Class hours: 10:05 – 2:45
Mr. Bohmann | wbohmann@ewsd.org
10:05 Today’s Notes & Attendance
Today is a Wacky Wednesday
Call Backs: Brodey
Mother’s Day is Sunday – don’t forget to do something nice – card or flowers is always a good call for that special person(s) that care for you.
10:10 Game Publishing with WebGL and Unity
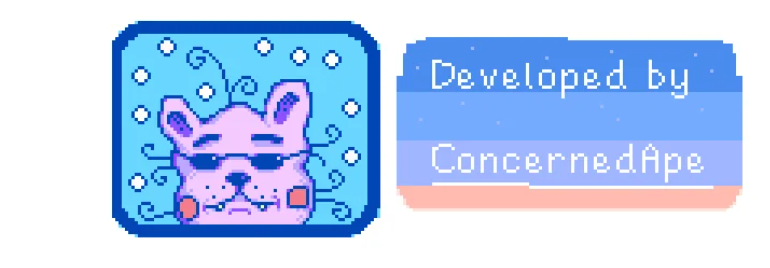
A splash screen is shown when a game first starts. Splash screens will usually display some combination of graphics and text to introduce the game or show author and publisher information. A splash screen can also be used to hide the time that it takes to initially load the game onto memory.
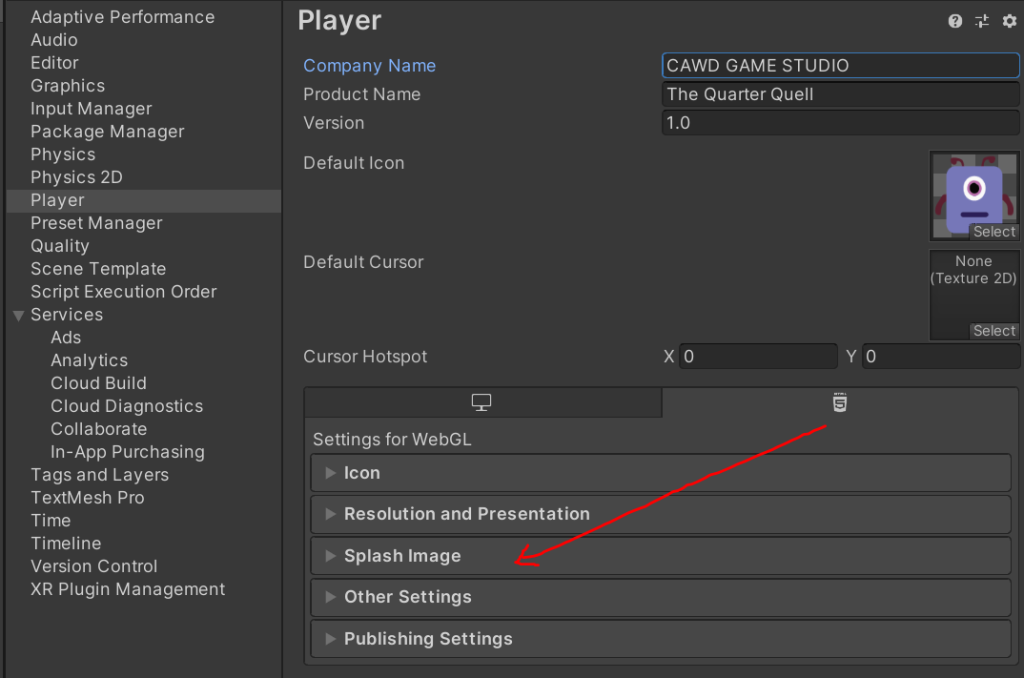
Unity has a default splash screen that is shown each time a Unity game is launched. The screen shows a “Made with Unity” graphic for a brief time. You will not see this screen until you have built your game for publication. Simmer.io is a publishing platform for WebGL builds (which Unity does well). if you published a game to that site you might have seen this splash screen.
You don’t normally see the splash screen until you build a game for publication. However, you can click the “Preview” button under the Splash Screen panel to see how your splash screen will look within the Unity IDE.
Game Icons
Every Unity game program has a game icon. This image identifies your program on the player’s computer or device. By default, Unity creates a small version of their own logo as your game’s icon. You will want to customize this icon to better identify your game. If publishing on the WebGL format, you will likely not have the ability to customize in the Icon area. It will use your chosen default icon.
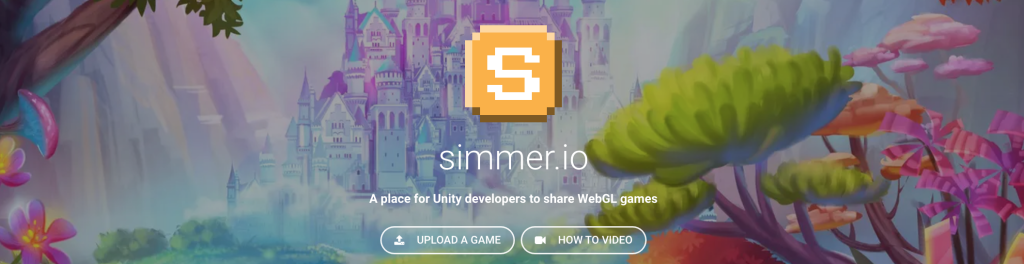
Let’s go through the steps to get your game BreakOut game published to the web. We’ll be publishing to Simmer.io. You will want to make an account there.
Follow along:
- Go to Project Settings and Change Publishing Settings to Disabled Compression Format (found under player / Other Settings / Publishing Settings)
- Go to Edit, Build Settings
- Change to the WebGL Platform (you may then have to select Switch Platform)
- Add Open Scenes to capture the scene you want to turn into a game
- Choose Build
- Create A Folder called WebGLBuild, select it and choose Build.
- It will take 5 minutes or so.
- Go to Simmer.io and create an account. Upload your WebGLBuild folder.
- Boom – Done!
- Fill in the meta details, add a screen shot, etc…
- Upload your finished and published game to this dropbox
Dropbox Results – click to play each other’s games this morning
10:50 Break
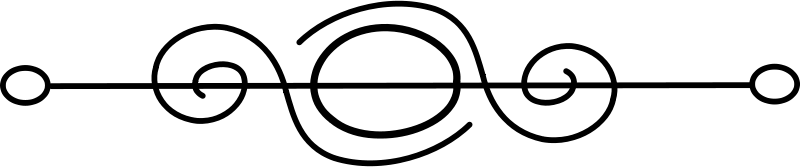
11:00 Accessing Scripts & Variables on other Game Objects
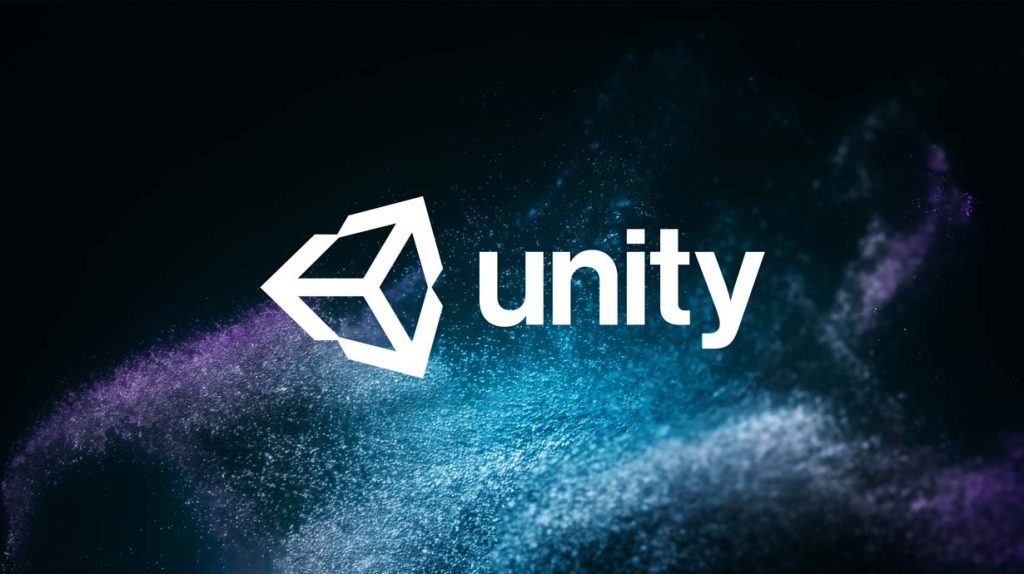
Your game logic may require scripts to interact with each other. How can one script on one GameObject get a reference to another script on a different GameObject during the game? Scripts are attached by a component so we just need to figure out how to access those game objects. Let’s try this out.
Let’s start by making a new scene – call it WorkingTwoSripts
- Create a Triangle Sprite
- Create a Square Sprite
- Create Two Scripts (name them:)
- Triangle Script
- Square Script
- Attach each script to the corresponding game objects
Now let’s write some code
First, let’s Add an audio clip to our project (there is one in Public Folders) and then add the Audio Source component to our Square
Then let’s open the Square Script
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SquareScript : MonoBehaviour
{
public AudioSource audioSrc; //to reference an audio clip
public int score = 0;
public bool puppyIsCute = false;
public void FunkySound()
{
audioSrc.Play();
Debug.Log("The SoundScript was called");
}
public void MakeTrue()
{
puppyIsCute = true;
}
public void SetScore()
{
score++;
}
}
Be sure to plug in the AudioSource component (or drag the Square game object to our new public variable slot in the inspector)
Then, let’s open the Triangle Script.
The first thing we need to do is create a reference to the script (and game object) that has the script we want to access. We’ll do this by making a variable that stores the script information.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TriangleScript : MonoBehaviour
{
public SquareScript squareScrpt; // this creates a reference to Square Script
// Update is called once per frame
void Update()
{
if (Input.GetKeyDown("space"))
{
squareScrpt.FunkySound();
Debug.Log("audio is playing");
squareScrpt.MakeTrue();
Debug.Log("My Pupply is cute: " + squareScrpt.puppyIsCute);
squareScrpt.SetScore();
Debug.Log("The Score is: " + squareScrpt.score);
}
}
}
Pretty cool. By using the dot operator (.) we can access variables like score, the boolean and we can access methods.
Now you try – create a method of your own. Make sure it is set to Public. Try making a variable too.
Bonus: Headers and Tool Tips
[Header("Variables")]
[Tooltip("Explains what your variable is about")]
11:55 Lunch
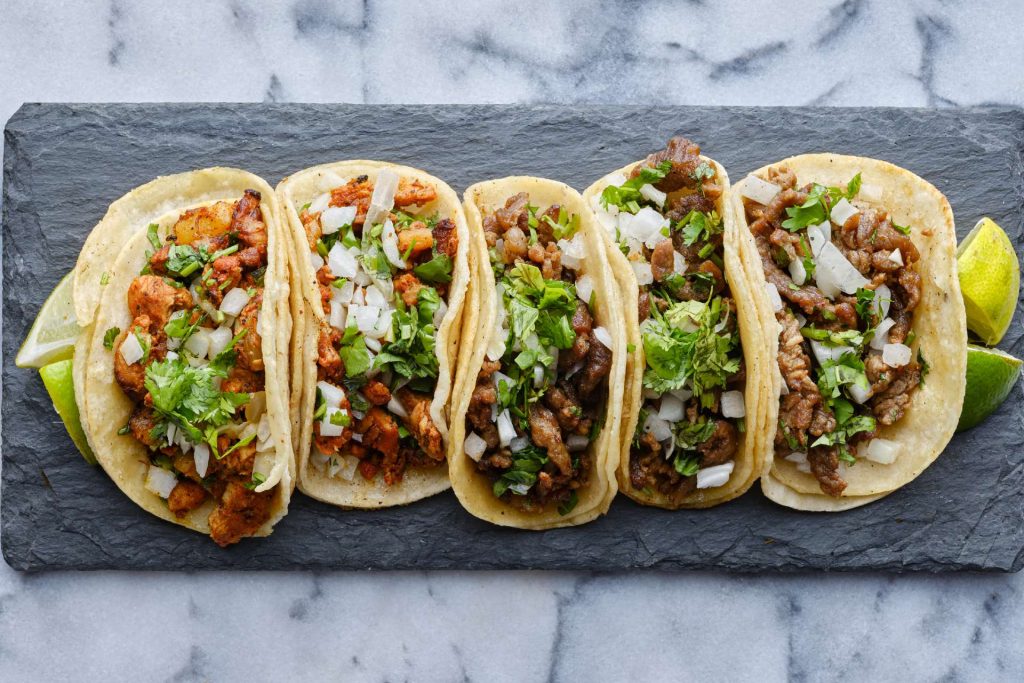
12:25 Independent Reading
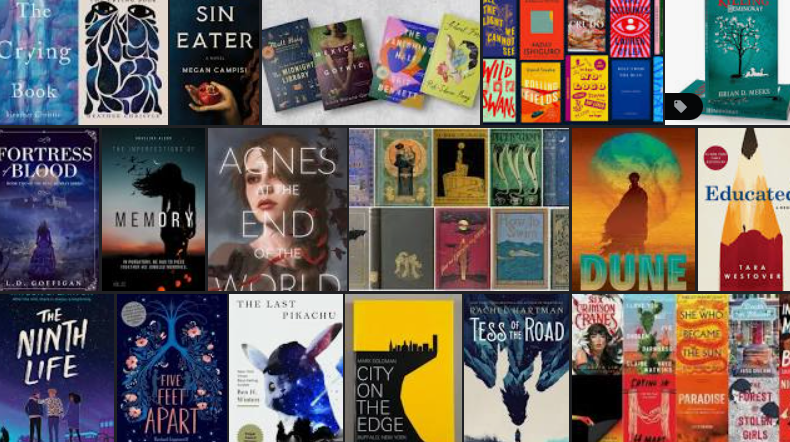
12:50 Break
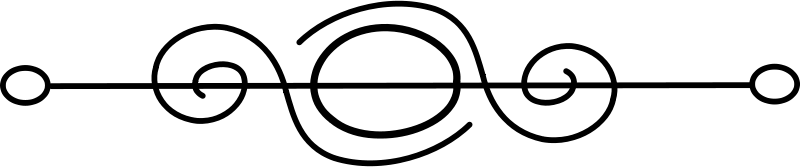
1:00 Production Time and Guided Support
1:50 Dailies
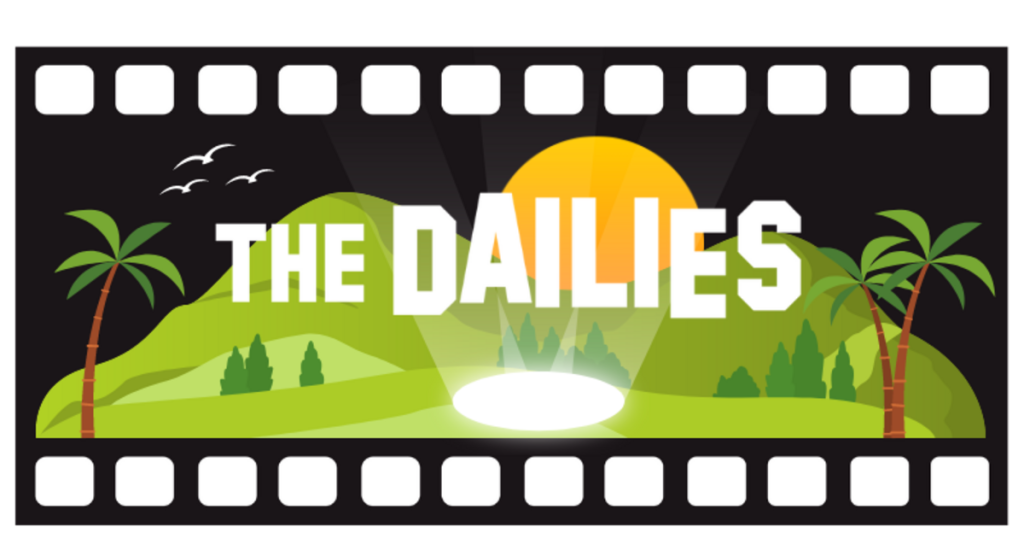
Dailies can be placed in the CAWD2 Dailies Folder on the CAWD2 Public Folders drive