Class hours: 10:05 – 2:45
Mr. Bohmann | wbohmann@ewsd.org
10:05 Today’s Notes & Attendance
Reminder – starting Monday – Food Drive – February 3rd – February 21st
We have an opportunity to participate in a state-wide Food Drive also a competition between Vermont’s Technical Centers for who can collect the most. Let’s make a difference: please bring in non-perishable foods and/or hygiene products.
Extra credit granted to one assignment in the quarter for each item you bring in!
Bird Animation Assignment -Posted on Google Classroom.
Add your bird (or use mine in public folders) to the playground set you modeled this week
Animate the swing
Add drag to the face by adding the simple deform modifier
Build the rest of your scene with lights and render in EEVEE
Animation duration: 3 seconds
Filename: BirdSwing.mp4 – project due next Thursday, February 6th
Team | SkillsUSA Comp |
---|---|
Peyton | Ben | Game Design |
Phoenix | Isaac | Animation |
Scotty | Hudson | Game Design |
Mia | Elle | Web Design |
Gabi | Tobi | Game Design |
Zander | Mallory | Animation |
Braden | Swing Man This role is to support / fill in / alternate |
SKills projects due at 12:55pm today for grading
10:10 SkillsUSA
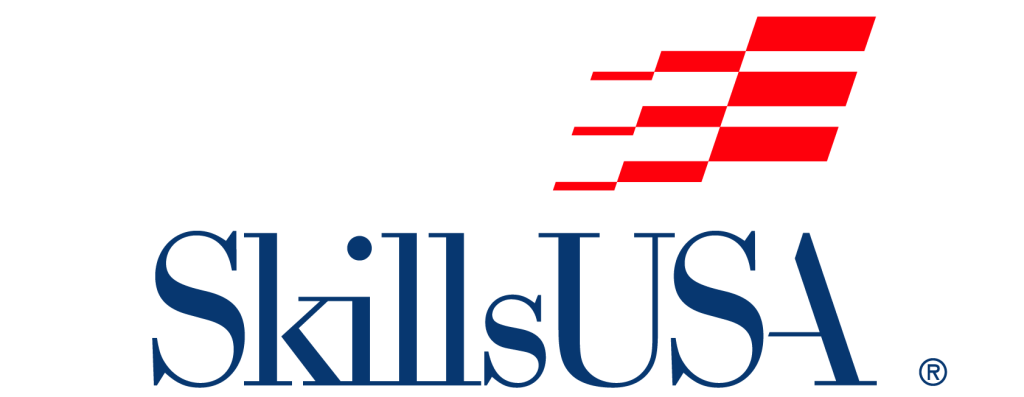
Each Thursday morning SkillsUSA Design Competition prep work will come out.
By Friday at end of lunch 12:55 Drop Dead Deadline! – have a folder with your two names on the Public inside of the weeks “skillsUSA” folder. The folder is found at Public/CAWD. For example if Mr. Cronin and Mr. Bohmann were working together the folder would be:
- “croninBohmann”
Create your folder in the discipline you are working in. DO THIS FIRST
Skills USA is a production grade that counts towards your Q3 grade. How much? – 20%. Your work will be graded on completion of deadlines, adherence to deliverables and your ability to work independently and with your partner. Time is limited, so use your production windows well. We’ll do Skills every Thursday & Friday up to the competition date in April. You’ll be graded on your work each week. Skills counts as 20% of your Quarter grade.
Skills Work for that week will always be due at 12:55 on Friday’s.
Watch the Time!
Once we pair up we are going to have a different task based on which event you and your partner wish to compete in.
This first week is really to get a working relationship with your Partner – most if not all of the team work will be done on a single computer this week. Sit together.

See Mr. Cronin’s Dayplan for specifics – read carefully

See Mr. Cronin’s Dayplan for specifics – read carefully

Create a single web page with your Team Profiles and come up with a Team Name. This project should push yourselves to create something visually attractive, and full of information about your team. Images of you and your partner or logos of you and your partner or something that represents you.
- Identify Who will be the Web Developer (In charge of the html and JS) – Web Dev does this part.
- Identify Who will be the Web Designer (In charge of more of the artistic choices and styles – CSS) – Web Designer does this part.
- Impress us with what you can create (even if you just got started with web)
- Add a custom font or two
- Include a brief bio of each team member so we can learn about you
- Don’t forget your meta data in the head tag
Upload your completed project to your team folder. It is only one page.
- Your page will be called index.html
- Images will be in an images folder
- CSS will be in a css folder
- Fonts will be in a Font Folder
- Include an image for your planning document – we’ll call this a rough wireframe (this means you will sketch your design on paper with a rough layout BEFORE you begin coding.
- For some of you, this might be new and you don’t know what some of these terms are – please ask me – Mr. B and I will help you.
As always, validate your code – HTML / CSS. Practice commenting and organizing your code.
10:50 Break
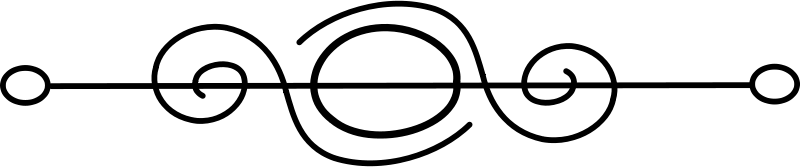
11:00 Unity
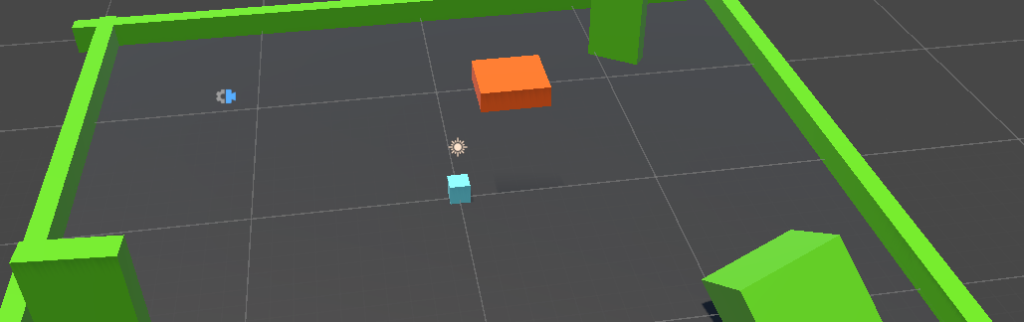
Last week Friday in Unity we tackled Cinemachine, collisions and some more basic scripting including refactoring and practicing methods by calling some built in Collision methods. Let’s review where we left off.
Collisions
The following function was in the ObjectHit script we made, that states when something hits this game object, turn this game object red. This script was placed on all the walls and obstacles.
void OnCollisionEnter(Collision other)
{
GetComponent<MeshRenderer>().material.color = Color.red;
//if you want it to change random colors try this one instead
//GetComponent().material.color = Random.ColorHSV(0f, 1f, 1f, 1f, 0.5f, 1f);
}
Code for the Player to initiate a Score and this script goes on the player
//global variables here
public int playerScore = 100;
public int hits;
void OnCollisionEnter(Collision other)
{
if (other.gameObject.name == "Wall")
{
playerScore = playerScore - 1;
Debug.Log("your score is " + playerScore);
hits = hits + 1;
}
if(hits > 4)
{
Destroy(gameObject);
Debug.Log("Game Over");
}
}
This Week in Unity – Getting Components from Game Objects and Conditionals
Since we are making an obstacle dodge type game – the process we are going through is setting up game functionality but not worrying so much about what it looks like, but how things work – a form a grayboxing in game design terms.
Goal/Problem: Let’s make a object fall from the sky after 3 seconds
Solution: What do you think we need to make this happen?
This code will go on any object that drops from the sky… (if you use this code, be sure to copy only the stuff below the first and last curly brace – as you might have named your script something else)
using UnityEngine;
public class ObjectDropper : MonoBehaviour
{
//Variables
[SerializeField] float timeToWait = 3f;
Rigidbody myRigidBody;
void Start()
{
GetComponent<MeshRenderer>().enabled = false;
myRigidBody = GetComponent<Rigidbody>();
myRigidBody.useGravity = false;
}
void Update()
{
if (Time.time > timeToWait)
{
GetComponent<MeshRenderer>().enabled = true;
myRigidBody.useGravity = true;
}
}
}
Some stationary obstacles might be nice to have – let’s add a rotating object
Goal/Problem: Have an object rotate when the game begins
Solution: What steps are needed to make this happen?
This code will go on any object you want to spin… (if you use this code, be sure to copy only the stuff below the first and last curly brace – as you might have named your script something else)
using UnityEngine;
public class ObjectRotate : MonoBehaviour
{
// variables
[SerializeField] float xAngle = 0f;
[SerializeField] float yAngle = 1f;
[SerializeField] float zAngle = 0f;
void Start()
{
}
// Update is called once per frame
void Update()
{
transform.Rotate(xAngle, yAngle, zAngle);
}
}
11:50 – 12:25 – SkillsUSA Production Continued…
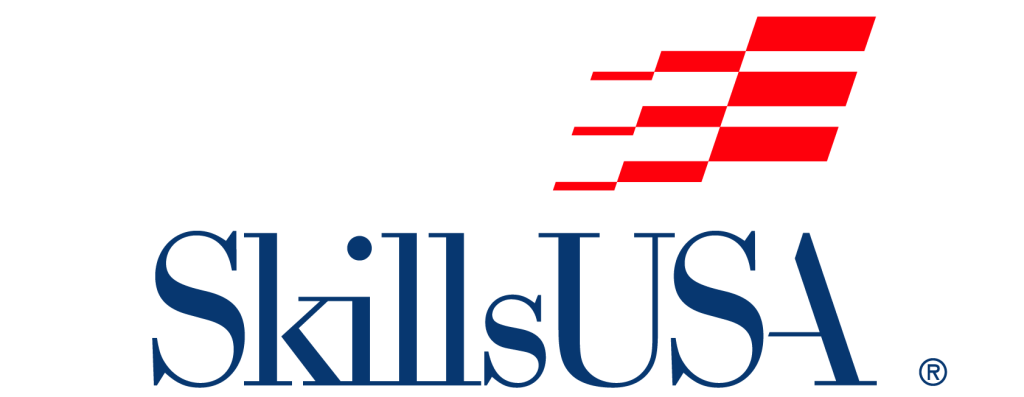
12:25 – 12:55 Lunch
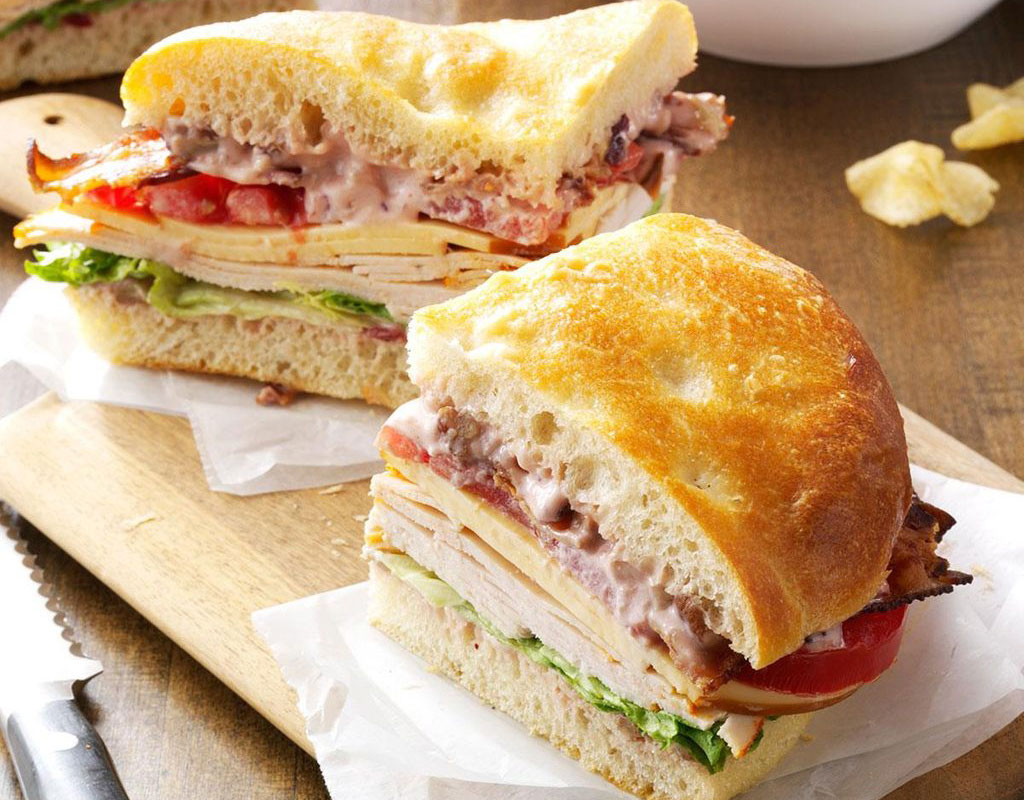
12:55 Independent Reading
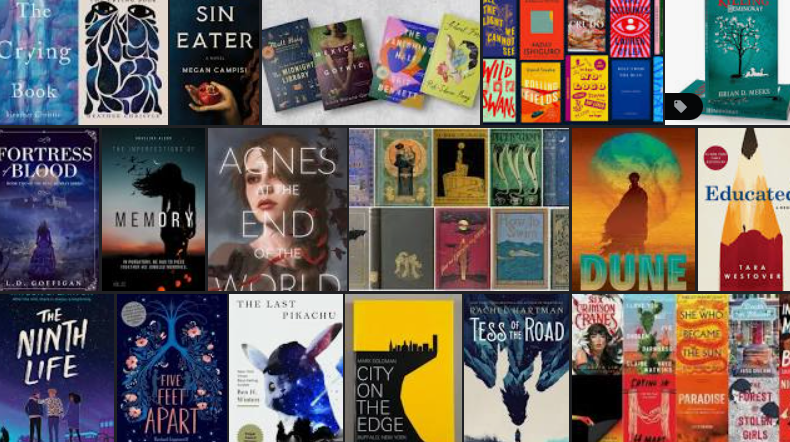
1:20 Break
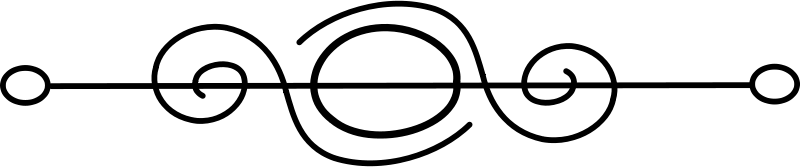
1:30 20% – Passion Project Work Time
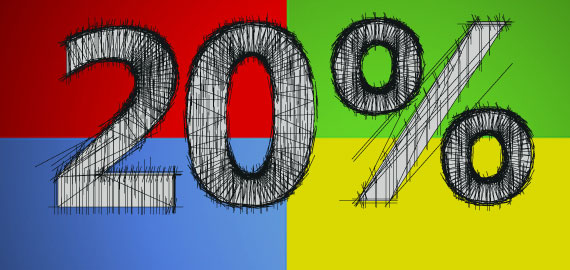
Production Time and Guided Support
Current Assignments:
- Principle #1 Squash & Stretch Animation – due Monday
- Bird Animation – Bird on Swing Set – Thursday, February 6th
2:38 Dailies
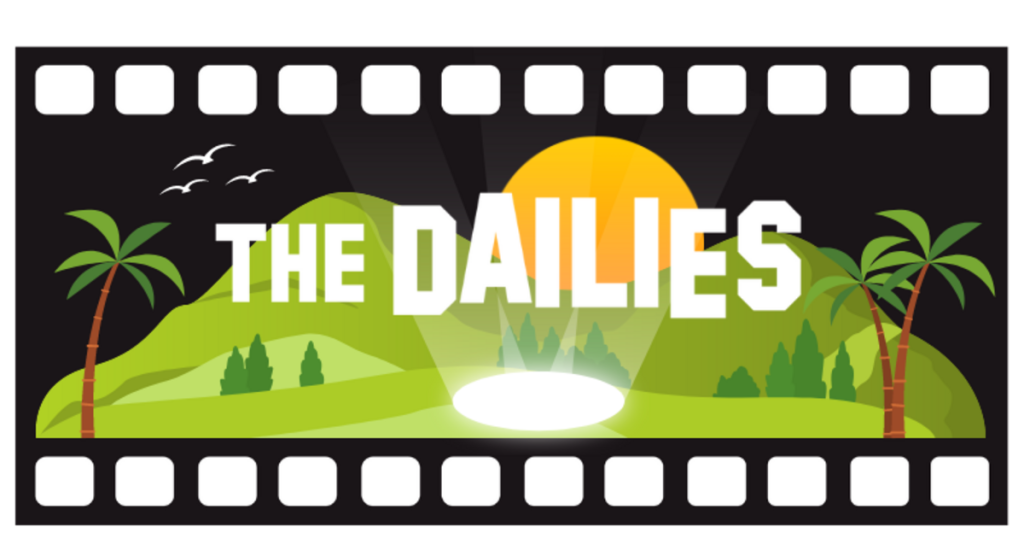
Dailies can be placed in the CAWD2 Dailies Folder on the CAWD2 Public Folders drive