Class Hours: 10:05 – 2:40
Mr. Bohmann | wbohmann@ewsd.org
10:05 Today’s Notes & Attendance
- SkillsUSA Gold Medalist Meeting 6pm Cafeteria tonight
SceneManager & Multiple Scenes
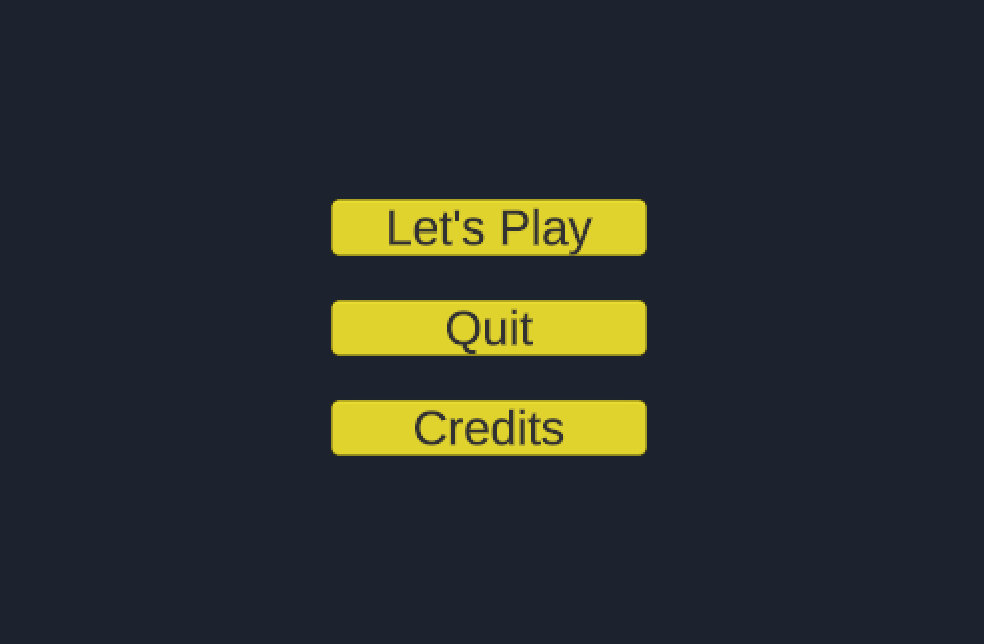
Scene Build in Settings – Let’s look at
Games will commonly start on a home or title scene that is shown before gameplay begins. Home screens often include some graphics, display the name of the game and offer the player a chance to configure some game settings. When the player is ready to start, they will click on a button to begin the game.
A settings screen may ask the user to enter a player name and adjust other settings like volume and difficulty level. Once the player has selected all of their options, they can usually click on a button to return to the home screen or begin playing the game.
The Unity Canvas allows you to add buttons to your scenes very easily. To add a new button, just select “GameObject” from the top menu, then choose “UI” and then “Button“. This will place a basic gray button on your scene with the text “Button”
Let’s create a Home Screen (Scene) for our Arkanoid Game. This will also allow us to review how to set up buttons. We’ll need to create a SceneLoader Script because buttons are only useful if they perform some action when clicked by a player. We’ll attach this SceneLoader Script to an empty game object that we can call Button Controller.
When a button is clicked, Unity can call one or more functions in response. To link your script functions to a button, click on the Button object in the Hierarchy. You will see an “OnClick” section in the Inspector panel. Initially, the list is empty, so your button won’t do anything.
Remember that you can attach a script to any GameObject, so your script function that handles a button click could be attached to the Button object itself, any other sprite on the screen, an empty controller object(like we are doing), and so on.
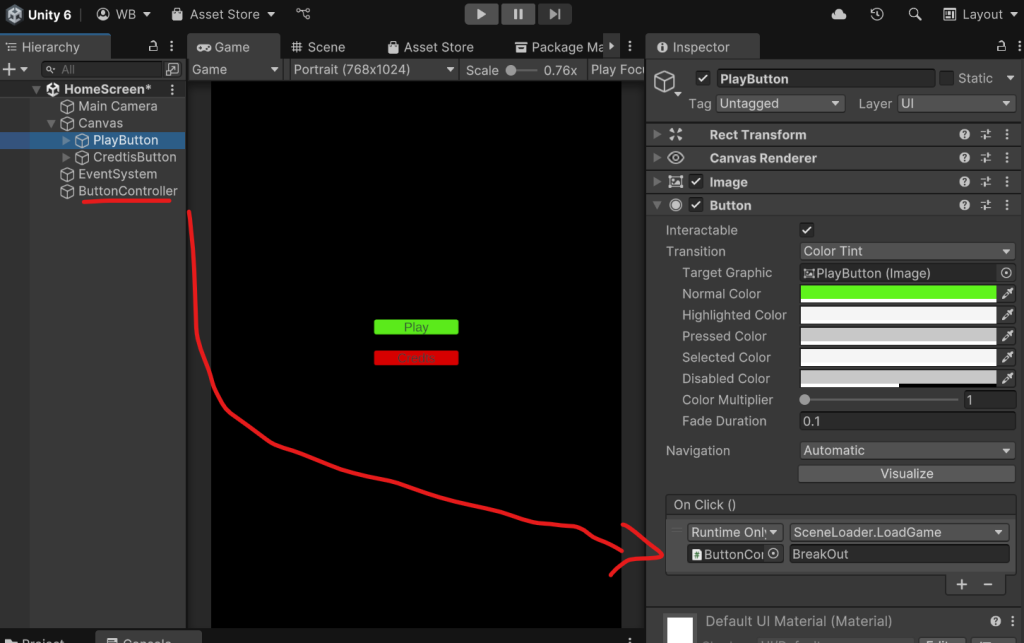
Once you select a game object, the “Function” drop-down box will display a list of all the components on the object, including all scripts. In this example, we have attached a script called “SceneLoader” to the PlayButton object, and you can see that script in the list.
On our Arkanoid game, lets add a UI.
using UnityEngine;
using UnityEngine.SceneManagement;
public class SceneLoader : MonoBehaviour
{
public void LoadGame(string sceneName)
{
SceneManager.LoadScene(sceneName);
Debug.Log("Button Clicked");
}
public void QuitGame()
{
Application.Quit();
}
public void LoadCredits(string sceneName)
{
SceneManager.LoadScene(sceneName);
Debug.Log("Credits button presseed");
}
}
Exiting or Quitting a Game
There is one last important consideration when publishing a standalone game. How do players exit
the program? When running in the Unity IDE, you can easily start and stop the entire game by clicking
on the “Play” arrow button above the Game tab.
However, regular players won’t have that option and are limited to the UI options you have included within the game.
None of our little mini games we’ve made have included any sort of “exit” feature that players can use to stop the game. You will commonly want to include a button on some scene that will allow users to exit the game. When that button is clicked, call the Application.Quit() function to cause the game to exit.
// this function can be called by a button click event
public void QuitGame()
{
// exit the game entirely if running as a standalone program
Application.Quit();
}
10:50 Morning Break (10 minutes)
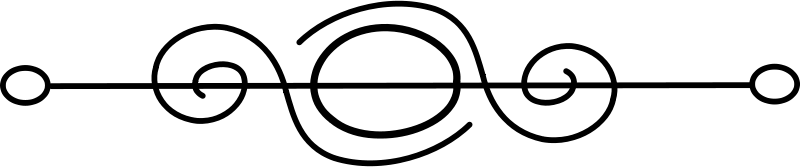
11:00 New Game Build – Shipyard Captain
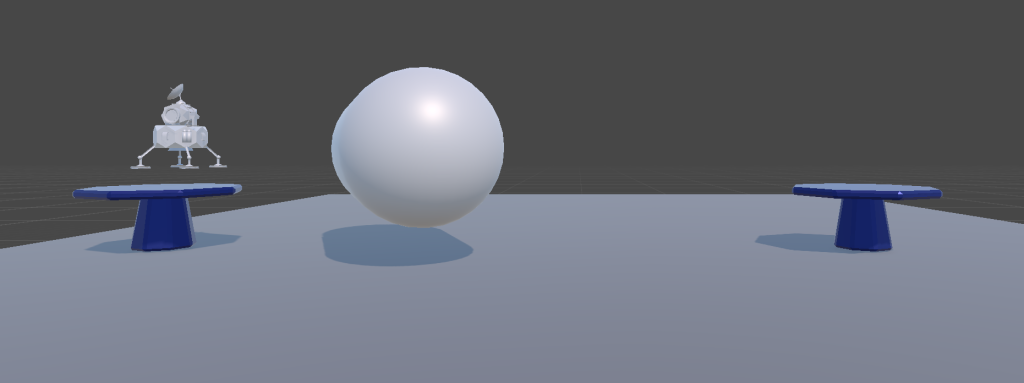
Game Type: 3D Game Flight Simulation
Game Mechanics: While set in 3D space, the user will fly a lander from one platform to another primarily along 1 axis. Left and Right Arrows (A&D alternates) space bar for thrust.
Win Condition: Avoid obstacles and successfully find the lander platform. Levels will become incresingly difficult. Nice to have fuel pickups and or power ups to scale game.
Lose Condition: Crashing
Environment: Space style feel with camera following the lander. Strong foreground and background to make parallax style effect.
We are going to need some assets for this. We can raid the Asset Store and create our own. The image above is a working prototype.
Today we’ll:
- Set up the Game Environment
- Try out the New Input System
- Build our thrust and rotation controls
Specifically for the Input system we will need:
UnityEngine.InputSystem in the namespace
Create InputAction variables (that are serialized)
Add bindings for each InputAction
Enable each input action
Use the Value of the InputAction for gameplay
using UnityEngine;
using UnityEngine.InputSystem;
public class Movement : MonoBehaviour
{
[SerializeField] InputAction thrust;
[SerializeField] InputAction rotation;
[SerializeField] float thrustStrength;
[SerializeField] float rotationStrength;
Rigidbody rb;
private void OnEnable()
{
thrust.Enable();
rotation.Enable();
}
void Start()
{
rb = GetComponent<Rigidbody>();
}
private void FixedUpdate()
{
VerticalThrust();
ProcessRotation();
}
private void VerticalThrust()
{
if (thrust.IsPressed())
{
rb.AddRelativeForce(Vector3.up * thrustStrength * Time.fixedDeltaTime);
}
}
private void ProcessRotation()
{
float rotationInput = rotation.ReadValue<float>(); //this will check to see //if the negative or positive key is pressed and store that value in the variable
if (rotationInput < 0)
{
ApplyRotation(rotationStrength);
}
else if (rotationInput > 0)
{
ApplyRotation(-rotationStrength);
}
//else if means that momvement is mutaually exclusive otherwise you could //press both keys at the same time.
}
private void ApplyRotation(float rotationThisFrame)
{
transform.Rotate(Vector3.forward * rotationThisFrame * Time.fixedDeltaTime);
}
}
11:55 GMetrix Coursework
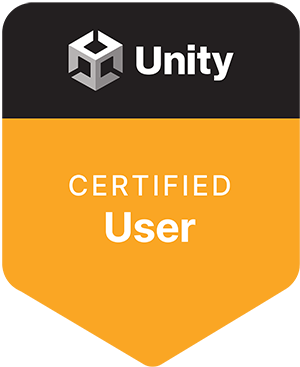
12:25 Lunch
12:55 Independent Reading
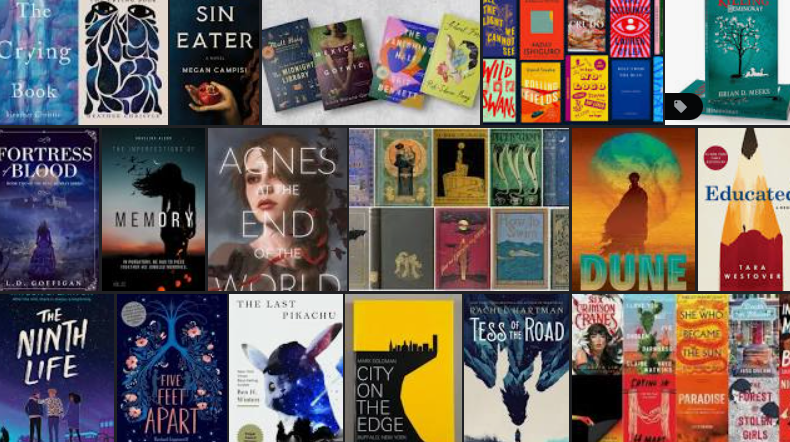
1:20 Afternoon Break (10 minutes)
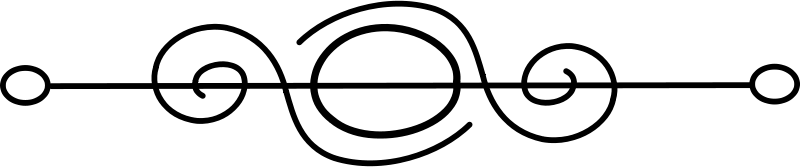
1:30 Speed Design
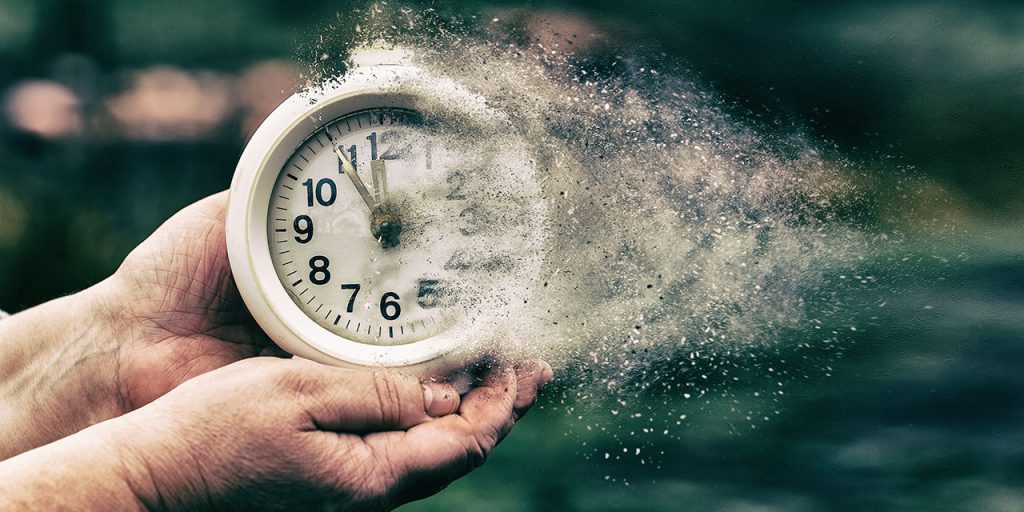
1:50 Independent Production & Guided Support
Breakout Game
2:38 Dailies
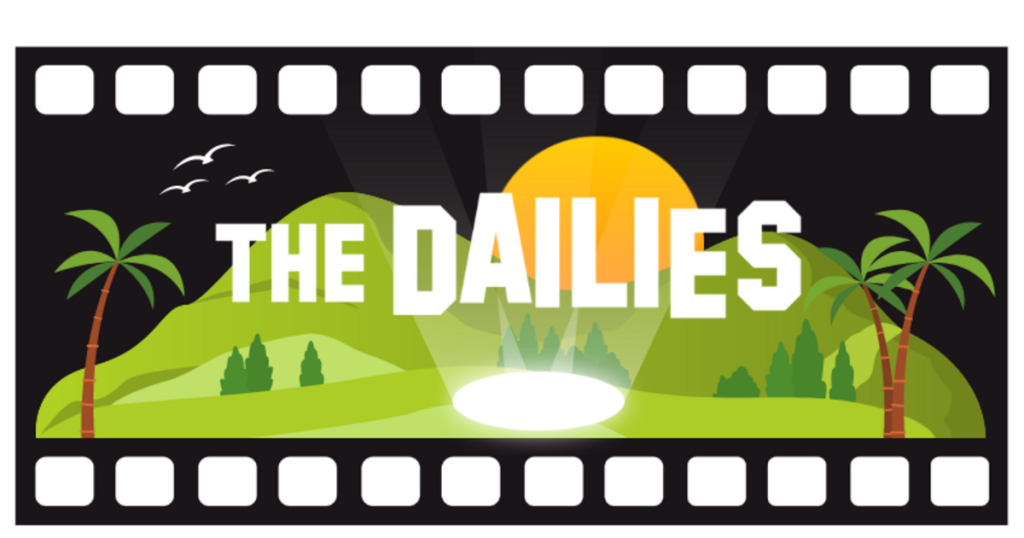