Class hours: 10:05 – 2:45
Mr. Bohmann | wbohmann@ewsd.org
10:05 Today’s Notes & Attendance
Today is a Wacky Wednesday
Call Backs: Philip – 12:25 Portfolio Presentations
SkillsUSA Test for National participants – tomorrow – check in the classroom first
Dissect Your Favorite Game: Aiden, Philip – we still want to hear from you…
Equipment: Please return to me ASAP
10:10 A Day at Work – Video Game Design
Getting to the core of design through gameplay
A Day in the Life of a Japanese Video Game Designer
By Friday many of you may be ready for some testing, or at least what you have built so far. By Monday, everyone will be testing.
Testing works best when you know what you are testing. The UI? The Core Mechanic? The Storyline? …..
Just like we did with web development, we are going to gather feedback. What kind of feedback do you want to know about. What do you want to know to make improvements while still in the early stages of your game? It’s not too early to think about this. Maybe include one additional question – In this game I wish……
You can ask your testers for feedback on specific parts of your game you want them to test. By early next week you will have feedback that you can use as maintenance for your game. (If you are on a team, work together to gather questions).
After game feedback, you will have a short sprint to incorporate changes, suggestions and make improvements before presenting your final game.
Assignment:
Part 1: Create a list of questions you’d like to ask during testing. Create a Questionnaire on Google Forms for easily gathering feedback. Share a link to your questions in Google Classroom.
Part 2: Every game will be tested to provide user feedback. That feedback will be summarized with actionable steps. It will count as 100 points. You will create the summary and actionable steps on a google doc. This might look nice as a table. This summary will be submitted to Google Classroom
CAWD Game Studio
OBS Walkthrough – Core Mechanic
The Task: Use OBS to demonstrate the core mechanic for your game. Use Premiere to create titles or use voice over to describe what the mechanic is. I want to see it in action, and I want to hear or read about it when I view your clip. While you play your game, explain what you are doing, and how it is the core mechanic of your game. For full credit we need to see the completed mechanic. If you don’t want to use your voice – use callouts/text
It is ok that you are in prototype mode. Doing a core mechanic overview give us the pitch/purpose of your game. I am going to catalog these videos to share with future classes.
Remember The core mechanic in a game will usually be the purposeful interaction that occurs the most frequently. Jumping, collecting, sliding, farming, etc…..
When complete label it “CoreGameMechanic_LastName.mp4”
This assignment is due by Friday Afternoon – end of day.
10:50 Break
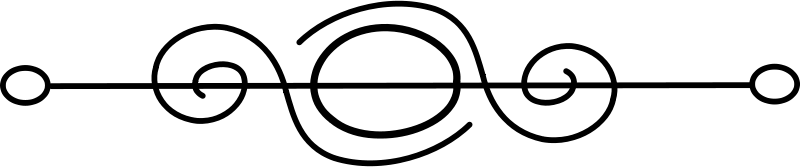
11:00 CAWD Game Studio
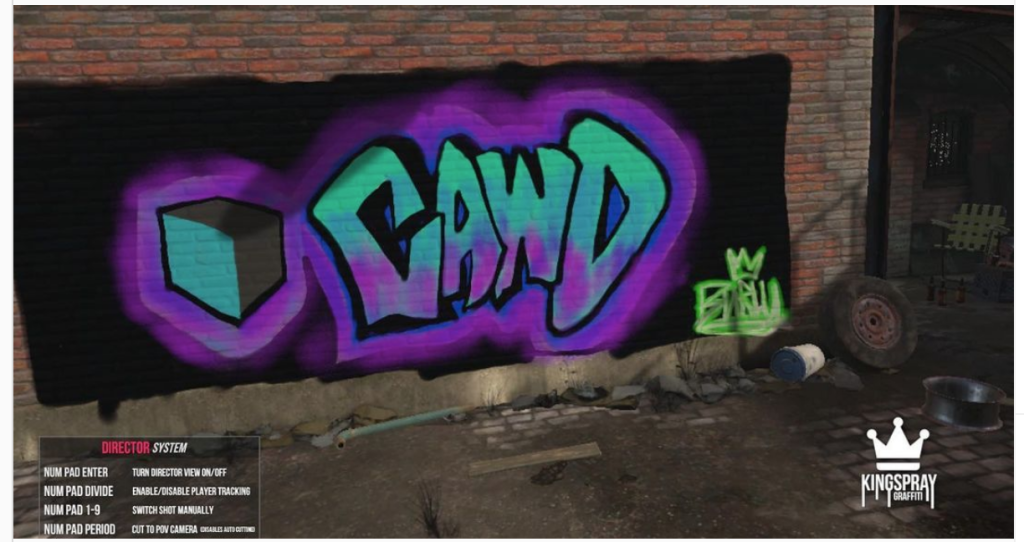
Enemy AI DEMO
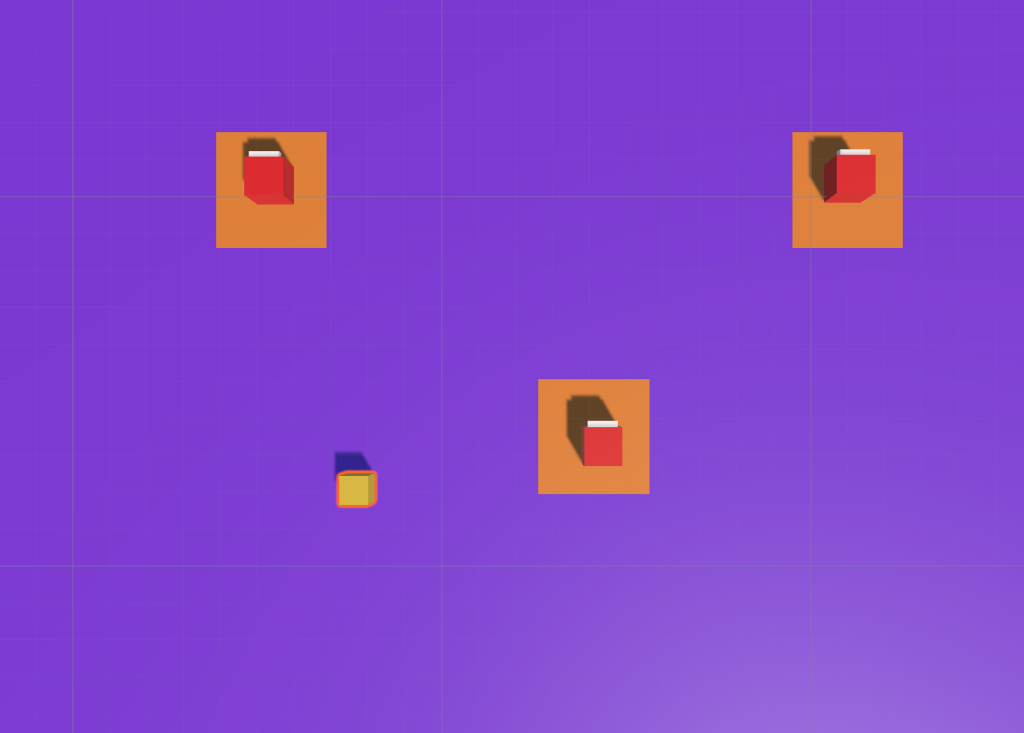
Now, for those of you with some enemy AI, I’ve created a little demo with some well commented code. I had some fun with this over the weekend.
I’ll take about 10 minutes to talk about what the code is doing, how it works and it might be something you can add to your game. If you are not interested, you can keep working on what you are working on.
There are two blocks of code – one is for basic player movement (no physics) and the other is for the enemy AI to chase the player. I did this one in 3D.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
float moveX = Input.GetAxis("Horizontal") * speed * Time.deltaTime;
float moveY = Input.GetAxis("Vertical") * speed * Time.deltaTime;
transform.Translate(moveX, 0, moveY);
}
}
Now for the enemy code – which you can place on your enemy.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyAI : MonoBehaviour
{
Transform player; //variable to access the player
[SerializeField] //makes the variable private but accessible in the editor
float enemySpeed, dis; //you can call more than one variable at a time on one line
Vector3 startPos; //this registers the players position at the game start
void Start()
{
player = GameObject.FindGameObjectWithTag("Player").transform; //this registers the players position at the game start
startPos = transform.position; // this registers the enemy's position at game start
}
void Update()
{
//the dis variable stores the distance constantly of the player and the enemy
dis = Vector3.Distance(transform.position, player.position);
if (dis <= 4) //if distance is less than 4 world units - chase the player
{
Chase();
}
if (dis >= 4) //if distance is greater than 4 units - the enemy goes back home
{
GoHome();
}
}
void Chase()
{
transform.LookAt(player); //this will make the AI look at the player - very cool
transform.Translate(0, 0, enemySpeed * Time.deltaTime); // enemy moving on Z axis
}
void GoHome()
{
transform.LookAt(startPos); //this will make the AI look for where they started
transform.position = Vector3.Lerp(transform.position, startPos, .002f);
//Lerp means from position a to position b over an amount of time
}
}
Here is some Code for a 2D version of an enemy chase. You get to determine the distance before the enemy chases you.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyMovement : MonoBehaviour
{
Transform player; //variable to access the player - which we'll get at the start of the game
[SerializeField] //makes the variable private but accessible in the editor
float enemySpeed, distance; //you can call more than one variable at a time on one line
public float distanceBetween; // decide how much distance you want to have until the player goes after you
void Start()
{
player = GameObject.FindGameObjectWithTag("Player").transform; //this finds and registers the players position at the game start
}
void Update()
{
distance = Vector2.Distance(transform.position, player.transform.position); //this function will get the distance between the player and the enemy and store in this float
Vector2 direction = player.transform.position - transform.position; //player position minus the enemy position will get the direction of the vector
direction.Normalize(); //this will make the vector have a magnitude of 1 so When normalized, a vector keeps the same direction but its length is 1.0
float angle = Mathf.Atan2(direction.y, direction.x) * Mathf.Rad2Deg; //this function is used to find the angle between two points
if ( distance < distanceBetween)
{
transform.position = Vector2.MoveTowards(this.transform.position, player.transform.position, enemySpeed * Time.deltaTime); //Move the enemy towards the player
transform.rotation = Quaternion.Euler(Vector3.forward * angle); //this is a rotation on the z axis (0,0,1) time the angle we found between two points.
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public int moveSpeed = 8;
void Update()
{
Movement();
}
void Movement()
{
float hmovement = Input.GetAxis("Horizontal");
float vmovement = Input.GetAxis("Vertical");
transform.Translate(Vector3.right * Time.deltaTime * moveSpeed * hmovement);
transform.Translate(Vector3.down * Time.deltaTime * moveSpeed * vmovement);
}
}
11:55 Lunch
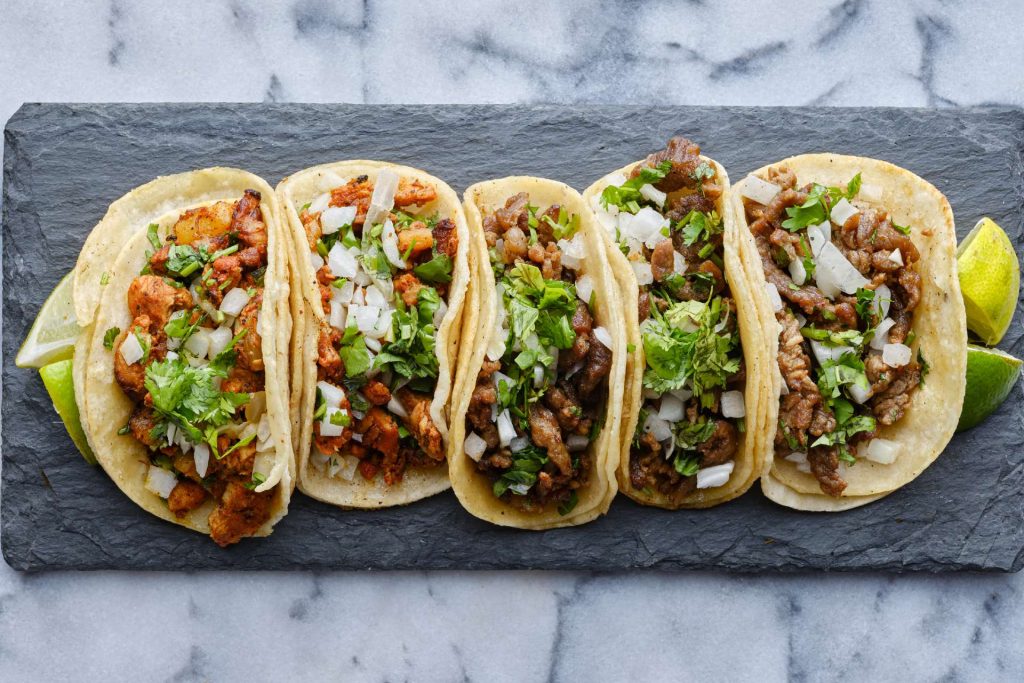
12:25 Independent Reading
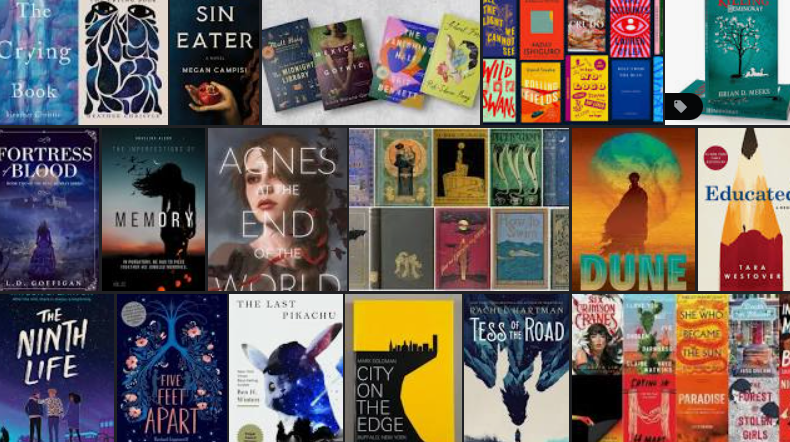
12:50 Break
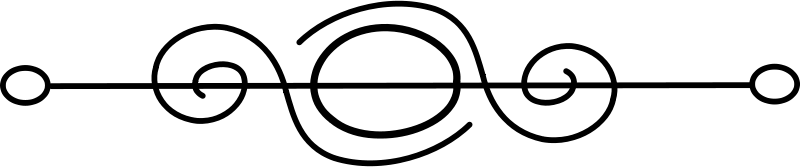
1:00 Production Time and Guided Support
Let’s make those games!
1:50 Dailies
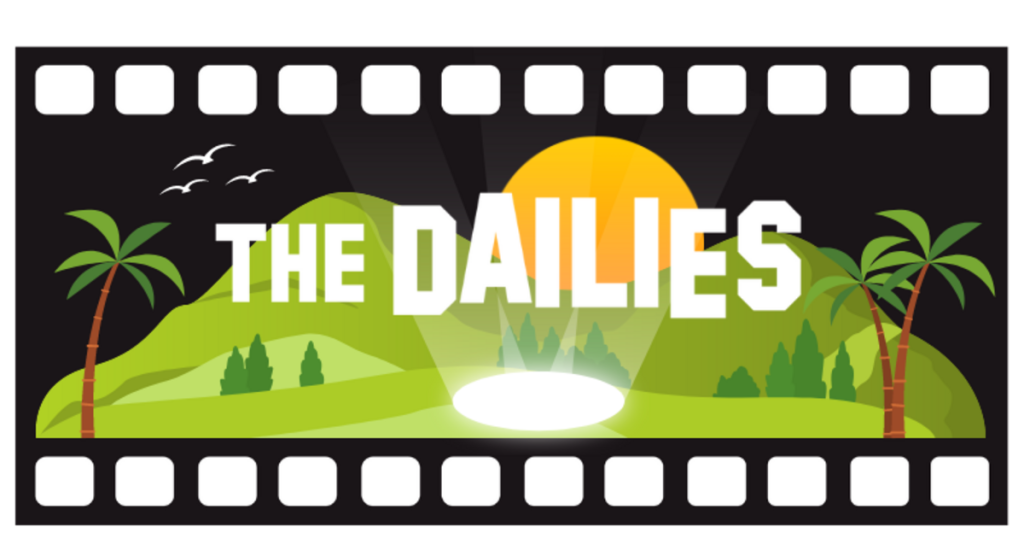
Dailies can be placed in the CAWD2 Dailies Folder on the CAWD2 Public Folders drive