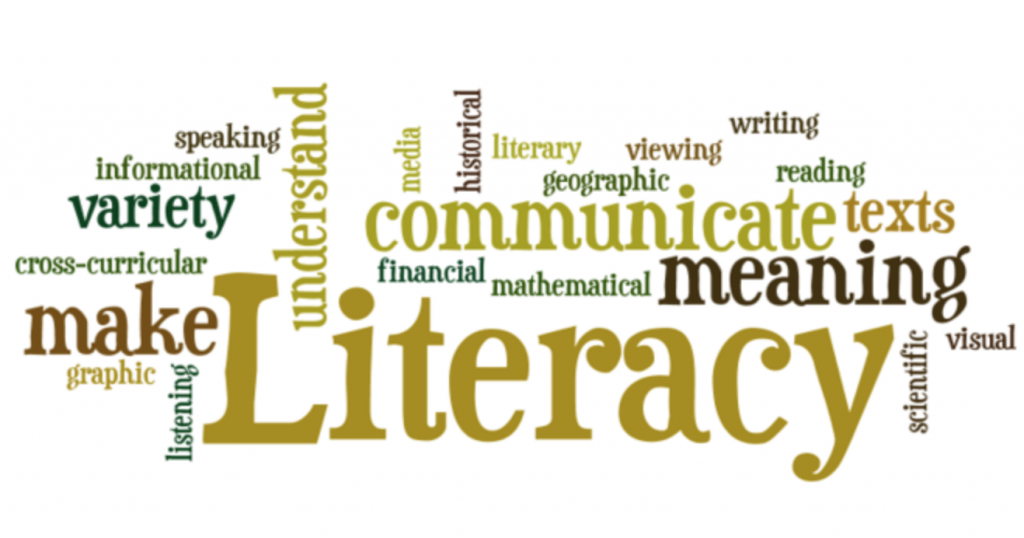
11:50 Core Game Loops and Game Mechanics
Most well thought out games have a core game loop. Every action and feature set is fed back into this core loop. For example, in Sea of Thieves you explore a open world, plunder ships and amass your fortune.
In Don’t Starve you explore, collect food, shelter and try to keep yourself alive by not freaking out (losing your mind), getting sick or starving. The core loop have a night and day cycle.
Every game has a core game mechanic. In a platform game this is usually jumping (SuperMario) or in CSGo it is shooting. The core mechanic is an purposeful interaction that happens most frequently. What’s Flappy Bird’s Mechanic? Halo – running, jumping and….shooting – the shooting is a major part of the core of the game – If you couldn’t shoot in Halo, it would be no different than a first person Sonic the Hedgehog.
One of the best ways to figure out what a game is all about is to sit down and play some. Let’s do just that. Spelunky Slither, deip.io and then take a stab and identifying what the game is all about.
12:25 – 12:55 Lunch
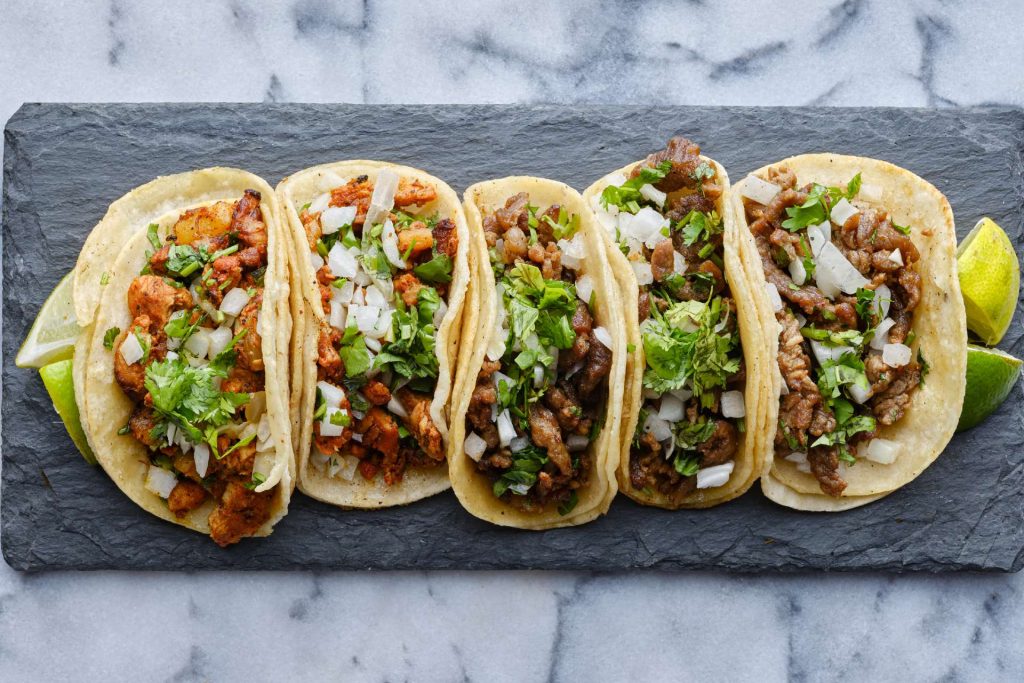
12:55 Independent Reading
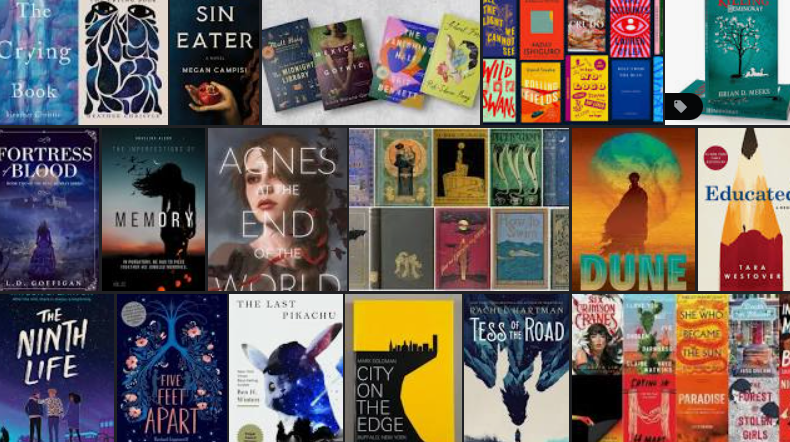
1:20 Break
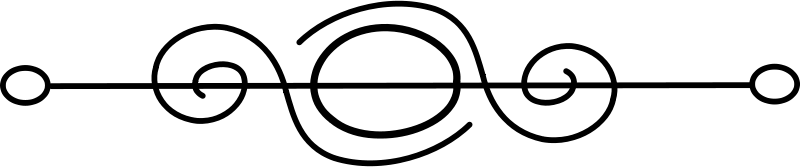
1:30 Design Challenge
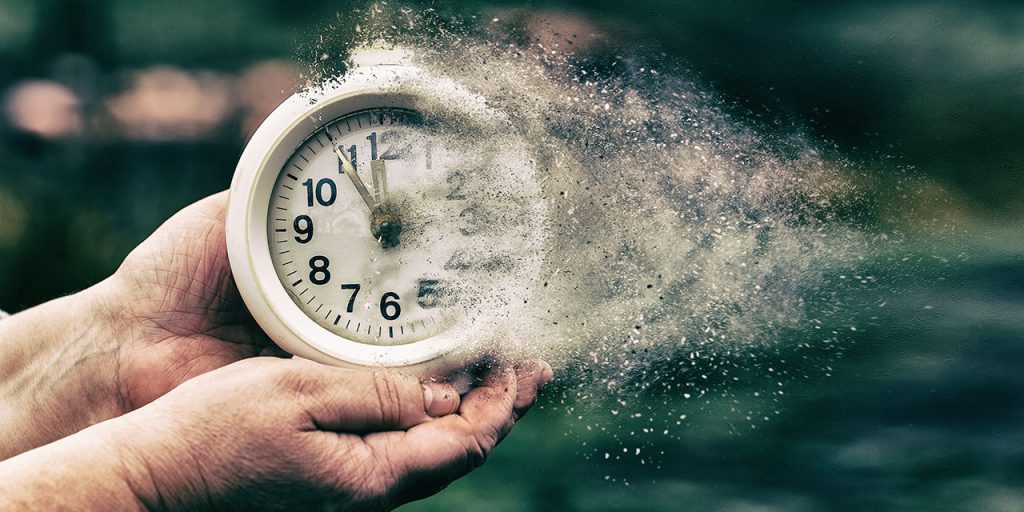
1:55 Production Time and Guided Support
Adding Health to Pinball – Answer:
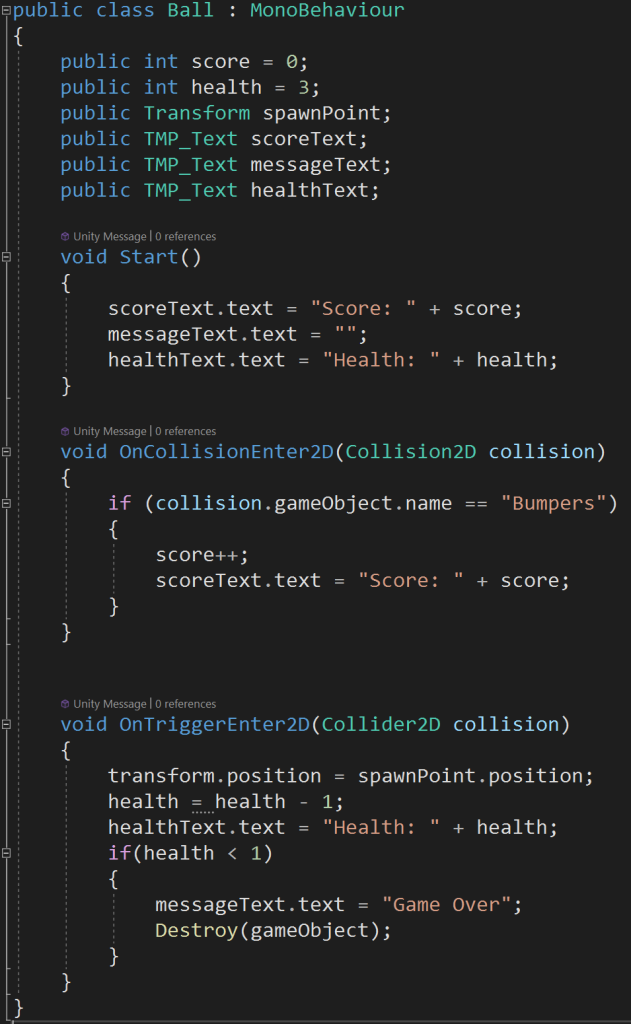
2:40 Dailies
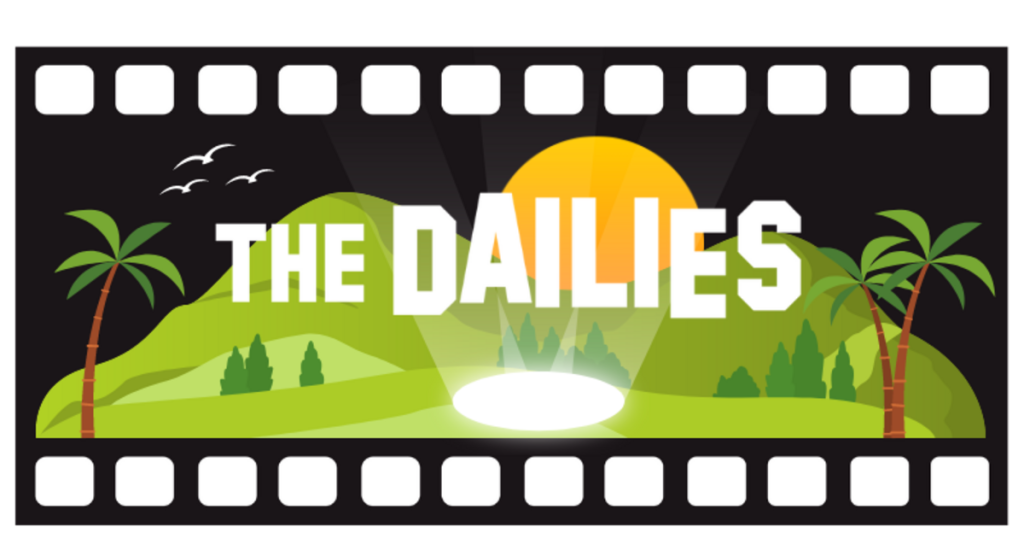
Dailies can be placed in the CAWD2 Dailies Folder on the CAWD2 Public Folders drive