Class hours: 10:05 – 2:45
Mr. Bohmann | wbohmann@ewsd.org
10:05 Today’s Notes & Attendance
- Today is a Wacky Wednesday
- Call Backs:
- Two Visitors today from PreTech – this will take place at 11am right after break.
10:10 THE DOM TREE
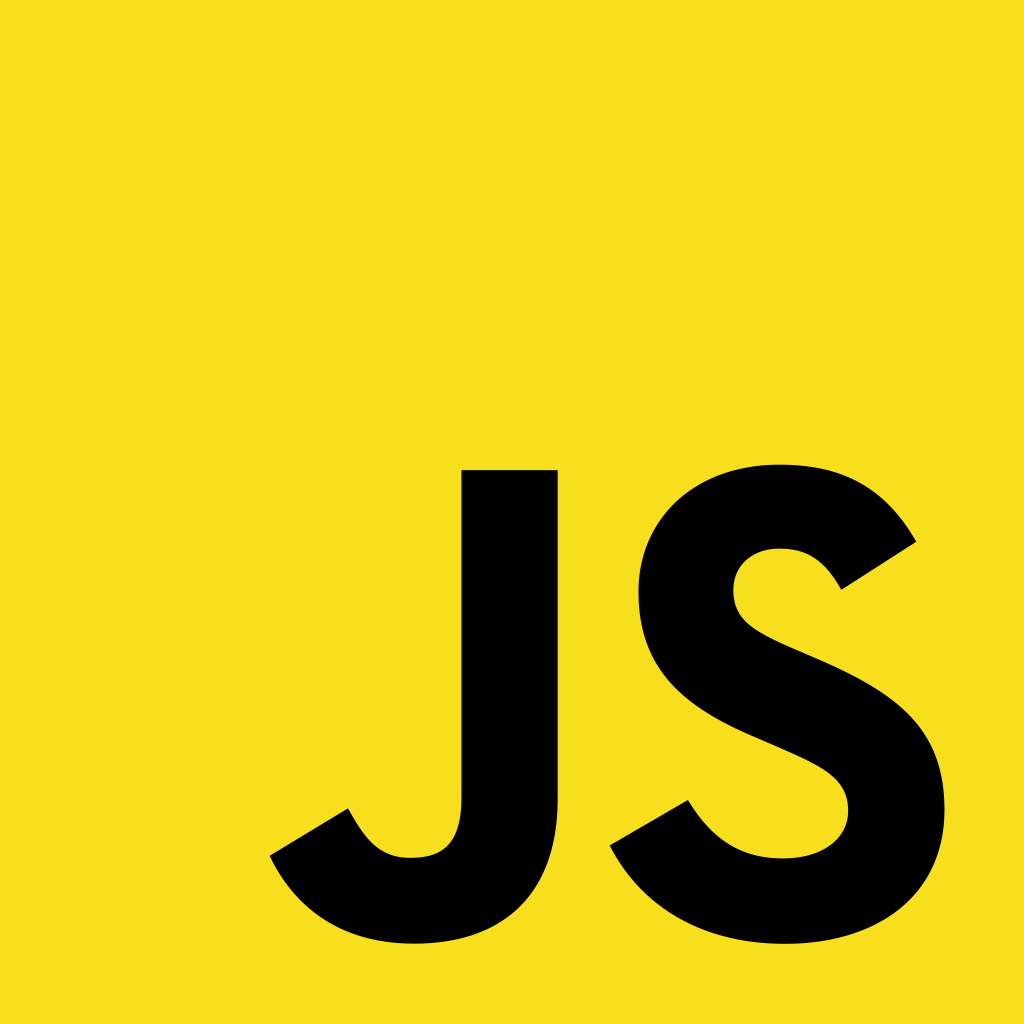
Why Study JavaScript?
JavaScript is one of the 3 languages all web developers must learn:
1. HTML to define the content of web pages
2. CSS to specify the layout / styling of web pages
3. JavaScript to program the behavior and interactivity of web pages
So if you want to have your webpage be interactive, you are going to need to learn some JavaScript. Fortunately there are thousands of resources, code snippets and tutorials to assist with all kinds of projects.
Our goal today is simple:
- Use the console to explore the DOM (Document Object Model)
- Learn about Elements and Nodes
- Get familiar with the developer tools and console
- Create a small project to teach us how to link our JS and do some sample code
The DOM stands for Document Object Model
When we set up an html document (index.html) we are creating the document object model. The DOM is just a visual structure of your document. We can see this in the inspector with our Dev Tools. Let’s do this quickly by going to example.com
Think of the DOM as an upside down tree. The root being the Document Type (HTML) and then the branches descending. So that means that the HTML element is the Root Element of our tree. Therefore the Head and Body elements are its children.
Working through the DOM with JavaScript allows programmers to be able to target elements and write programs to make (in this case) web sites interactive. The elements that provide structure to the web are objects.
All Elements in the DOM are nodes, but not all nodes are elements. Now, you already know a thing or two about this but maybe didn’t think about it much in terms of JS.
The three most popular types of nodes are:
- Element Node
- Attribute Node
- Text Node
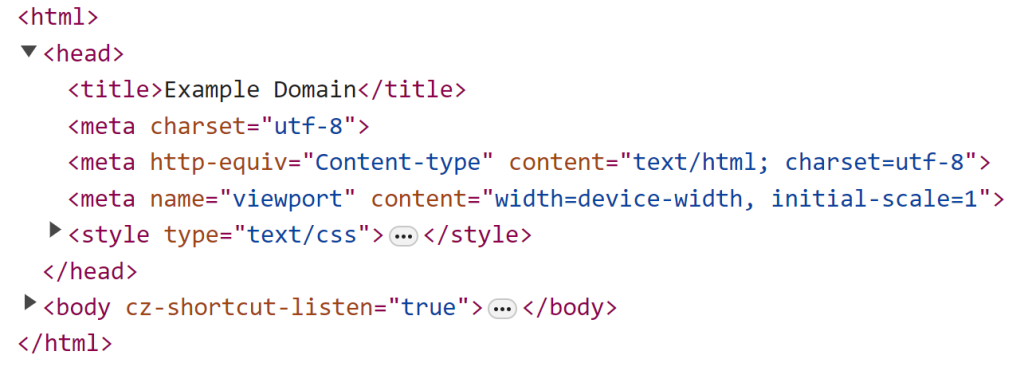
<title> is an Element Node
name, content are Attribute Nodes
for an img tag – img is the element, src and alt are the attribute nodes
Example Domain is the text between the elements and are the Text Nodes
nodeType – tells you what kind of node you have
nodeName – returns the name of the node
w3schools Node Types
Using a sample webpage, let’s explore the Node interface, the document object and some document object methods.
10:50 Break
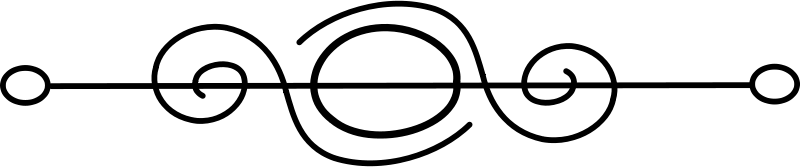
11:00 – 11:30 Visitors and Portfolio Production Time
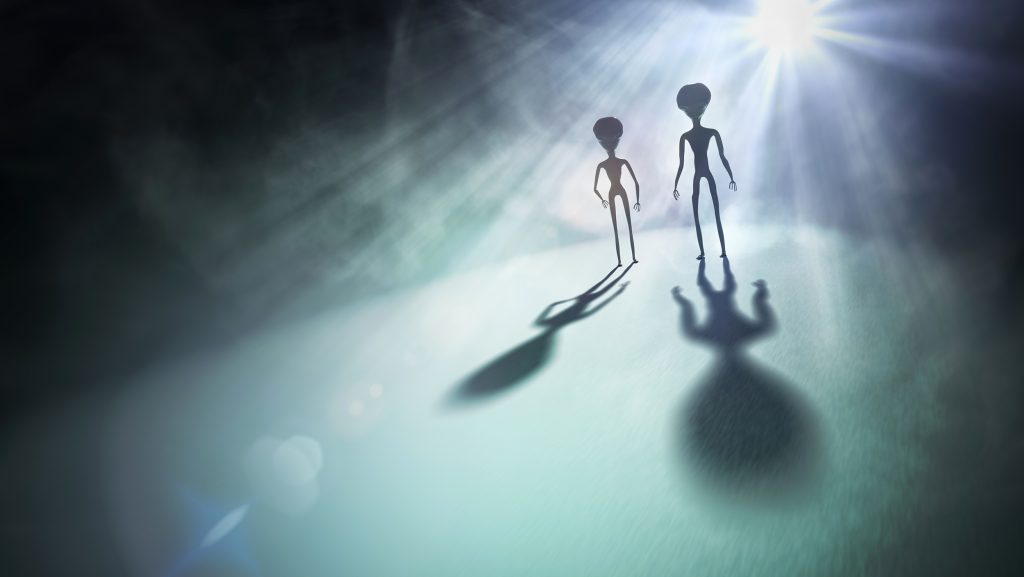
Portfolio Jam Session
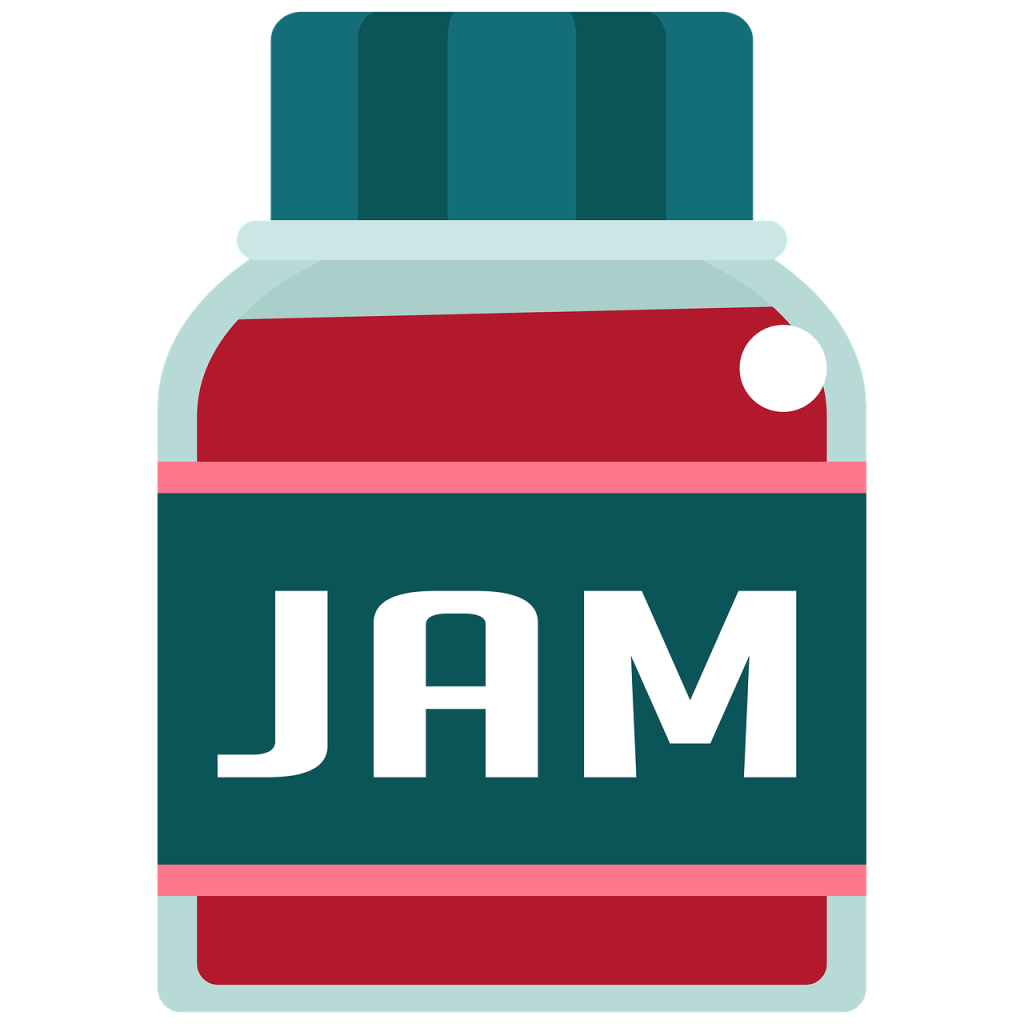
- Wireframe of rest of your site (due Friday)
- Build the structure based on your desktop wireframe design (you can CSS later or use Bootstrap)
- Connect Pages with Navigation
- By the end of the week the rough design of your site is complete, based on your wireframe designs
11:30 CSS Lightbox –
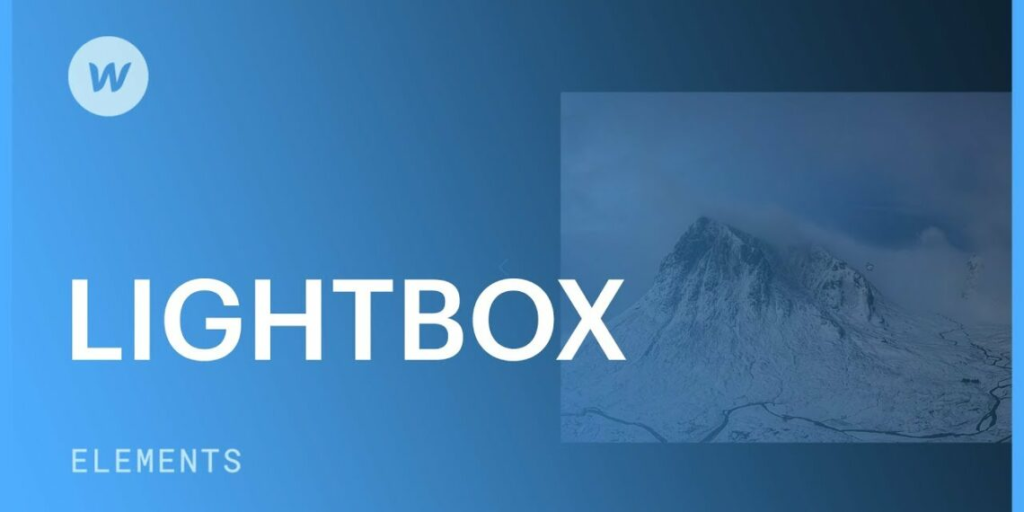
No JS, just good old html and CSS.
This one is easy and effective. I think you’ll like it.
Sample Code CSS
.container {
width: 50%;
display: flex;
margin: auto;
gap:5px;
}
img {
max-width: 100%;
height: auto;
}
/* lightbox markup */
.lightbox span {
display: block;
width: 50%;
height: 100vh; /* makes span height of viewport */
/* to center the content on the screen */
display: flex;
flex-direction: column;
align-items: center;
margin: auto;
}
.lightbox> div >p {
color: white;
font-size: 1.5em;
}
.lightbox {
/* default to hidden */
display: none;
background: rgba(0, 0, 0, .9);
width: 100%;
/* Overlay the screen */
position: fixed;
z-index: 999;
top: 0;
left: 0;
right: 0;
bottom: 0;
/* space around image */
padding: 2em;
text-decoration: none;
}
/* to unhide the lightbox when it is targeted */
.lightbox:target {
display: block;
}
Sample Code – HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Lightbox</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1>CSS Lightbox</h1>
<main class="container">
<!-- images container -->
<div class="img-container">
<a href="#img1">
<img src="images/bevinsScarecrow.jpg" alt="scarecrow">
</a>
<a href="#" class="lightbox" id="img1">
<div>
<img src="images/bevinsScarecrow.jpg" alt="scarecrow">
<p>This is a ScareCrow</p>
</div>
</a>
</div>
</main>
</body>
</html>
11:55 Lunch
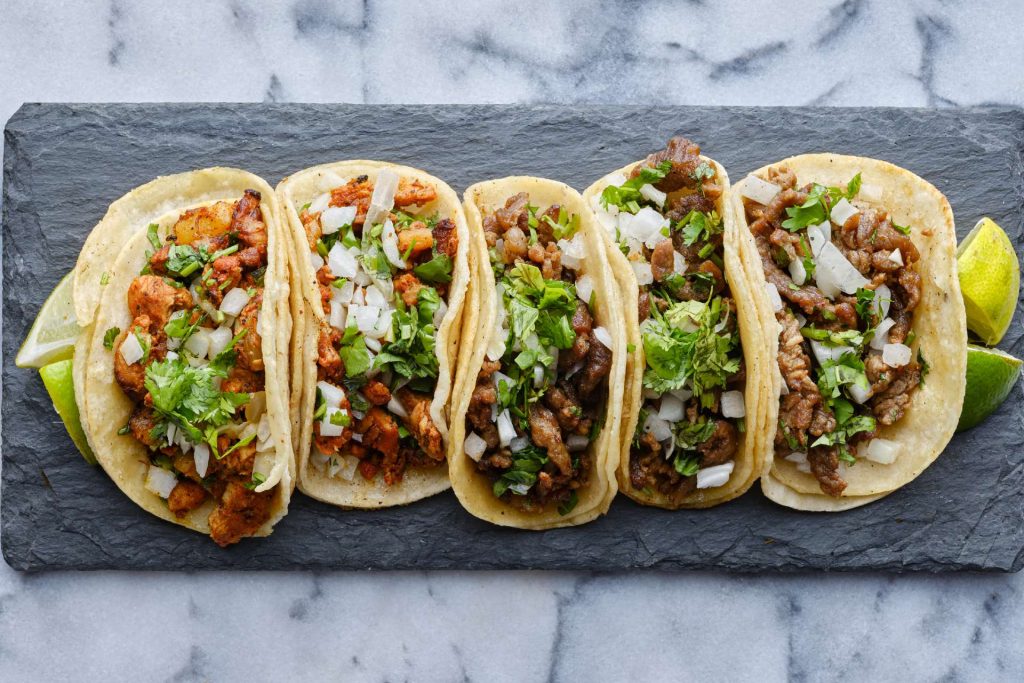
12:25 Independent Reading
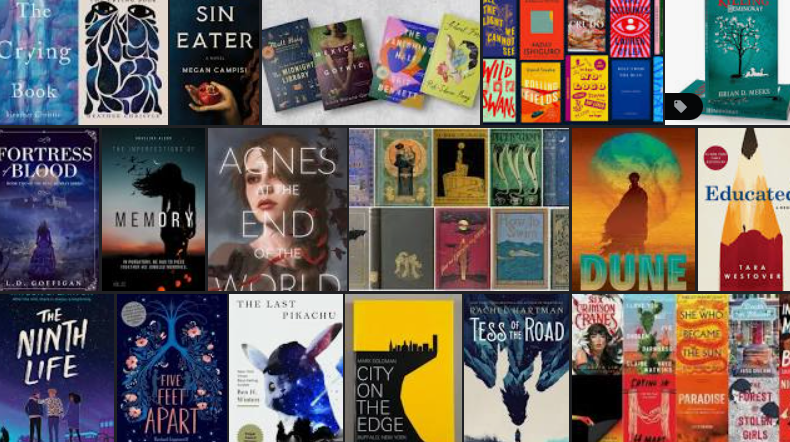
12:50 Break
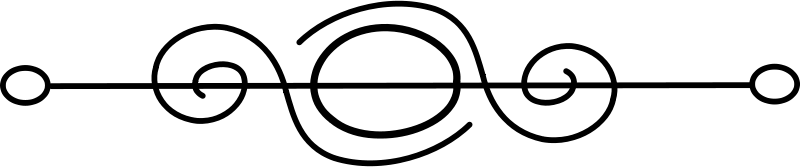
1:00 Production Time and Guided Support
1:50 Dailies
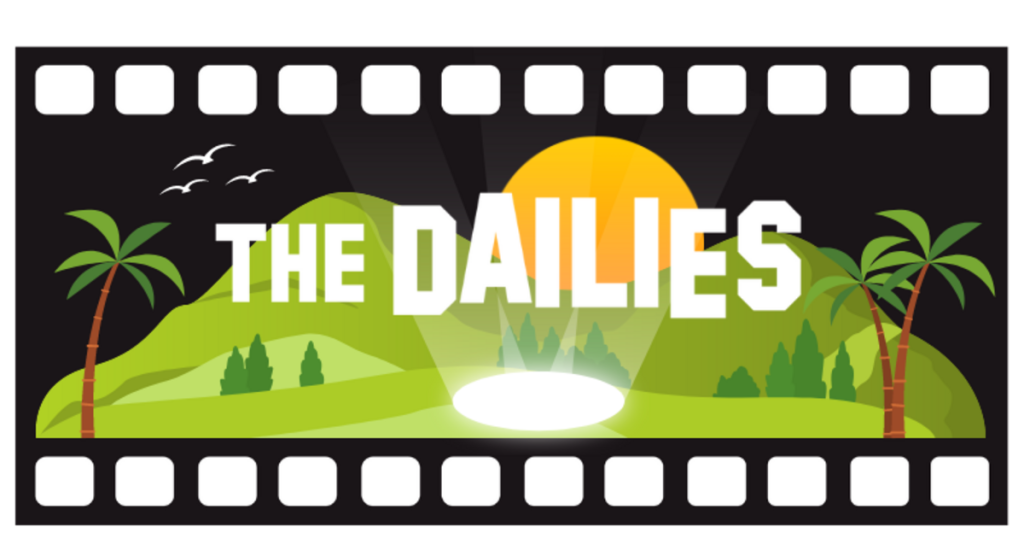
Dailies can be placed in the CAWD2 Dailies Folder on the CAWD2 Public Folders drive